Question
LibraryMain.java: import java.util.Scanner; /* This application manages a collection of media items for a user. The items * are representeed by MediaItem objects. The items
LibraryMain.java:
import java.util.Scanner; /* This application manages a collection of media items for a user. The items * are representeed by MediaItem objects. The items are managed by a MediaList object. * Their are currently three types of MediaItems: books (electronic format), movies, and songs. * Each type is modeled as a subclass of MediaItem. * A user has several options that are displayed in a menu format. * This class runs a console interface between a user and the mediaList */ import java.io.*;
public class LibraryMain { public static void main(String[] args) throws IOException{ System.out.println("My Media Library"); Scanner scan = new Scanner(System.in); MediaList mediaList = new MediaList(5); boolean keepGoing = true; String userStr = ""; int position; while(keepGoing) { System.out.println("Main Menu:"); System.out.println("Enter A to add a new media item."); System.out.println("Enter F to find media items."); System.out.println("Enter P to view all media items."); System.out.println("Enter X to quit."); System.out.println(""); userStr = scan.nextLine(); if (userStr.equalsIgnoreCase("A")){ MediaItem item = null; System.out.println("Enter the title: "); String title = scan.nextLine(); System.out.println("Enter the author/artist/director: "); String author = scan.nextLine(); System.out.println("Enter the genre: "); String genre = scan.nextLine(); System.out.println("What type of media? Enter B for book, M for movie, S for song: "); String type = scan.nextLine(); if(type.equalsIgnoreCase("B")){ System.out.println("Enter the number of pages: "); String pages = scan.nextLine(); System.out.println("Enter the preferred font size: "); String font = scan.nextLine(); item = new Book(title, author, genre, Integer.parseInt(pages), Double.parseDouble(font)); } else if(type.equalsIgnoreCase("M")){ System.out.println("Enter the playing time (minutes): "); String playTime = scan.nextLine(); System.out.println("Enter the lead actor: "); String actor = scan.nextLine(); System.out.println("Enter the release year YYYY: "); String year = scan.nextLine(); item = new Movie(title, author, genre, Integer.parseInt(playTime), actor, year); } else if(type.equalsIgnoreCase("S")){ System.out.println("Enter the playing time (minutes): "); String playTime = scan.nextLine(); System.out.println("Enter the album: "); String album = scan.nextLine(); System.out.println("Enter the label: "); String label = scan.nextLine(); item = new Song(title, author, genre, Double.parseDouble(playTime), album, label); } else System.out.println("Unrecognized type."); mediaList.addItem(item); } else if (userStr.equalsIgnoreCase("F")){ System.out.println("Enter T to search by title, G to search by genre:"); userStr = scan.nextLine(); if(userStr.equalsIgnoreCase("T")){ System.out.println("Enter the title:"); String title = scan.nextLine(); MediaItem[] items = mediaList.getItemsByTitle(title); if(items.length>0){ String listStr = getItemListAsString(items); System.out.println(listStr); } else System.out.println("Could not find "+title+" in the list."); } else if(userStr.equalsIgnoreCase("G")){ System.out.println("Enter the genre:"); String genre = scan.nextLine(); MediaItem[] items = mediaList.getItemsByGenre(genre); if(items.length>0){ String listStr = getItemListAsString(items); System.out.println(listStr); } else System.out.println("Could not find "+genre+" in the list."); } else System.out.println("Unrecognized search type."); } else if (userStr.equalsIgnoreCase("P")){ System.out.println("Your media: "); MediaItem[] items = mediaList.getItemList(); String listStr = getItemListAsString(items); System.out.println(listStr); } else if(userStr.equalsIgnoreCase("X")) keepGoing = false; else System.out.println("Unrecognized input."); } System.out.println("Bye for now."); scan.close(); } /* This method returns a String which consists of the String * representations of all MediaItems in the array passed in. * Assume the itemList does not contain NULL values. * Assume the itemList is not null, and length >=0. */ public static String getItemListAsString(MediaItem[] itemList){ StringBuilder sb = new StringBuilder(); if(itemList.length>0){ for(int i=0; i MediaList.java /* This class encapsulates a list of media items in a user's collection. * The list is implemented as an array of type MediaItem. * Media items are either a book (electronic format), movie, or song. * Each type of media item is represented by an instance of the Book, Movie, or Song class. * These three classes are subclasses of MediaItem. The array stores media items as * references of type MediaItem. */ public class MediaList { //Class member variable declarations: /* Constructor that initializes the member variables. The array is * created using the initial length passed in to the constructor. * The initialLength is assigned to the initial length passed in to the constructor. * The numItems is initialized to 0. * Any other member variables are initialized as well. */ public MediaList(int initialLen){ } /* Add the newItem passed in to the next available cell in the itemList. * Available cells have the vaue NULL. The numItems variable may be used * to keep track of the index of the next available cell. * For example, if the list contained: item1, item2, NULL, NULL, * the next available cell is at index 2. */ public void addItem(MediaItem newItem){ } /* This method returns an array that contains only MediaItem objects whose * title matches the targetTitle passed in. * The array returned does not contain any NULL values. * This method returns an array of length 0 if there are no matches. * This method may call the getOnlyItems method. */ public MediaItem[] getItemsByTitle(String targetTitle){ return null; } /* This method returns an array that contains only MediaItem objects whose * genre matches the targetGenre passed in. * The array returned does not contain any NULL values. * This method returns an array of length 0 if there are no matches. * This method may call the getOnlyItems method. */ public MediaItem[] getItemsByGenre(String targetGenre){ return null; } /* This method returns an array of all of the MediaItem objects that are * stored in the itemList. The array returned does not contain any NULL * values. This method returns an array of length 0 if the itemList is empty. * This method may call the getOnlyItems metho */ public MediaItem[] getItemList(){ return null; } /* Returns the number of items stored in the itemList. */ public int getNumItems(){ return -5; } /* Returns true if the itemList contains no MediaItems, false otherwise. */ public boolean isEmpty(){ return false; } /****** Private, "helper" method section ******/ /* Creates a new array that is double the size of the array passed in, copies the data * from that array to the new array, and returns the new array. * Note that the new array will contain the items from the previous array followed by NULL values. */ private MediaItem[] expandList(MediaItem[] inputList){ return null; } /* A full item list is an array where all cells contain an item. That * means there is no cell that contains NULL. * This method returns true if all cells in the array contain a String * object, false otherwise. */ private boolean isFull(){ return true; } /* * This method takes an array of MediaItems as an input as well as * the number of MediaItems on that array. The input array may contain * some NULL values. * This method returns an array that contains only the MediaItems in * the input array and no NULL values. * It returns an array of length 0 if there are no MediaItems in the input array. */ private MediaItem[] getOnlyItems(MediaItem[] inputArray, int size){ return null; } } MediaItem.java /** * This class encapsulates the data required to represent a MediaItem. * The attributes common to all MediaItems are: title, author and genre. * Subclasses should override the toString method. **/ public class MediaItem { private String title; private String author; private String genre; /* Subclasses may add specific parameters to their constructor's * parameter lists. */ public MediaItem(String title, String author, String genre){ this.title = title; this.author = author; this.genre = genre; } // get method for the title public String getTitle(){ return title; } // get method for the author public String getAuthor(){ return author; } // get method for the genre public String getGenre(){ return genre; } // Subclasses should override. public String toString(){ return title+", "+author+", "+genre; } } Book.java /** * This class encapsulates the data required to represent a book in electronic format * in a collection of MediaItems. It derives from MediaItem. * In addition to its superclass attributes, the attributes of a book are: * number of pages and font size. **/ public class Book extends MediaItem { private int numPages; private double fontSize; /* Constructor. */ // get method for the number of pages // get method for the font size /* Override the superclass toString method. Use a call to the superclass toString method * to get the superclass attributes. * The return should have the format: * Book: [title], [author], [genre], [numPages], [fontSize] * For example: * "Book: Snow Crash, Neil Stephenson, sci fi, 480, 3.5" */ } Movie.java /** * This class encapsulates the data required to represent a movie in a collection * of MediaItems. It derives from MediaItem. * In addition to its superclass attributes, the attributes of a movie are: * playing time, lead actor, and year of release in the form YYYY (i.e. 2018). **/ public class Movie extends MediaItem { private int playTime;// in minutes private String leadActor; private String releaseYear; //in the form YYYY /* Constructor */ // get method for the playing time // get method for the lead actor // get method for the release year /* Override the superclass toString method. Use a call to the superclass toString method * to get the superclass attributes. * The return should have the format: * Movie: [title], [author], [genre], [playTime], [leadActor], [releaseYear] * For example: * "Movie: Black Panther, Coogler, fantasy, 134, Chadwick Boseman, 2018" */ } Song.java /** * This class encapsulates the data required to represent a song in a collection * of MediaItems. It derives from MediaItem. * In addition to its superclass attributes, the attributes of a song are: * playing time, album, and label. **/ public class Song extends MediaItem { private double playTime;//minutes private String album; private String label; /* Constructor */ // get method for the play time // get method for the album // get method for the label /* Override the superclass toString method. Use a call to the superclass toString method * to get the superclass attributes. * The return should have the format: * Song: [title], [author], [genre], [playTime], [album], [label] * For example: * "Song: Humble, Kendrick Lamar, hip hop, 2.57, Damn, Top Dawg" */ }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
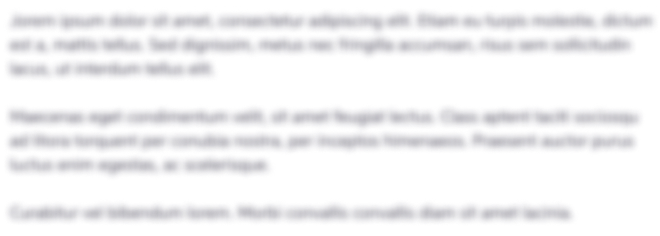
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started