Question
Javascript Assignment: Instructions: Define and assign the variables based upon the instructions in the script tags on this page. Look at the code to see
Javascript Assignment:
Instructions: Define and assign the variables based upon the instructions in the script tags on this page. Look at the code to see where to complete each task. Make sure it is correct.
/*Define and assign the following global variables schoolName assign a value of "UNI" firstName assign a value of your first name lastName assign a value of your last name */ function formatRosterName(in_first_name, in_last_name) { return in_last_name + ", " + in_first_name; } function printSchoolRoster(inFirstName, inLastName, inSchoolName) { let rosterName = formatRosterName(inFirstName, inLastName); let detailLine = rosterName + " " + inSchoolName; return detailLine; } function displayName(fName, lName) { alert("Name:" + fName + lName); }
Javascript
Assignment - Functions & Parameters
Directions:
Instructions: Define and assign the variables based upon the instructions in the script tags on this page.
Example 1
- Create a runtime script that will call formatRosterName().
- Place the script so that it will display the name in the following heading.
- Pass the global variables firstName and lastName into the function.
Student Name: display name here
Example 2
- Create a button called "Display Name".
- Assign an onclick event handler to the button that will call displayName().
- Pass the global variables firstName and lastName into the function.
- Fix the function so that there is proper spacing
Example 3
- Create a function called displaySchool( ).
- the function will accept one input parameter called inValue
- the function will display the input value on the console using console.log().
- Call this function using a button and the onclick event handler. Pass in the schoolName variable as the parameter to the displaySchool().
Example 4
- Create a function called printName( ).
- the function will accept two parameters, inFirstName and inLastName
- concatenate the names into a single string formatted as "firstName lastName"
- display the result string to the console using console.log()
- Call this function during runtime. Pass in the firstName and lastName variables as the parameters.
Example 5
- Create a global variable called totalSales. Assign it a value of 3435.6. It should be numeric data type.
- Create a function called formatCurrency().
- the function will accept one input parameter called inNumber
- the function will use the currency formatting discussed in this unit
- use a 'return' statement to deliver the formatted number to where the function is called
- Use a runtime script to display the formatted number instead of the message in the heading shown below.
Your Total Sales: display formatted value here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
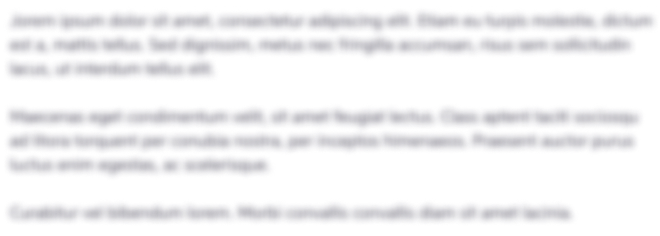
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started