Question
Javascript help! I'm so close, but I cannot get checks to work other than checking if it is blank. I need it to also check
Javascript help! I'm so close, but I cannot get checks to work other than checking if it is blank. I need it to also check if the phone number is valid, the email, and the arrival date.
In this exercise, youll develop a Web application that stores data in session storage. The interface looks like this (this interface has been provided to you in the index.html file -- be sure to rename this file and follow the required course naming conventions):
Then, when you click on the Submit Reservation button, a new page gets the data from session storage and displays it like this:
HTML code:
Reservation Request
My javascript code that I need help on
"use strict"; var $ = function(id) { return document.getElementById(id); };
/////////////////////////////////////////////////////////////////////////////// //validate and store information var saveReservation = function() { var name=document.getElementById("name").value; var phone=document.getElementById("phone").value; var email=document.getElementById("email").value; var nights=document.getElementById("nights").value; var arrival_date=document.getElementById("arrival_date").value; //Validate and store name if(name == "") //if name is blank { nameMessage.innerHTML = "Name cannot be left blank."; return false; }
else { sessionStorage.setItem('name', $("name").value ); //save the name } //////////////////////////////////////////////////////////////////////////// //Validate and store phone number function chkPhone() { var myPhone=document.getElementById("phone").value; var pos=myPhone.value.search(/^\(?([0-9]{3})\)?[-. ]?([0-9]{3})[-. ]?([0-9]{4})$/); if(pos!=0) { return false; } }
if(phone == "") { phoneMessage.innerHTML = "Phone cannot be left blank."; return false; }
else if(chkPhone(phone)) { phoneMessage.innerHTML = "wrong format"; return false; }
else { sessionStorage.setItem('phone', $("phone").value ); //save the phone number value in sessionStorage }
/////////////////////////////////////////////////////////////////////////////////////////// function emailIsValid (email) { var emailTest = (/\S+@\S+\.\S+/); if(!emailTest) { return false; } else { return true; } } //Validate and store email if(email == "") //if email is blank { emailMessage.innerHTML = "Email address cannot be left blank"; return false; } else if(emailIsValid(email)==false) { emailErrorMessage.innerHTML = "Email address cannot be this format"; }
else { sessionStorage.setItem('email', $("email").value ); //save the email value } /////////////////////////////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////////////////////////////// function isValidDate(dateStr) { var datePat = /^(\d{1,2})(\/|-)(\d{1,2})\2(\d{4})$/;
var matchArray = dateStr.match(datePat); // is the format ok? if (matchArray == null) { return false; } month = matchArray[1]; // parse date into variables day = matchArray[3]; year = matchArray[4]; if (month 12) { // check month range return false; } if (day 31) { return false; } if ((month==4 || month==6 || month==9 || month==11) && day==31) { return false } if (month == 2) { // check for february 29th var isleap = (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)); if (day>29 || (day==29 && !isleap)) { return false; } } return true; // date is valid } //Validate and store arrivalDate if(arrival_date === "") //if its blank { dateMessage.innerHTML = "Please Enter the Arrival Date"; return false; }
else if(!isValidDate(arrival_date)) { dateMessage.innerHTML = "Arrival date must be in: mm/dd/year"; return false; }
else { sessionStorage.setItem('arrivalDate', $("arrival_date").value ); //save the arrival_date } ///////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////////////////////////////////////////////// //Validate and store nights if(nights == "") //if its blank { nightMessage.innerHTML = "Please Enter the Number of Nights"; return false; }
else if(isNaN(nights)) //if it is not a number { nightMessage.innerHTML = "Nights must be a number"; return false; }
else { sessionStorage.setItem('nights', $("nights").value ); //save the nights value } ////////////////////////////////////////////////////////////////////////////////////
//store the rest of the items sessionStorage.setItem('adults', $("adults").value ); //adults
sessionStorage.setItem('children', $("children").value ); //children
sessionStorage.setItem('roomType', $("standard").value ); //standard
sessionStorage.setItem('roomType', $("business").value ); //business
sessionStorage.setItem('roomType', $("suite").value ); //suite
sessionStorage.setItem('bedType', $("king").value ); //king
sessionStorage.setItem('bedType', $("double").value ); //double
if($("smoking").checked)//check if the smoking checkbox is selected { sessionStorage.setItem('smoking', 'yes' ); // save the smoking value to yes in sessionStorage }
else { sessionStorage.setItem('smoking', 'no' ); // save the smoking value to no in sessionStorage }
// submit form $("reservation_form").submit(); };
window.onload = function() { $("submit_request").onclick = saveReservation; $("arrival_date").focus(); };
Reservation Request General Information 12/2/2015 Arrival date: Nights Adults Children: 21 0 Preferences Suite Room type Bed type: Smoking Standard Business King Double Double Contact Information Name: Email: Phone: Mary mary@yahoo.com 555-555-5555 Submit Reservation The following reservation has been submitted Name: Mary Phone: 555-555-5555 Email: mary@yahoo.com Arrival Date: 12/2/2015 Nights: 3 Adults: 2 Children: 0 Room Type: business Bed Type: king Smoking: no Reservation Request General Information Arrival date: 12/39/2016 Nights three Adults: Children: 0 Arrival date is invalid. Nights must be a number -Preferences Suite Room type: Bed type: Smoking Standard Business King Double Double -Contact Information Name: Email: Phone: graceyahoo.com 123-456-789 Name is required. Email address is invalid. Phone number is invalid Submit Reservation ClearStep by Step Solution
There are 3 Steps involved in it
Step: 1
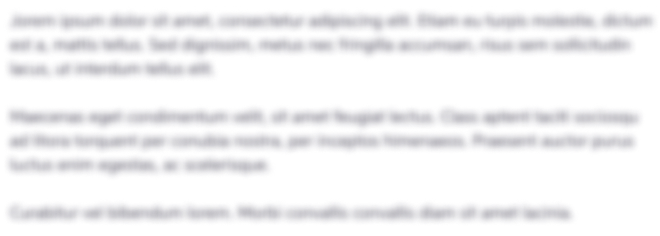
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started