Question
Javascript and HTML What is the code for this game? It provides most of it but am having a hard time completing what it says
Javascript and HTML
What is the code for this game? It provides most of it but am having a hard time completing what it says to do. What is all of the code for game when its done?
You need to make a new HTML page called Hangman.html
The first thing we have to do is to choose a random word. Heres how that will look:
1 var words = [
"javascript",
"monkey",
"amazing",
"pancake"
];
2 var word = words[Math.floor(Math.random() * words.length)];
We begin our game at 1 by creating an array of words (javascript, monkey, amazing, and pancake) to be used as the source of our secret word, and we save the array in the words variable. The words should be all lowercase. At 2 we use Math.random and Math.floor to pick a random word from the array
Next we create an empty array called answerArray and fill it with underscores (_) to match the number of letters in the word.
var answerArray = [];1 for (var i = 0; i < word.length; i++) { answerArray[i] = "_"; } var remainingLetters = word.length;
The for loop at 1 creates a looping variable i that starts at 0 and goes up to (but does not include)word.length. Each time around the loop, we add a new element to answerArray, at answerArray[i]. When the loop finishes, answerArray will be the same length as word. For example, if word is "monkey" (which has six letters), answerArray will be ["_", "_", "_", "_", "_", "_"] (six underscores).
Finally, we create the variable remainingLetters and set it to the length of the secret word. Well use this variable to keep track of how many letters are left to be guessed. Every time the player guesses a correct letter, this value will be decremented (reduced) by 1 for each instance of that letter in the word.
The skeleton of the game loop looks like this:
while (remainingLetters > 0) { // Game code goes here // Show the player their progress // Take input from the player // Update answerArray and remainingLetters for every correct guess}
We use a while loop, which will keep looping as long as remainingLetters > 0 remains true. The body of the loop will have to update remainingLetters for every correct guess the player makes. Once the player has guessed all the letters, remainingLetters will be 0 and the loop will end.
The first thing we need to do inside the game loop is to show the player their current progress:
alert(answerArray.join(" "));
We do that by joining the elements of answerArray into a string, using the space character as the separator, and then using alert to show that string to the player. For example, lets say the word is monkey and the player has guessed m, o, and e so far. The answer array would look like this ["m", "o", "_", "_", "e", "_"], andanswerArray.join(" ") would be "m o _ _ e _".
Now we have to get a guess from the player and ensure that its a single character.
1 var guess = prompt("Guess a letter, or click Cancel to stop playing.");
2 if (guess === null) { break;
3 } else if (guess.length !== 1) { alert("Please enter a single letter."); } else {
4 // Update the game state with the guess }
At 1, prompt takes a guess from the player and saves it to the variable guess. One of four things will happen at this point.
First, if the player clicks the Cancel button, then guess will be null. We check for this condition at 2 with if (guess === null). If this condition is true, we use break to exit the loop.
The second and third possibilities are that the player enters either nothing or too many letters. If they enter nothing but click OK, guess will be the empty string "". In this case, guess.length will be 0. If they enter anything more than one letter, guess.length will be greater than 1.
At 3, we use else if (guess.length !== 1) to check for these conditions, ensuring that guess is exactly one letter. If its not, we display an alert saying, Please enter a single letter.
The fourth possibility is that the player enters a valid guess of one letter. Then we have to update the game state with their guess using the else statement at 4, which well do in the next section.
Once the player has entered a valid guess, we must update the games answerArray according to the guess. To do that, we add the following code to the else statement:
1 for (var j = 0; j < word.length; j++) {
2 if (word[j] === guess) { answerArray[j] = guess;
3 remainingLetters--; } }
At 1, we create a for loop with a new looping variable called j, which runs from 0 up to word.length. (Were using j as the variable in this loop because we already used i in the previous for loop.) We use this loop to step through each letter of word. For example, lets say word is pancake. The first time around this loop, when j is 0,word[j] will be "p". The next time, word[j] will be "a", then "n", "c", "a", "k", and finally "e".
At 2, we use if (word[j] === guess) to check whether the current letter were looking at matches the players guess. If it does, we use answerArray[j] = guess to update the answer array with the current guess. For each letter in the word that matches guess, we update the answer array at the corresponding point. This works because the looping variable j can be used as an index for answerArray just as it can be used as an index for word,
For example, imagine weve just started playing the game and we reach the for loop at 1. Lets say word is "pancake", guess is "a", and answerArray currently looks like this:
["_", "_", "_", "_", "_", "_", "_"]
The first time around the for loop at 1, j is 0, so word[j] is "p". Our guess is "a", so we skip the if statement at 2 (because "p" === "a" is false). The second time around, j is 1, so word[j] is "a". This is equal to guess, so we enter the if part of the statement. The line answerArray[j] = guess; sets the element at index 1 (the second element) of answerArray to guess, so answerArray now looks like this:
["_", "a", "_", "_", "_", "_", "_"]
The next two times around the loop, word[j] is "n" and then "c", which dont match guess. However, when j reaches 4, word[j] is "a" again. We update answerArray again, this time setting the element at index 4 (the fifth element) to guess. Now answerArray looks like this:
["_", "a", "_", "_", "a", "_", "_"]
The remaining letters dont match "a", so nothing happens the last two times around the loop. At the end of this loop, answerArray will be updated with all the occurrences of guess in word.
For every correct guess, in addition to updating answerArray, we also need to decrement remainingLettersby 1. We do this at 3 using remainingLetters--;. Every time guess matches a letter in word,remainingLetters decreases by 1. Once the player has guessed all the letters correctly, remainingLetters will be 0.
As weve already seen, the main game loop condition is remainingLetters > 0, so as long as there are still letters to guess, the loop will keep looping. Once remainingLetters reaches 0, we leave the loop. We end with the following code:
alert(answerArray.join(" "));alert("Good job! The answer was " + word);
The first line uses alert to show the answer array one last time. The second line uses alert again to congratulate the winning player.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
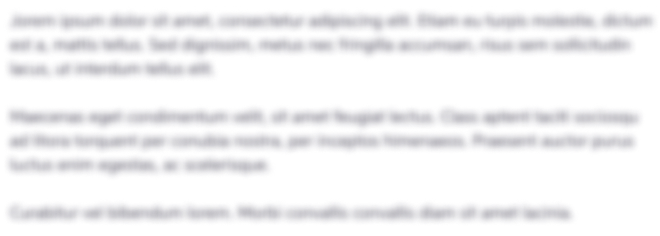
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started