Question
Link 1 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.VBox; import javafx.scene.layout.FlowPane; import javafx.scene.text.Text; import javafx.geometry.Pos; import javafx.geometry.Insets; import javafx.event.ActionEvent; /** * Project
Link 1
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.VBox; import javafx.scene.layout.FlowPane; import javafx.scene.text.Text; import javafx.geometry.Pos; import javafx.geometry.Insets; import javafx.event.ActionEvent;
/** * Project 4 main driver. * * This is the main class of an app that presents a simulation of the model * created by economist Thomas Schelling to help explain self-segregation. * * @author * @version 2017.04.20 */ public class SchellingSim extends Application { private SimGrid simGrid; private int round; private Text roundText; private Text satisfiedText; /** * Presents the simulation window with buttons to run and reset the * simulation. * * @param primaryStage the main application window */ public void start(Stage primaryStage) { Button stepButton = new Button("Step"); stepButton.setOnAction(this::handleStep); Button runButton = new Button("Run"); runButton.setOnAction(this::handleRun); Button resetButton = new Button("Reset"); resetButton.setOnAction(this::handleReset); FlowPane buttons = new FlowPane(stepButton, runButton, resetButton); buttons.setAlignment(Pos.TOP_CENTER); buttons.setPadding(new Insets(20, 0, 0, 0)); buttons.setHgap(20); simGrid = new SimGrid(30, 30); round = 0; roundText = new Text(); satisfiedText = new Text(); updateRoundData(); FlowPane roundData = new FlowPane(roundText, satisfiedText); roundData.setAlignment(Pos.TOP_CENTER); roundData.setHgap(20); VBox root = new VBox(buttons, roundData, simGrid); root.setSpacing(20); Scene scene = new Scene(root, 550, 600);
primaryStage.setTitle("Schelling Self-Segregation Simulator"); primaryStage.setScene(scene); primaryStage.show();
// Include the following line to avoid a bug in BlueJ primaryStage.setOnCloseRequest(e -> System.exit(0)); } /** * Handles the user pressing the Step button by performing one step of * the simulation and updating the display. * @param event the event representing the button being pushed */ public void handleStep(ActionEvent event) { if (simGrid.getSatisfiedPercent()
/** * Handles the user pressing the Run button by repeatedly performing steps * of the simulation until all agents are satisfied. * @param event the event representing the button being pushed */ public void handleRun(ActionEvent event) { while (simGrid.getSatisfiedPercent()
Link 2
import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import java.util.ArrayList; import java.util.Random;
/** * Part of the solution to Project 4. * * Represents the grid of agents in the Schelling Simulation. * * @author * @version 2017.04.20 */ public class SimGrid extends Pane { private Agent[][] grid; private Random gen; private int numCells; private double satisfiedPercent; // satisfaction threshold of 30% private final static double THRESHOLD = 0.3;
/** * Creates and initializes an agent grid of the specified size. * * @param rows the number of rows in the simulation grid * @param cols the number of cols in the simulation grid */ public SimGrid(int rows, int cols) { gen = new Random(); numCells = rows * cols; grid = new Agent[rows][cols]; initializeGrid(); // set initial satisfaction percentage ArrayList
/** * Fills the grid with an initial, random set of agents and vacant spaces. * Approximately 10% of the grid locations are left vacant. The rest are * evenly distributed between red and blue agents. */ public void initializeGrid() { // TO DO: Create an Agent for every cell in the grid. Each agent // will be either white, red, or blue. Give a 10% chance of it being // a white (vacant) cell. If it's not going to be vacant, give a // 50/50 chance that it will be red or blue. Also, add the square // for each agent to the pane (this object) so that it will be // displayed. } /** * Gets the current percentage of satisfied agents in the grid. * * @return the percentage of satisfied agents in the simulation */ public double getSatisfiedPercent() { return satisfiedPercent; } /** * Performs one step of the simulation by finding the location of all * unsatisfied agents, then moving each one to a randomly chosen vacant * location. * * @return the number of unsatisfied agents found */ public int performSimulationStep() { ArrayList
// TO DO: For each unsatisfied agent location, find a vacant // location and switch the colors of the agents. That is, make // the vacant agent blue or red as appropriate and make the // unsatisfied agent white (don't bother actually moving the Agent // objects). // update satisfaction percentage satisfiedPercent = (numCells - unsatisfied.size()) / (double) numCells * 100; return unsatisfied.size(); }
/** * Creates a list of all grid locations that contain an unsatisfied agent. * * @return a list of the locations of all currently unsatisfied agents */ private ArrayList
// TO DO: Examine each agent in the grid and, if it is not vacant and // if it is not satisfied, add that agent's grid location to the // unsatisfied list. return unsatisfied; } /** * Determines if the agent at the specified location is satisfied. First * gets a list of all valid, non-vacant neighbors, then counts the number * of those neighbors that are the same type. An agent is satisfied with * its current location if the ratio of similar agents is greater that * a set threshold. * * @return true if the agent is satisfied with its current location */ private boolean agentIsSatisfied(int i, int j) { ArrayList
// TO DO: Count how many of the neighbors have the same color // as the agent in question. return (double) sameCount / neighbors.size() > THRESHOLD; } /** * Gets a list of agents that are neighbors (adjacent) to the specified * grid location. Checks each potential location individually, making sure * that each is valid (on the grid) and not vacant. * * @return a list of agents that are adjacent to the specified location */ private ArrayList
// TO DO: Check all of the other potential neighbors, one at a time.
return neighbors; } /** * Determines if the specified grid location is valid. * * @return true if the specified location is a valid grid cell */ private boolean validLocation(int i, int j) { // TO DO: Determine if the values specified for i and j are // valid locations in the grid. } /** * Finds a vacant location in the simulation grid. Keeps checking cell * locations at random until a vacant one is found. * * @return the grid location of a vacant cell */ private GridLocation findVacantLocation() { // TO DO: Randomly pick a valid row and column and keep picking // until a vacant one is found and then return it as a GridLocation // object. } /** * Resets the simulation grid by clearing the pane of agent squares and * reinitializing the grid. */ public void resetGrid() { getChildren().clear(); initializeGrid(); // set initial satisfaction percentage ArrayList
Link 3
import javafx.scene.shape.Rectangle; import javafx.scene.paint.Color;
/** * Part of the solution to Project 4. * * Represents a simulation agent using a square of a particular color. * A white agent represents an unoccupied grid cell. * * @author * @version 2017.04.20 */ public class Agent { private Rectangle square;
/** * Sets the agent's display location (based on the specified row and * column) as well as the agent's color. * * @param row the grid row for this agent * @param col the grid column for this agent * @param color the color of this agent */ public Agent(int row, int col, Color color) { square = new Rectangle(col * 15 + 50, row * 15, 15, 15); square.setStroke(Color.BLACK); square.setFill(color); }
/** * Gets the rectangle (square) representing this agent. * @return the square representing this agent */ public Rectangle getSquare() { return square; } /** * Gets the color of this agent. * @return the color of this agent */ public Color getColor() { return (Color)square.getFill(); } /** * Sets the color of this agent. * * @param color the new color for this agent */ public void setColor(Color color) { square.setFill(color); } /** * Determines if this agent represents a vacant (white) location. * @return true if location is vacant and false otherwise */ public boolean isVacant() { return square.getFill().equals(Color.WHITE); } }
Link 4
/** * Part of the solution to Project 4. * * Represents one 2-dimensional grid location. * * @author * @version 2017.04.20 */ public class GridLocation { private int row; private int col;
/** * Sets the grid location. * * @param row the row of this grid location * @param col the column of this grid location */ public GridLocation(int row, int col) { this.row = row; this.col = col; }
/** * Gets the row of this location in the grid. * * @return the row value */ public int getRow() { return row; }
/** * Gets the column of this location in the grid. * * @return the column value */ public int getCol() { return col; } }
Problem Overview Racial segregation has always been a pernicious social problem in the United States. Although efforts have been made to desegregate our schools, churches, and neighborhoods, the U.S. continues to be segregated along racial and economic lines. n 1971, American economist Thomas Schelling created an agent-based model that might help explain why segregation is so difficult to combat. His model of segregation showed that even when individuals or "agents") didn't mind being surrounded or living by agents of a different race, they would still choose to segregate themselves from other agents over time! Although the model is quite simple, it gives a fascinating look at how individuals might self-segregate, even when they have no explicit desire to do SO In this assignment, you will create a simulation of Schelling's model. More precisely, you will be given the classes that make up the solution and asked to fill in missing details to make the solution work. How the Model Works Suppose there are two types of agents: X and O. The two types of agents might represent different races, ethnicity, economic status, etc. Two populations of the two agent types are initially placed into random locations of a neighborhood represented by a grid. After placing all the agents in the grid, each cell is either occupied by an agent or is vacant (empty) as shown here: X X O X O O O O O Agents placed Problem Overview Racial segregation has always been a pernicious social problem in the United States. Although efforts have been made to desegregate our schools, churches, and neighborhoods, the U.S. continues to be segregated along racial and economic lines. n 1971, American economist Thomas Schelling created an agent-based model that might help explain why segregation is so difficult to combat. His model of segregation showed that even when individuals or "agents") didn't mind being surrounded or living by agents of a different race, they would still choose to segregate themselves from other agents over time! Although the model is quite simple, it gives a fascinating look at how individuals might self-segregate, even when they have no explicit desire to do SO In this assignment, you will create a simulation of Schelling's model. More precisely, you will be given the classes that make up the solution and asked to fill in missing details to make the solution work. How the Model Works Suppose there are two types of agents: X and O. The two types of agents might represent different races, ethnicity, economic status, etc. Two populations of the two agent types are initially placed into random locations of a neighborhood represented by a grid. After placing all the agents in the grid, each cell is either occupied by an agent or is vacant (empty) as shown here: X X O X O O O O O Agents placedStep by Step Solution
There are 3 Steps involved in it
Step: 1
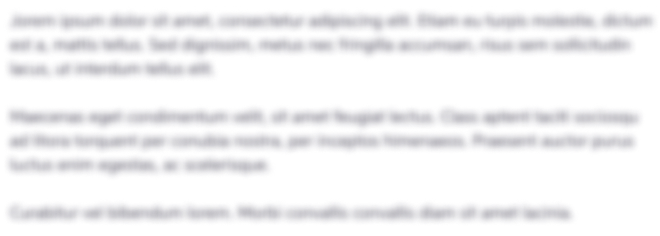
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started