Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// Lesson 4 - Linked List Practice #include #include using namespace :: std ; const int NUMBER_NAMES = 19 ; const string names[NUMBER_NAMES] = {
// Lesson 4 - Linked List Practice #include#include using namespace::std; const int NUMBER_NAMES = 19; const string names[NUMBER_NAMES] = { " printForward"," printReverse"," length"," lengthR"," sum"," max"," min"," countValueOccurences"," addFront", " addBack","removeFront","removeBack","clear","isSorted","isSortedR","hasDuplicate","equal","equalR","search" }; struct node { int data; node * next; }; void printForward(const node *); void printReverse(const node *); int length(const node *); // count the number of nodes in the linked list int lengthR(const node *); int sum(const node *); // sum the data values in the linked list int max(const node *); // assume linked list is not empty int min(const node *); // assume linked list is not empty int countValueOccurences(const node *, int valueToTally); void addFront(node * &, int); void addBack(node * &, int); void removeFront(node * &); // if the list is empty, just return void removeBack(node * &); // if the list is empty, just return void clear(node * &); // return all heap memory bool isSorted(const node *); // check if in ascending order, ok to have adjacent equal values bool isSortedR(const node *); bool hasDuplicate(const node *); bool equal(const node *, const node *); // same number of members and all corresponding members are equal bool equalR(const node *, const node *); const node * search(const node *, int); // return the addres of the first node found holding the dat value, returnn nullptr if not found // Utility functions written by instructor void buildSpecificLinkedList(node * & start); void buildFromArray(node * & start, const int * theArray, int howMany); void returnMemory(node * & start); int menu(); void main() { node * testLL = nullptr; int testData[10] = { 8,2,9,6,2,12,2,6,1,7 }; buildFromArray(testLL, testData, 10); int choice = 0; while (choice != -1) { choice = menu(); switch (choice) { case 0: cout << "testing " << names[choice] << endl; printForward(testLL); break; case 1: cout << "testing " << names[choice] << endl; printReverse(testLL); break; case 2: cout << "testing " << names[choice] << endl; cout << "length is " << length(testLL) << endl; break; case 3: cout << "testing " << names[choice] << endl; cout << "length is " << lengthR(testLL) << endl; break; case 4: cout << "testing " << names[choice] << endl; cout << "sum is " << sum(testLL) << endl; break; case 5: cout << "testing " << names[choice] << endl; cout << "max is " << max(testLL) << endl; break; case 6: cout << "testing " << names[choice] << endl; cout << "min is " << min(testLL) << endl; break; case 7: cout << "testing " << names[choice] << endl; int valueToCount; cout << "Enter value to tally "; cin >> valueToCount; cout << "tally is " << countValueOccurences(testLL,valueToCount) << endl; break; case 8: cout << "testing " << names[choice] << endl; int valueToAddFront; cout << "Enter the value to add at the front "; cin >> valueToAddFront; addFront(testLL, valueToAddFront); break; case 9: cout << "testing " << names[choice] << endl; int valueToAddBack; cout << "Enter the value to add at the back "; cin >> valueToAddBack; addFront(testLL, valueToAddBack); break; case 10: cout << "testing " << names[choice] << endl; removeFront(testLL); break; case 11: cout << "testing " << names[choice] << endl; removeBack(testLL); break; case 12: cout << "testing " << names[choice] << endl; clear(testLL); break; case 13: cout << "testing " << names[choice] << endl; cout << "is sorted " << isSorted(testLL) << endl; break; case 14: cout << "testing " << names[choice] << endl; cout << "is sorted R " << isSortedR(testLL) << endl; break; case 15: cout << "testing " << names[choice] << endl; cout << "has duplicate " << hasDuplicate(testLL) << endl; break; case 16: cout << "testing " << names[choice] << endl; // need code to test equal break; case 17: cout << "testing " << names[choice] << endl; // need code to test equarR break; case 18: cout << "testing " << names[choice] << endl; int valueToSearchFor; cout << "Enter value to search for "; cin >> valueToSearchFor; cout << "value found in node with address " << search(testLL, valueToSearchFor) << endl; break; default: cout << "Invalid choice" << endl; break; }; // end switch } // end while } // end main // function calls written below void printForward(const node * start) { if (start == nullptr) { cout << "Empty Linked List" << endl; return; } const node * walker = start; while (walker != nullptr) { cout << walker->data << ' '; walker = walker->next; } cout << endl; return; } void printReverse(const node * start) { return; } int length(const node * start) { const node * temp = start; int count=0; while (temp!=NULL) { count++; temp = temp->next; } return count; } int lengthR(const node * start) { return -1; } int sum(const node *start) { if (start==NULL) return 0; else return start->data + sum(start->next); } int max(const node *start) { const node * temp = start; int mx=0; while (temp!=NULL) { mx=max(mx, temp->data); temp = temp->next; } return mx; } int min(const node * start) { const node * temp = start; int mn=0; while (temp!=NULL) { mn=min(mn, temp->data); temp = temp->next; } return mn; } int countValueOccurences(const node * start, int valueToTally) { const node * temp = start; int count=0; while (temp!=NULL) { if (temp->data == valueToTally) count++; temp=temp->next; } return count; } void addFront(node * & start, int value) { return; }; void addBack(node * & start, int value) { return; } void removeFront(node * & start) { return; } void removeBack(node * &) { return; } void clear(node * & start) { return; } bool isSorted(const node * start) { return false; } bool isSortedR(const node * start) { return false; } bool hasDuplicate(const node * start) { return false; } bool equal(const node * left, const node * right) { return false; } bool equalR(const node * left, const node * right) { return false; } const node * search(const node * start, int valueToSearchFor) { return nullptr; } // ******************************************************************************************* // void buildSpecificLinkedList(node * & start) // start--> 8 --> 4 --> 15 --> 7 { start = new node; start->data = 8; start->next = new node; start->next->data = 4; start->next->next = new node; start->next->next->data = 15; start->next->next->next = new node; start->next->next->next->data = 7; start->next->next->next->next = nullptr; } void returnMemory(node * & start) { if (start == nullptr) { cout << "Empty Linked List" << endl; return; } node * walker = start; node * previous = nullptr; while (walker != nullptr) { cout << "deleting " << walker -> data << endl; previous = walker; walker = walker->next; previous->next = nullptr; delete previous; } start = nullptr; } void buildFromArray(node * & start, const int * theArray, int howMany) { if (howMany <= 0) { start = nullptr; return; } start = new node; start->data = theArray[0]; node * walker = start; for (int i = 1; i < howMany; i++) { walker->next = new node; walker->next->data = theArray[i]; walker->next->next = nullptr; walker = walker->next; } } int menu() { cout << endl << endl; cout << "Select for the following = use -1 to quit" << endl; for (int i = 0; i < NUMBER_NAMES; i++) cout << i << ' ' << names[i] << endl; int choice; cin >> choice; return choice; }
I need help with the remaining functions?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
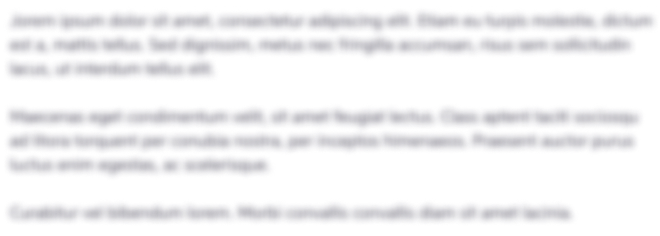
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started