Question
LAB 11 [1] Consider the following method and give the answer in a document that needs to be submitted or you could write a test
LAB 11
[1] Consider the following method and give the answer in a document that needs to be submitted or you could write a test driver (TestMystery class) which contains a main() method as follows: (1pts)
import java.io.*;
import java.util.*;
public class TestMystery {
public static void main(String[] args) {
System.out.println("Answer to mystery(4, 19) equals " + mystery(4, 19));
System.out.println("Answer to mystery(32, 56) equals " + mystery(32, 56));
System.out.println("Answer to mystery(12, 20) equals " + mystery(12, 20));
System.out.println("Answer to mystery(4, 18) equals " + mystery(4, 18));
System.out.println("Answer to mystery(48, 128) equals " + mystery(48, 128));
} // end of main()
public static int mystery(int x, int y) {
if (x % 2 == 1 || y % 2 == 1) {
return 1;
} else {
return 2 * mystery(x / 2, y / 2);
}
} // end of mystery()
} // end of TestMystry
For each call below, indicate what value is returned:
Method Call Value Returned
mystery(4, 19) ______________
mystery(32, 56) ______________
mystery(12, 20) ______________
mystery(4, 18) ______________
mystery(48, 128) ______________
[2] Consider the following method and give the answer in a document that needs to be submitted and you could include this method to the test driver (TestMystery class) and write the following test method calls in main() method: (1pts)
public static int mystery1(int x, int y) {
if (x < y)
return x;
else
return mystery1(x - y, y);
}
For each call below, indicate what value is returned:
Method Call Value Returned
mystery1(6, 13) _______________
mystery1(14, 10) _______________
mystery1(37, 10) _______________
mystery1(8, 2) _______________
mystery1(50, 7) _______________
[3] Consider the following method and give the answer in a document that needs to be submitted and you could include this method to the test driver (TestMystery class) and write the following test method calls in main() method: (1pts)
public static void mystery2(int n) {
if (n <= 1)
System.out.print(n);
else {
mystery2(n / 2);
System.out.print(", " + n);
}
}
For each call below, indicate what output is produced by the method:
Method Call Output Produced
mystery2(1) _______________________
mystery2(2) _______________________
mystery2(3) _______________________
mystery2(4) _______________________
mystery2(16) _______________________
mystery2(30) _______________________
mystery2(100) _______________________
O Recursive Programming
[4] Write a program which contains a main() method and a method multiplyEvens() that returns the product of the first n even integers. (2pts)
For example:
multiplyEvens(1) returns 2
multiplyEvens(2) returns 8 (2 * 4)
multiplyEvens(3) returns 48 (2 * 4 * 6)
multiplyEvens(4) returns 384 (2 * 4 * 6 * 8)
multiplyEvens(5) returns 3840 (2 * 4 * 6 * 8 * 10)
Remark: Do not use a while loop, for loop or do/while loop to solve this problem; you MUST use the recursive mechanism (implicit loop) instead of the external loop structure. The method should check whether the argument is greater than 0 or not first.
public static int multiplyEvens(int n) {
if (n < 1) {
throw new IllegalArgumentException();
} else if (n == 1) {
return 2;
} else {
// Fill your recursive code here
}
}
[Extra Credit] Write recursive methods of the following problems and the associate test driver program. All of them are covered in class. Please refer to the class materials and lecture. (4pts)
(1) Factorial Number : Factorial (int n) : returns n! number. (1pt)
(2) Fibonacci : Fibonacci (int n) : returns n'th Fibonacci number. (1pt)
(3) PowerE : PowerE (int base, int exponent) : returns the based raised to that exponent, n. (1pt)
(4) PrintBinary : PrintBinary (int n) : returns corresponding binary number (String) of the decimal number n. (1pt)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
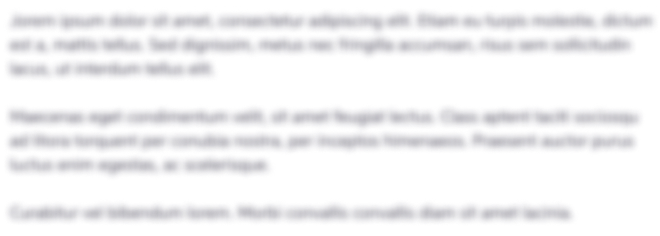
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started