Question
LAB11 Instructions You will modify the provided Employee and ProductionWorker classes so that they throw exceptions when the following errors occur: InvalidEmployeeNumber, InvalidShift, and InvalidPayRate.
LAB11 Instructions
You will modify the provided Employee and ProductionWorker classes so that they throw exceptions when the following errors occur: InvalidEmployeeNumber, InvalidShift, and InvalidPayRate.
In JGrasp, create a project called LAB11:
Before starting this lab, download and run the starter code and verify that the starter code produces the output below.
Download EmployeeStarterCode2.java, ProductionWorkerStarterCode2.java, and WorkerDemoStarterCode2.java from BB, open in JGrasp, and save in project LAB11
Run WorkerDemo and verify runs and outputs
Part I : The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999.
Create the InvalidEmployeeNumber class:
So that it extends Exception: public class InvalidEmployeeNumber extends Exception
The constructor includes: super(ERROR: Invalid employee number.);
Modify the ProductionWorker class:
So that the constructor throws InvalidEmployeeNumber exception
Modify Employee class:
So that the constructor throws InvalidEmployeeNumber
So that the setEmployeeNumber method so that if the employee number is invalid: throw new InvalidEmployeeNumber( )
Modify the WorkerDemo class:
Add in try and catch statements into createWorker to catch an InvalidEmployeeNumber exception:
try { ProductionWorker pw = new ProductionWorker ( n, num, date, sh, rate );
catch ( InvalidEmployeeNumber e ) {
System.out.println(e.getMessage ( ) );
Run WorkerDemo and verify that the code produces the output
Part II : The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift.
Create the InvalidShift class:
So that it extends Exception: public class InvalidShift extends Exception
The constructor includes: super(ERROR: Invalid shift number.);
Modify the ProductionWorker class:
So that the constructor throws InvalidShift exception
So the constructor calls setShift to set the shift, i.e.
replace shift = sh; with setShift ( sh ) ;
So that the setShift method throws InvalidShift exception
Add code to the setShift method so that if the shift is invalid:
throw new InvalidShift ( ) ;
Modify the WorkerDemo class:
Add in try and catch statements into createWorker to catch an InvalidShift exception:
catch ( InvalidShift e ) {
System.out.println(e.getMessage ( ) );
Run WorkerDemo and verify that the code produces the output
Part III : The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for an hourly pay rate.
Create the InvalidPayRate class:
So that it extends Exception: public class InvalidPayRate extends Exception
The constructor includes: super(ERROR: Invalid pay rate.);
Modify the ProductionWorker class:
So that the constructor throws InvalidPayRate exception
So the constructor calls setPayRate to set the shift, i.e.
replace payRate = rate; with setPayRate ( rate ) ;
So that the setPayRate method throws InvalidPayRate exception
Add code to the setPayRate method so that if the pay rate is negative:
throw new InvalidPayRate ( ) ;
Modify the WorkerDemo class:
Add in try and catch statements into createWorker to catch an InvalidPayRate exception:
catch ( InvalidPayRate e ) {
System.out.println(e.getMessage ( ) );
Run WorkerDemo and verify that the code produces the output
Your submission to BB should be your Employee.java, InvalidEmployeeNumber.java, InvalidPayRate.java, InvalidShift.java, ProductionWorker.java and WorkerDemo.java files only, Not included in a .zip file or a .gpj file
Here are the starter codes
package lab11startercode2; /** * * @author GHouseman */ /** The Employee class stores data about an employee for the Employee and ProductionWorker Classes programming challenge. */ public class Employee { private String name; // Employee name private String employeeNumber; // Employee number private String hireDate; // Employee hire date /** This constructor initializes an object with a name, employee number, and hire date. @param n The employee's name. @param num The employee's number. @param date The employee's hire date. */ public Employee(String n, String num, String date) { name = n; setEmployeeNumber(num); hireDate = date; } /** The no-arg constructor initializes an object with null strings for name, employee number, and hire date. */ public Employee() { name = ""; employeeNumber = ""; hireDate = ""; } /** The setName method sets the employee's name. @param n The employee's name. */ public void setName(String n) { name = n; } /** The setEmployeeNumber method sets the employee's number. @param e The employee's number. */ public void setEmployeeNumber(String e) { if (isValidEmpNum(e)) employeeNumber = e; else employeeNumber = ""; } /** The setHireDate method sets the employee's hire date. @param h The employee's hire date. */ public void setHireDate(String h) { hireDate = h; } /** The getName method returns the employee's name. @return The employee's name. */ public String getName() { return name; } /** The getEmployeeNumber method returns the employee's number. @return The employee's number. */ public String getEmployeeNumber() { return employeeNumber; } /** The getHireDate method returns the employee's hire date. @return The employee's hire date. */ public String getHireDate() { return hireDate; } /** isValidEmpNum is a private method that determines whether a string is a valid employee number. @param e The string containing an employee number. @return true if e references a valid ID number, false otherwise. */ private boolean isValidEmpNum(String e) { boolean status = true; int num = 0; // First, try to convert e to an integer try{ num = Integer.parseInt(e); } catch (IllegalArgumentException ex) { status = false; } // If e is an integer, determine whether it is // in the range of 0 through 9.999 if (status == true){ if (num < 0 || num > 9999) { status = false; } } // Return the status. return status; } /** toString method @return A reference to a String representation of the object. */ public String toString() { String str = "Name: " + name + " Employee Number: "; if (employeeNumber == "") str += "INVALID EMPLOYEE NUMBER"; else str += employeeNumber; str += (" Hire Date: " + hireDate); return str; } }
package lab11startercode2; /** * * @author GHouseman */ /** The ProductionWorker class stores data about an employee who is a production worker for the Employee and ProductionWorker Classes programming challenge. */ public class ProductionWorker extends Employee { // Constants for the day and night shifts. public static final int DAY_SHIFT = 1; public static final int NIGHT_SHIFT = 2; private int shift; // The employee's shift private double payRate; // The employee's pay rate /** This constructor initializes an object with a name, employee number, hire date, shift, and pay rate @param n The employee's name. @param num The employee's number. @param date The employee's hire date. @param sh The employee's shift. @param rate The employee's pay rate. */ public ProductionWorker(String n, String num, String date, int sh, double rate) { super(n, num, date); shift = sh; payRate = rate; } /** The no-arg constructor initializes an object with null strings for name, employee number, and hire date. The day shift is selected, and the pay rate is set to 0.0. */ public ProductionWorker() { super(); shift = DAY_SHIFT; payRate = 0.0; } /** The setShift method sets the employee's shift. @param s The employee's shift. */ public void setShift(int s) { shift = s; } /** The setPayRate method sets the employee's pay rate. @param p The employee's pay rate. */ public void setPayRate(double p) { payRate = p; } /** The getShift method returns the employee's shift. @return The employee's shift. */ public int getShift() { return shift; } /** The getPayRate method returns the employee's pay rate. @return The employee's pay rate. */ public double getPayRate() { return payRate; } /** toString method @return A reference to a String representation of the object. */ @Override public String toString() { String str = super.toString(); str += " Shift: "; if (shift == DAY_SHIFT) str += "Day"; else if (shift == NIGHT_SHIFT) str += "Night"; else str += "INVALID SHIFT NUMBER"; str += " Hourly Pay Rate: "; if (payRate > 0) str += String.format("$%,.2f",payRate); else str += "INVALID PAY RATE"; return str; } }
package lab11startercode2; /** * * @author GHouseman */ /** This program demonstrates a solution to the Employee and ProductionWorker Classes programming challenge. */ public class WorkerDemo { public static void main(String[] args) { String shift; // To hold a shift value // Create a ProductionWorker object with valid data System.out.println("Employee with valid employee number, valid shift " + "number, valid pay rate ..."); createWorker("John Smith", "123", "11-15-2005", ProductionWorker.DAY_SHIFT, 16.50); // Try to use an invalid employee number System.out.println(); System.out.println("Attempting an invalid employee number..."); createWorker("John Smith", "10001", "11-15-2005", ProductionWorker.DAY_SHIFT, 16.50); // Try to use an invalid shift number System.out.println(); System.out.println("Attempting an invalid shift number..."); createWorker("John Smith", "123", "11-15-2005", 66, 16.50); // Try to use an invalid pay rate System.out.println(); System.out.println("Attempting an invalid pay rate..."); createWorker("John Smith", "123", "11-15-2005", ProductionWorker.DAY_SHIFT, -99.50); } /** * createWorker method * @param n * @param num * @param date * @param sh * @param rate */ public static void createWorker(String n, String num, String date, int sh, double rate){ ProductionWorker pw; pw = new ProductionWorker(n, num, date, sh, rate); System.out.println(pw); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
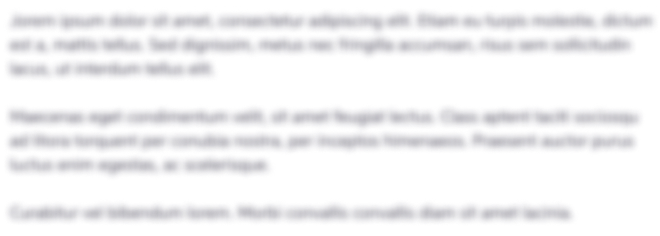
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started