Question
Locate the PHP code for validating the user's login information. Use the variables defined there to restrict the content page to only those who have
Locate the PHP code for validating the user's login information. Use the variables defined there to restrict the content page to only those who have successfully logged into the system.
Describe how the session is managed from page to page in the application. How long is the session active by default? What conditions will end the session?
Identify the form validation rules that need to be put in place for the login form and the registration form. What format should the data have? What is the maximum length of each item? Identify the necessary functions in JavaScript and PHP to create these validation rules and list them in your report.
// Variable to store errors as script processes
$err = '';
// Database Access Information
$hostname="db.petsales.com";
$username="petsales";
$password="password";
$dbname="petsales";
$con = mysql_connect($hostname,$username, $password) or die ("");
mysql_select_db($dbname);
// Navigation Management Variables
$def = true;
$mynav = '';
$mycontent = '';
$mylogin = false;
// Administrative Variables
$adm_pg = '';
$adm_un = 'admin'; // Username for administrator
$adm_pw = 'password'; // Password for administrator
// Check session information from form - email, session, fname lookup -
// Returns user type ('U' is default for active user, '0' means unregistered)
$mytype = '0';
$exp = false;
// This tests to see if the user is logged into the system successfully
// If so, $mylogin is set to TRUE
// If not, an error is generated into $err
if ((time() < intval($_POST['session']))) {
if ((($_POST['fname'] != '')&&($_POST['email'] != ''))&&($_POST['session'] != '')) {
$result = mysql_query("SELECT email,pw,fname,id FROM sessions WHERE email = '" . $_POST['email'] . "'"); // Email address is used directly.
$row = mysql_fetch_array($result);
if (!$result) {
$err = $err . "Your session is no longer valid. Please login again. ";
} else if ($row[0] == $_POST['email']) {
$email = $row[0];
$pw = $row[1];
$fname = $row[2];
$sess = time() + (60*60);
$exp = true;
// if successful, reset session expiration
$result = mysql_query("UPDATE sessions SET id = '" . $sess . "' WHERE email = '" . $email . "' AND pw = '" . $pw . "'");
$mylogin = true;
} else {
$err .= "Data did not return correctly. Please try your request again. ";
}
}
}
// This script processes the main request of the system
// Options are: register, login, logout
switch ($_POST['tokenid']) {
case "logout": // Logout from system
//kill session parameter in form
$_POST['session'] = '-1';
$_POST['email'] = '';
$_POST['fname'] = '';
$email = '';
$fname = '';
$sess = -1;
$email = '';
$mytype = '0';
$mylogin = false;
$exp = false;
break;
case "login": // Login to system
// verify login
// perform tests on values used
if (($_POST['us'] == $adm_un)&&($_POST['pw'] == $adm_pw)) { // Display Admin Results
//admin verification
$email = 'Admin';
$pw = $_POST['pw'];
$fname = $adm_un;
$mytype = 'A';
$sess = time() + (10*60);
$def = false;
$adm_pg = '';
$adm_result = mysql_query("SELECT email,fname,lname,pw FROM sessions");
if (!$adm_result) {
$err = $err . "The results from the database could not be returned. ";
$def = true;
} else {
$adm_i = 0;
$adm_j = 0;
$adm_pg = $adm_pg . '
Name | Password | |
' . $adm_row[0] . ' | ' . $adm_row[1] . ' ' . $adm_row[2] . ' | ' . $adm_row[3] . ' |
}
} else { // Test for regular login
$result = mysql_query("SELECT email,pw,fname,id FROM sessions WHERE email = '" . ($_POST['us']) . "' AND pw = '" . $_POST['pw'] . "'");
if (!$result) {
// false return; do not change default
$err = $err . "Login information did not match existing records. ";
} else {
$row = mysql_fetch_array($result);
if (($row[0] == $_POST['us'])&&($row[1] == $_POST['pw'])) { // Password is used directly with no modification
$email = $row[0];
$fname = $row[2];
$sess = $row[3];
$exp = true;
$err = $err . "Login successful! Welcome, " . $fname . "!";
//update session time
$sess = time() + (60*60);
$result = mysql_query("UPDATE sessions SET id = '" . $sess . "' WHERE email = '" . $_POST['email'] . "' AND pw = '" . $_POST['pw'] . "'");
$mylogin = true;
} else {
$err = $err . "Login information did not match existing records. ";
}
}
}
break;
case "register": // Register new user
$err_ct = 0;
// validate form data
//test for empties
//make sure passwords match
//test length of variables
//test for existing email registration
// perform registration
if ($err_ct == 0) {
$result = mysql_query("INSERT INTO sessions (email, pw, fname, lname, id) VALUES ('" . $_POST['email'] . "', '" . $_POST['pw'] ."','" . $_POST['fname'] . "','" . $_POST['lname'] . "','" . (time()+(60*60)) . "'");
}
if (!$result) {
$err = $err . "No data has been stored.";
} else {
$result = mysql_query("SELECT email,pw,fname,id FROM sessions WHERE email = '" . $_POST['email'] . "' AND pw = '" . $_POST['pw'] . "'");
$row = mysql_fetch_array($result);
if (!$result) {
// false return; do not change default
$err = $err . "Data storage unsuccessful. Please try again. ";
} else {
if (($row[0] == $_POST['email'])&&($row[1] == hash('md5',$_POST['pw']))) {
$email = $row[0];
$fname = $row[2];
$sess = $row[3];
$exp = true;
$err = $err . "Registration successful! Welcome, " . $fname . "! ";
$mylogin = true;
} else {
$err = $err . "Data storage unsuccessful. Please try again. ";
}
}
}
break;
// The following cases are for navigation only - Do not edit!
case "reg": // Register new user
$def = false;
echo 'document.getElementById("thispage").innerHTML = displayfile("' . $_POST['pagereq'] . '");' . PHP_EOL;
break;
case "navigate":
// select navigation parameters
if ($mylogin) {
echo 'document.getElementById("thispage").innerHTML = displayfile("' . $_POST['pagereq'] . '");' . PHP_EOL;
} else {
echo 'document.getElementById("thispage").innerHTML = "You do not have the correct permissions to view this content.";' . PHP_EOL;
}
$def = false;
break;
case "request":
// request restricted item
// return or deny
if ($mylogin) {
echo 'document.getElementById("thispage").innerHTML = displayfile("./' . $_POST['pagereq'] . '");' . PHP_EOL;
} else {
echo 'document.getElementById("thispage").innerHTML = "You do not have the correct permissions to view this content.";' . PHP_EOL;
}
$def = false;
break;
case "js":
if ($mylogin) {
echo $_POST['pagereq'] . PHP_EOL;
} else {
echo 'document.getElementById("thispage").innerHTML = "You do not have the correct permissions to view this content.";' . PHP_EOL;
}
$def = false;
break;
default:
$def = true;
break;
}
//session type sets navigation options - Do not edit!
$mynav = "'";
if ($mylogin) { // Test for login before displaying option
$mynav .= "View Pet Inventory";
}
$mynav .= "';";
if ($exp) {
if (($fname == '')&&($_POST['fname'] != '')) {
$fname = $_POST['fname'];
}
echo 'this.document.getElementById("mylogin").innerHTML = "Welcome, ' . $fname . '! (Logout) Change Password";'. PHP_EOL;;
}
if ($def) {
if ($err == '') {
echo 'document.getElementById("thispage").innerHTML = displayfile("include/desc.html");'. PHP_EOL;
} else {
echo 'document.getElementById("thispage").innerHTML = " Message: ' . $err . ' " + displayfile("include/desc.html");'. PHP_EOL;
}
} else if ($mycontent != '') {
echo "document.getElementById(\"thispage\").innerHTML = '" . $mycontent . "';" . PHP_EOL;
}
if ($adm_pg <> '') {
echo "document.getElementById(\"thispage\").innerHTML = '" . $adm_pg . "';" . PHP_EOL;
}
echo 'document.getElementById("thisnav").innerHTML = ' . $mynav . PHP_EOL;
?>
Step by Step Solution
There are 3 Steps involved in it
Step: 1
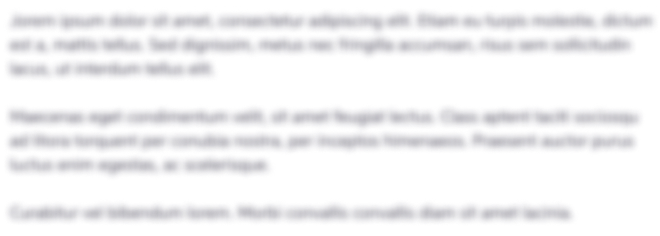
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started