Question
Lab1 Edit-Compile-Test-Edit Algorithms, Errors, and Testing Lab Objectives Be able to write an algorithm in pseudo-code Be able to compile a Java program Be able
Lab1 Edit-Compile-Test-Edit Algorithms, Errors, and Testing
Lab Objectives
Be able to write an algorithm in pseudo-code Be able to compile a Java program Be able to execute a Java program using the Sun JDK or a Java IDE Be able to test a program Be able to debug a program with syntax, run-time, and logic errors. Introduction We will be using Eclipse, an Integrated Development Environment (IDE) for programming in Java. In this lab we will also compile and execute a Java program in the Windows (or Mac) command-line environment using the Java Development Kit (JDK) directly. Follow the directions in the Tasks specified below. In this lab, you are given a program called Function1.java, which calculates the value of a function. Normally, you design a program first, often using pseudocode, and then implement the program based on the design. In this lab, however, you are given a program and are required to write pseudocode from the Java code. This will give you practice with algorithms while allowing you to explore and understand a little Java code while we begin learning the Java programming language. Then you will compile and run function1.java from a console (command-line using the Java JDK directly), and from the Eclipse IDE. This program should compile and run without errors. You will also be debugging a program. You will debug and run a program called Function2.java. This program contains a few syntax errors, run-time errors, and logic errors. You will need to develop test data that will represent all possible kinds of data that the user may enter. There are several types of errors. In this lab, you will encounter syntax, runtime, and logic errors. 1. Syntax Errorserrors in the grammar of the programming language. These are caught by the compiler and listed out with line number and error found. You will learn how to understand what they tell you with experience. All syntax errors must be corrected before the program will run. If the program runs, this does not mean that it is correct, only that there are no syntax errors. Examples of syntax errors are spelling mistakes in variable names, missing semicolons, unpaired curly braces, etc.
2. Run time errorserrors that do not occur until the program is run, and then may only occur with some data. These errors emphasize the need for completely testing your program.
3. Logic Errorserrors in the logic of the algorithm. These errors emphasize the need for a correct algorithm. If the statements are out of order, if there are errors in a formula, or if there are missing steps, the program can still run and give you output, but it may be the wrong output. Since logic errors are not reported by Java, you may not realize you have errors unless you check your output. It is very important to know what output you expect. You should test your programs with different inputs, and know what output to expect in each case. You should usually test your programs with several normal cases, and also with boundary conditions and known erroneous inputs. Calculate each case by hand before running your program so that you know what to expect. You may get a correct answer for one case, but not for another case. This will help you figure out where your logic errors are.
Task #1 Writing an Algorithm
1. Copy the file Function1.java (see code listing 1.1) from Blackboard.
2. Open the file in your Java Integrated Development Environment (IDE) or a text editor. Examine the file, and write pseudocode that provides a guide to the code. You do not need a line of pseudocode for every line of code. The program code includes identifier declarations and a statement that is needed to enable Java to read from the keyboard. These are not part of actually completing the task of calculating the function, so they are not included in the pseudocode. On the other hand, your pseudocode should be detailed enough to allow direct translation into Java. Do not just write find the answer, but write statements that have parallels in Java. See Chapter 1.6, section 3 for an example. There are three principles when writing pseudocode:
a. Purpose: to describe the algorithm, i.e., what happens in code
b. Detail: pseudocode should be detailed enough that a Java programmer could write code from it without having to create parts of the algorithm.
c. Generality: pseudocode should not have all the details of declaring variables, importing libraries, and using correct syntax. Write in simplified English, not in Java. Write your pseudocode in a Word document, and submit it in Blackboard. Task #2 Compile and Execute a Program 1. Compile Function1.java using a command console (see the Lecture notes in Blackboard for specific instructions about compiling and running a Java program in a command console.
2. You should not receive any error messages.
3. When this program is executed, it will ask the user for input. You should calculate several different cases by hand. Since there is a critical point at which the calculation changes, you should test three different cases: the critical point, a number above the critical point, and a number below the critical point. You should calculate by hand first so that you can check the logic of the program. Fill in the chart below with your test cases and the result you get when calculating by hand. x f(x) (hand calculated) f(x) (program result)
x | f(x) (Hand calculated) | f(x) (program results) |
4. Execute the program using your first set of data. Record your result. You will need to execute the program at least three times to test all your data. Note: you do not need to compile again. Once the program compiles correctly once, it can be executed many times. You only need to compile again if you make changes to the code.
5. Take a screen shot of your command console with the program being compiled and running one of the test cases.
6. Now open Eclipse, create a Project, and import the file Function1.java into the src directory of the Project. Check that there are no syntax errors, and run the program from Eclipse. Take another screen shot of the program running one of the test cases.
Task #3 Debugging a Java Program 1. Copy the file Function2.java (see code listing 1.2) from Blackboard and import it into Eclipse into your existing project.
2. Open the file in Eclipse. This file contains a simple Java program that contains errors. Eclipse will automatically compile the program. You should see syntax errors (red lines under a command and a red x show where there are syntax errors). Correct all the syntax errors. Hint: start at the first syntax error, fix it, and work your way down the file. Later errors will often be fixed by correcting an earlier error.
3. OPTIONAL: to see how a command-line compilation reports errors, compile Function2.java in a command console like you did for Task #2, part 1.
4. When all syntax errors are corrected, the program should compile. As in the previous exercise, you need to develop some test data. Use the chart below to record your test data and results when calculated by hand.
5. Execute the program using your test data and record the results. If the output of the program is different from what you calculated, this usually indicates a logic error. Examine the program and correct the logic error. Compile the program and execute using the test data again. Repeat until all output matches what is expected. x f(x) (hand calculated) f(x) (program result) 6. Run Function2 with input of x=0. Java may record a run-time error, or it may print Infinity. In either case, this is a divide-by-zero error. Fix it by putting the following code around the computation and the printout: if (x==0) System.out.println(Divide-by-zero error: try again); else { result = (2*Math.pow(x,3)-5*Math.pow(x,2)-12*x+4)/x; System.out.println("f("+x+")="+result); } Recompile and run the resulting code with x=0 and with some other value of x. Turn in the following:
a. Pseudocode of Function1.java (Word or txt document)
b. Screen shot of command-line compilation and run of Function1.java
c. Screen shot of Eclipse console running Function1.java
d. Test table for Function1 of hand-calculated and program results e. Function2.java with errors corrected (NOT the .class file; submit the .java file) f. Test table for Function2 of hand-calculated and program results
Function1
import java.util.Scanner; /* * This program should compile as written. * Use it to "reverse-engineer" a pseudo-code description of the algorithm as explained in the Lab 1 description. */ public class Function1 { public static void main(String[] args) { //this line creates an object called input from the Java library class Scanner. //System.in specifies the keyboard as the input stream Scanner will use Scanner input = new Scanner(System.in); //initialize the program's variables double result; double x=-999.999; //print out prompts to tell the user what to do System.out.println(" Program to evaluate a function... "); System.out.println("Compute the value of the function:"); System.out.println(" for x>0 f(x)=2*x^3 - 5*x^2 - 12*x + 4"); System.out.println(" for x=0 f(x)=0"); System.out.println(" for x<0 f(x) + 5*x^2 12*x - 4"); system.out.println("enter the value of x for which will be evaluated (numeric values):");>0) { result = 2*Math.pow(x,3)-5*Math.pow(x,2)-12*x+4; } else if (x<0) { result = -2*Math.pow(x,3)+5*Math.pow(x,2)+12*x-4; } else { result = 0; } //print out the result to the console System.out.println("f("+x+")="+result); System.out.println(" Goodbye"); } }
Function2
import java.util.Scanner; /* * This program contains some syntax and logic errors, and depending on the input, contains a run-time error. * Fix the errors as explained in the Lab 1 description. */ public class Function2 { public static void main(String[] args) { //this line creates an object called input from the Java library class Scanner. //System.in specifies the keyboard as the input stream Scanner will use Scanner input = new Scanner(System.in); //initialize the program's variables double result; double x=-999.999; //print out prompts to tell the user what to do System.out.println(" Program to evaluate a function... ") System.out.println("Compute the value of the function f(x)=(2*x^3 - 5*x^2 - 12*x + 4)/x"); System.out.println("Enter the value of x for which f(x) will be evaluated (numeric values):"); //read what the user types and set x to that value x = input.nextDouble(); //use built-in Java library method Math.pow and standard operators to compute the result result = (2*Math.pow(x,2)-5*Math.pow(x,2)-12*x+4)/x; //print out the result to the console System.out.println("f("+x+")="+reslt); System.out.println(" Goodbye"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
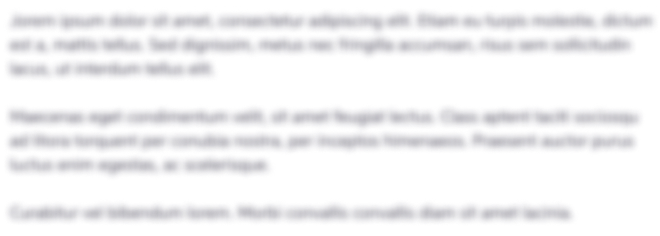
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started