Question
Language = C -------------------------------------------------------------------------------------------------- /* File is createBinary.c Purpose: a program that creates a binary file for testing the prime number program input: a sequence
Language = C
--------------------------------------------------------------------------------------------------
/*
File is createBinary.c
Purpose:
a program that creates a binary file for testing the prime number program
input:
a sequence of numbers to be tested
output:
0 - if success
1 - if an error occured
a binary file with the name.
Assumption:
1. the program does not check if the number is a positive integer
2. the program overwrites that file testPrime.bin
*/
/**************************************************************/
// INCLUDE FILES
//
#include "stdio.h"
#include "stdlib.h"
#include
/*************************************************************/
// PROTYPES
//
/*************************************************************/
int main(int argc, char *argv[])
{
int i;
FILE *fd1;
char s[32];
unsigned int n;
int rc = 0;
unsigned int number;
if (argc
printf(" Usage: %s filename number number number etc. ",argv[0]);
return(1);
}
fd1 = fopen(argv[1],"wb");
if (fd1 == NULL) {
printf("error when openning file %s ",argv[1]);
return(1);
}
for (i = 2; i
n = strtoul(argv[i],NULL,10);
rc = fwrite(&n, sizeof(unsigned int), 1, fd1);
if (rc != 1) {
printf("error when writing to file %s ",argv[1]);
if (fd1 != NULL) fclose(fd1);
return(1);
}
}
if (fd1 != NULL) fclose(fd1);
exit(0);
}
---------------------------------------------------------------------------------------
/*
File is isPrime.c
Purpose:
a program that checks if a given number is a prime number
input:
number - a positive int entered via the command line parameters. For example isPrime 1234
output:
0 - if the input number is not a prime number
1 - if the input number is a prime number
2 - if the command line parameter is not correct
Assumption:
the program does not check if the number is a positive integer
*/
/**************************************************************/
// INCLUDE FILES
//
#include "stdio.h"
#include "stdlib.h"
#include
/*************************************************************/
// PROTYPES
//
int isPrime(unsigned int number);
/*************************************************************/
int main(int argc, char *argv[])
{
int i;
unsigned int number;
if (argc
else number = atoi(argv[1]);
if (isPrime(number)) {
exit(1);
}
exit(0);
}
/*************************************************************/
/*
Purpose: check if the input number is a prime number
input:
number - the number to be checked
return:
0 - if the number is not a prime number
1 - if the number is a prime number
*/
int isPrime(unsigned int number)
{
int i;
for(i = 2; i*i
usleep(100);
if (number % i == 0) {
return(0);
}
}
printf("%d is a prime number ",number);
return(1);
}
-------------------------------------prime.txt---------------------------------------------------
35788631
299902247
961751257
13417
299905653
2356985123
152648967
12397854
200003627
299905657
199905059
299902243
1. (10 pts.) Create a program singlePrime that accepts the binary file name as a single command line parameter. Here you can use 1.1. (5 pts.) The program will check that a file name was provided as command line parameter. If not file was provided it will 1.2. (5 pts.) The program will check if the file exists. If the file does not exist then the program should produce an error 2. (10 pts.) Create a function morph) which will take as input a string (the input number) and morph itself to the isPrime program the tutorial tools that you developed. Name the code file singlePrime.c, print how to use the program -Usage: singlePrime fileName. See below for correct program return code message: file file.bin does not exist, where file.bin is the provided file name. See below for correct program return code. using the excev or execvp system function call. 2.1. Prototype int morph(char *number; 2.2. Input: unsigned number to be checked 2.3. Return -1 if the morph failed (10 pts.) If the binary file exists then The program should read the first unsigned integer from the file, convert it to a string using the function sprintf) and then call the morph function that you created. (5 pts.) Program return codes: 4.1. 0-if the input number is not a prime number 4.2. 1- if the input number is a prime number 4.3. 2- if file name was not provided 4.4. 3- if the file does not exist (5 pts.) Create a makefile to compile the program. The make file name should be Makefile1. The executable program name should be singleMorph. Test your program. Here you are provided with: a. a binary file prime.bin which contains 12 unsigned integer in a binary format. b. a utility to create a binary file (the utility is createBinary.c) 3. 4. 5. 6. 1. (10 pts.) Create a program singlePrime that accepts the binary file name as a single command line parameter. Here you can use 1.1. (5 pts.) The program will check that a file name was provided as command line parameter. If not file was provided it will 1.2. (5 pts.) The program will check if the file exists. If the file does not exist then the program should produce an error 2. (10 pts.) Create a function morph) which will take as input a string (the input number) and morph itself to the isPrime program the tutorial tools that you developed. Name the code file singlePrime.c, print how to use the program -Usage: singlePrime fileName. See below for correct program return code message: file file.bin does not exist, where file.bin is the provided file name. See below for correct program return code. using the excev or execvp system function call. 2.1. Prototype int morph(char *number; 2.2. Input: unsigned number to be checked 2.3. Return -1 if the morph failed (10 pts.) If the binary file exists then The program should read the first unsigned integer from the file, convert it to a string using the function sprintf) and then call the morph function that you created. (5 pts.) Program return codes: 4.1. 0-if the input number is not a prime number 4.2. 1- if the input number is a prime number 4.3. 2- if file name was not provided 4.4. 3- if the file does not exist (5 pts.) Create a makefile to compile the program. The make file name should be Makefile1. The executable program name should be singleMorph. Test your program. Here you are provided with: a. a binary file prime.bin which contains 12 unsigned integer in a binary format. b. a utility to create a binary file (the utility is createBinary.c) 3. 4. 5. 6Step by Step Solution
There are 3 Steps involved in it
Step: 1
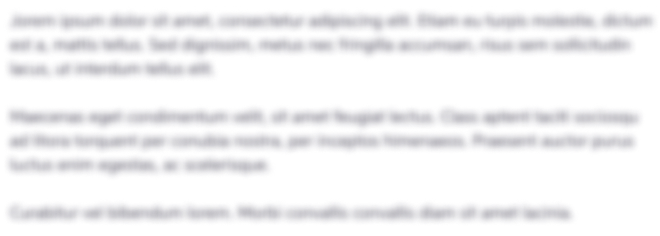
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started