Question
Lab 2: Find & Threaded Find Introduction This assignment will require you to write your version of the find command that will go through the
Lab 2: Find & Threaded Find
Introduction
This assignment will require you to write your version of the "find" command that will go through the filesystem looking for a file based on a string you pass to the command. The goal in this assignment is to build 2 versions of a find command, one will be a direct find that could use a recursive algorithm, and the second version will use threads to accomplish the same goal. You will start your program and pass a directory to start as well as the filename you are looking for. Once you find a file that matches the name you passed into the program you will output the absolute path of the file and do this for every match found.
You should start by building a fully working (non-threaded) version of the find command that works properly. Then copy that into a new program source and modify to add threading in the place of recursion.
To accomplish this lab you will write your program(s) in the C language and use the following libraries to do the directory reading as well as threading:
Directory operations:
- opendir(), readdir(), struct dirent*, ...
File (if it is a directory or not)
- stat(); // this will give the information if it is a directory or not by using the test for
- S_IFDIR
Threading operations:
- pthread_create(), pthread_mutex_lock(), .....
NOTE: When compiling with pthreads, you need to add the following in the command line to bring in the pthread library:
-lpthread
There are 2 goals in this assignment:
- Build a find command to utilize both operating system calls to search across a filesystem (or more) and identify where files that match your search string are and:
- Add threading to do the same thing, but then test the speed of both to see if the threaded one is faster than the non-threaded one.
Major components required in your shell
- Find functionality
- Pass in a directory to start searching from
- Pass in a string of a name you want to "find" in your filesystem on your machine
- Start at the given directory and for each file that matches the search string, print the absolute path + filename to the screen
- Recursive algorithm
- Create a way to search from the initial directory and for each directory found in a given directory, start searching that directory for filenames matching as well
- Each time you pull a filename from a directory entry you will need to test if it is a directory file or other kind of file and if it is a directory, you will need to open that directory and search through that one before going back to the previous directory
- Threading
- Take the "working" find program and add threading in place of the recursive algorithm
- Your goal here is to search many directories "at the same time"
- You will need to spawn detached threads and ensure they properly run without locks or any limitations to see how fast you can make this program vs the non-threaded versions
- Race condition
- In your program you will need to have a variable at "file scope" (global) that will act as the counter for how many files were searched.
- This variable will have the ability to turn off and on through another variable or #define (i.e. sometimes you want to run the program with it on, and others with it off). If you want to send a switch at the command line as with the --threaded below, that would be preferred, but not required.
- The main reason for this variable is to define a thread mutex lock if threading is imposed and the need to count is imposed as well. Other variations will not require this lock as there is no race condition.
- This count variable should start at 0 and increase by 1 each time a file is evaluated to see if it matches the passed in
- Ability to test the speed of each program
- With the use of a program called 'time' or 'timex' you will test the time it takes to find the same filename across your filesystem
- You will need to do this in a way that takes some time, so start at the root of your filesystem as this will take the most time to go through your entire directory/file tree.
- You will need to test 4 conditions
- No thread, no counting
- No thread, counting
- Threading, no counting
- Threading, counting
Find processing
You will run your program as either 2 different programs or with a command line switch to let the program run as normal or threaded. This can be done by accepting commands as follows:
$ myFind
$ myFind --threaded
When the program is initiated the determination is made on whether to thread or not.
Grading for this assignment will be based on the following guidelines:
- Use "script" to capture your testing to a file. To use script, simply type script
at a prompt (filename can be any name and if omitted the file will be called typescript). Once you have entered :
$ script
All I/O will be copied from the screen into the named file, and when you are done simply type "exit" to stop copying to the file.
- NOTE: exit will only stop script, not exit your session.Write a README.TXT file that describes the various commands that your shell will support. This file should contain pertinent information on how you designed your shell, list the commands and usage of the commands, what works and what does not work, as well as who worked on the project with you (no name = no grade).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
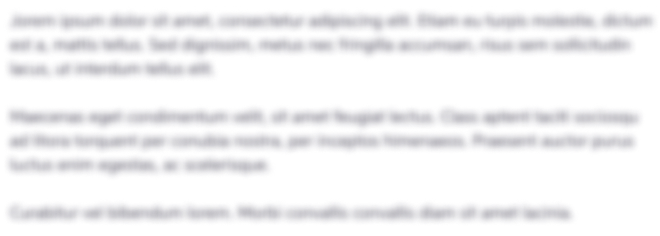
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started