Question
Language: C++ Description: Write a program to simulate image processing. An image file is simulated by a 2D array of ints. Shell code is provided
Language: C++
Description:
Write a program to simulate image processing. An image file is simulated by a 2D array of ints. Shell code is provided in below. The program includes the following functions:
Open an image file (input a 2D array of ints from a text file). The first two numbers from the file are the dimension of the image (2D array), the first number is height (# of rows) and the second number is width (# of columns). Next comes the 2D array stored in row order (row 0, row1, ). There should be (# of rows) (# of columns) integers. Sometimes an image file has been corrupted (not invalid dimension or not enough numbers in the file). You need to test and report such a file.
Process an image file (blur the image).
Print out blurred image on screen.
You need to add code to ADD #1-3:
ADD #1: declaration of function blur
ADD #2: implementation of function openOneImage. Your function should work as specified in the given declaration (pre, post conditions).
ADD #3: implementation of function blur
Your code should also contain:
Algorithms (pseudo code) for functions openOneImage and blur. Add them as block comments at the beginning of the source code file.
Pre- and Post- condition comments for each function.
/*IN*/, /*OUT*/, /*INOUT*/ comments to function parameters
Youre not supposed to modify the given code in other unspecified manners.
The screenshot below shows a sample run on image1.txt Images link (https://www.sendspace.com/filegroup/loNvuNvRglNkfpIZOVk5rNg%2BdL1y0TzB)
Input image file:
Approach 1: pixels without certain neighbors are not processed
Blurred result:
Test your program with the four testing cases (four image?.txt files in link provided above) and provide screenshots of your running program. Explain how each testing case is different.
Code:
// image processing // open an image file. blur the image. print it on screen
#include #include // std::ifstream #include // std::string #include // std::setw()
//---------------------------------------------- // global declarations //----------------------------------------------
// return values const int SUCCESS = 0; const int ERR_OPENFILE_FAILED = 2; const int ERR_CORRUPTED_FILE = 4;
// max dimension of image (2D array) const int MAX_ROW = 100; const int MAX_COL = 100;
//---------------------------------------------- // function declarations //----------------------------------------------
// read one image (2D array) file from input file int openOneImage(std::string filename/*IN*/, int pic[][MAX_COL]/*OUT*/, int& height, int& width); // Pre: filename initialized // Post: return ERR_FAILED_OPEN if file can't open // return ERR_CORRUPTED_FILE if invalid data (invalid dimension data or not enough image data) from file // return SUCCESS if file opened and an image (2D array) read successfully. // pic would store the image, height stores # of rows, and width stores # of columns
// blur an image // ADD #1
// print an image to output stream obj out void printOneImage(std::ostream& out/*INOUT*/, const int pic[][MAX_COL]/*IN*/, int height/*IN*/, int width/*IN*/); // Pre: pic filled with height x width numbers // out has been openned successfully // Post: image printed to output stream object out. The height and width are printed first and then the image file data is printed
//----------------------------------------------
int main() { int image[MAX_ROW][MAX_COL] = {0}; // one image int imgHeight; // height of image int imgWidth; // width of image
std::string filename = "image1.txt";
// load an image int result = openOneImage(filename, image, imgHeight, imgWidth);
if (result == ERR_OPENFILE_FAILED) { std::cerr
if (result == ERR_CORRUPTED_FILE) { std::cerr
// process the image blur(image, imgHeight, imgWidth); printOneImage(std::cout, image, imgHeight, imgWidth);
return 0; } // end main
//---------------------------------------------- // Function Implementation //----------------------------------------------
// read one image (2D array) file from input file int openOneImage(std::string filename/*IN*/, int pic[][MAX_COL]/*OUT*/, int& height, int& width) { // ADD #2
return SUCCESS; } // end readOneImage
// blur an image // ADD #3
// print an image to output stream obj out void printOneImage(std::ostream& out/*INOUT*/, const int pic[][MAX_COL]/*IN*/, int height/*IN*/, int width/*IN*/) { out
} // end printOneImage
4 6 10 100 10 100 10 100 10 100 10 100 10 100 100 10 100 10 100 10 100 10 100 10 100 10 4 6 10 100 10 100 10 100 10 100 10 100 10 100 100 10 100 10 100 10 100 10 100 10 100 10Step by Step Solution
There are 3 Steps involved in it
Step: 1
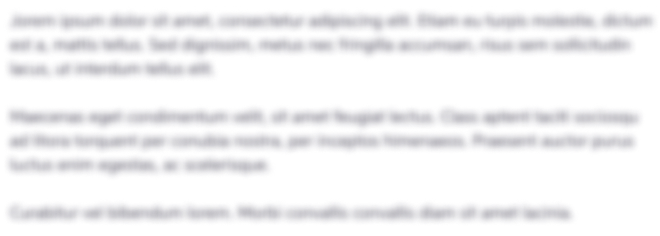
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started