Question
Kaprekar's routine is an algorithm that can be performed on any three-digit number, except for numbers with three of the same digit (such as 111).
Kaprekar's routine is an algorithm that can be performed on any three-digit number, except for numbers with three of the same digit (such as 111). The algorithm always produces the number 495 at the end.
Here are the instructions that describe Kaprekar's routine.
- Construct two new numbers by arranging the digits of n in ascending and descending order.
- Subtract the smaller number from the larger one.
- Repeat the process on the resulting number until you reach 495.
Here is Kaprekar's routine run on the number 251.
- Construct the numbers 125 and 521.
- Subtract 125 from 521, which results in 396.
- Repeat the process on the resulting number (396) until you reach 495:
- 396 --> 963 - 369 --> 594
- 594 --> 954 - 459 --> 495
Note that if the algorithm ever produces a 2 digit number, a zero should be preserved so we consider the number as having three digits. For example, here is Kaprekar's routine run on 898.
- 898 --> 988 - 889 --> 099
- 099 --> 990 - 099 --> 891
- 891 --> 981 - 189 --> 792
- 792 --> 972 - 279 --> 693
- 693 --> 963 - 369 --> 594
- 594 --> 954 - 459 --> 495 With this in mind, write the function stepsToReach495(n), which takes a three-digit integer n, runs Kaprekar's routine on it, and returns the number of times it completed step 2 of the routine before reaching 495.
stepsToReach495(251)will return 3, because we complete step 2 of Kaprekar's routine 3 times before reaching 495. Similarly,stepsToReach495(898)will return 6.
Return None if the routine cannot be completed because the number has three of the same digit.
@testFunction def teststepsToReach495(): assert (stepsToReach495 (495) assert (stepsToReach495 (594) assert (stepsToReach495 (396) == 2) # 963- 369 = 594 # 954 459 495 assert (stepsToReach495 (936) == 2) # Same as 396 assert(stepsToReach495 (251) ==3) # 521 125 396 # 963 369 = 594 # 954 459 = 495 == 0) assert (stepsToReach495 (516) == 1) # 651-156 = 495 assert (stepsToReach495 (891) == 4) assert(stepsToReach495 (898) == 6) # 988 - 889 = 099 #990 899 = 891 #981 189 = 792 # 972 279 = 693 #963 369 = 594 # 954 459 = 495 def main(): == 1) # 954 459 = 495 assert(stepsToReach495 (111) == None) assert(stepsToReach495 (555) == None) assert (stepsToReach495 (777) == None) teststepsToReach495 () main()
Step by Step Solution
3.52 Rating (149 Votes )
There are 3 Steps involved in it
Step: 1
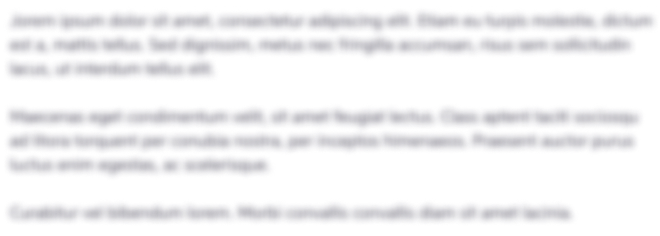
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started