Question
LAB 10 [1] Please complete the classes we have created in the class (Refer to Chapter 9 and follow all steps one by one until
LAB 10
[1] Please complete the classes we have created in the class (Refer to Chapter 9 and follow all steps one by one until you reach EmployeeMain class.) (2 pts) :
(1) Employee class
(2) Lawyer class
(3) Secretary class
(4) Marketer class
(5) LegalSecretary class
(6) EmployeeMain class
[2] Please consider the following class to answer the questions (2 pts).
// Represents a university student
public class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public void setAge(int age) {
this.age = age;
}
} // end of class Student
public class UndergraduateStudent extends Student {
private int year;
...
}
(1) Please complete the UndergraduateStudent with constructor which accepts a name as a parameter and initializes the UndergraduateStudent's state with that name, an age value of 18, and a year value of 0. (1 pt)
(2) Write a version of the setAge method in the UndergraduateStudent class that not only set the age but also increments the year field's value by one. (1 pt)
[3] Assume that the following classes have been defined. (2 pts)
public class Bay extends Lake {
public void method1() {
System.out.print("Bay 1 ");
super.method2();
}
public void method2() {
System.out.print("Bay 2 ");
}
} // end of class Bay
public class Pond {
public void method1() {
System.out.print("Pond 1 ");
}
public void method2() {
System.out.print("Pond 2 ");
}
public void method3() {
System.out.print("Pond 3 ");
}
} // end of class Pond
public class Ocean extends Bay {
public void method2() {
System.out.print("Ocean 2 ");
}
} // end of class Ocean
public class Lake extends Pond {
public void method3() {
System.out.print("Lake 3 ");
}
} // end of class Lake
(1) Please write a class testMain which includes the following code fragment within main() and answer what output is produced. (1 pt)
Pond[] ponds = {new Ocean(), new Pond(), new Lake(), new Bay()};
for (Pond p: ponds) {
p.method1();
System.out.println();
p.method2();
System.out.println();
p.method3();
System.out.println(" ");
}
(2) Suppose that the following variables referring to the classes are declared within main():
Pond var1 = new Bay();
Object var2 = new Ocean();
Please include and execute the following statements within the main(). (1pt)
((Lake) var1).method1();
((Bay) var1).method1();
((Pond) var2).method2();
((Lake) var2).method2();
((Ocean) var2).method3();
[4] Write a class MonsterTruck1 and MonsterTruck2 that are inherited from Car and Truck classes, respectively. Whenever possible, use inheritance to reuse behavior(s) from the superclasses. (4 pts)
Car and Truck classes are as follows
public class Car {
public void m1() {
System.out.println("car 1");
}
public void m2() {
System.out.println("car 2");
}
public String toString() {
return "vroom";
}
} // end of class Car
public class Truck extends Car {
public void m1() {
System.out.println("truck 1");
m2();
}
} // end of class Truck
Behavior of the MonsterTruck1 class inherited from Car:
Method | Method call/Output/Return |
m1 | println "monster 1" super.m1(); |
m2 | println "truck 1" println "car 1" |
toString | return "monster1 vroomvroom" |
Behavior of the MonsterTruck2 class inherited from Truck:
Method | Method call/Output/Return |
m1 | println "monster 2" |
m2 | super.m2(); println "truck 2" println "car 2" |
toString | return "monster2 vroomvroomvroom" |
Write a TestMonsterTruck class with Main() which contains the following statements:
MonsterTruck1 mTruck1 = new MonsterTruck1();
MonsterTruck2 mTruck2 = new MonsterTruck2();
mTruck1.m1();
mTruck1.m2();
System.out.println(mTruck1.toString());
mTruck2.m1();
mTruck2.m2();
System.out.println(mTruck2.toString());
Step by Step Solution
There are 3 Steps involved in it
Step: 1
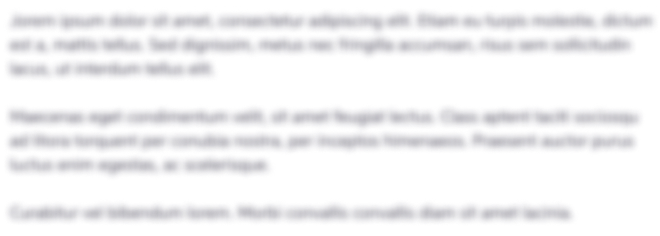
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started