Question
(LOCATION) public class Location { public static final Location JMU = new Location(38.435427, - 78.872942); public static final Location ISAT = new Location(38.434663, - 78.863732);
(LOCATION)
public class Location
{
public static final Location JMU = new Location(38.435427, -
78.872942);
public static final Location ISAT = new Location(38.434663, -
78.863732);
private double latitude;
private double longitude;
/**
* Explicit value constructor for the this Location.
*
* @param latitude The latitude in degrees
* @param longitude The longitude in degrees
*/
public Location(double latitude, double longitude)
{
this.latitude = latitude;
this.longitude = longitude;
}
/**
* Are the two values within .000001 of each other?
*
* @return true if the two values are the same in
* the two objects and false otherwise
*/
public boolean equals(Location other)
{
return (this.latitude - other.latitude
&& this.longitude - other.longitude
}
/**
* Returns the latitude and longitude for this Location.
*
* @return string representation of this Location
*/
public String toString()
{
return String.format("%.6f/%.6f", latitude, longitude);
}
}
(ALIEN)
public class Alien {
private static int alienCount;
private static int rogueCount;
private String name; // original name
private String homePlanet; // the home planet
private Location location; // where the alien is currently located
private String alias; // name that they go by on earth
private boolean wanted; // if the MIB agency is looking for them
/**
* Default constructor - We know we have an alien but don't know much
* about it. This method simply calls the explicit value constructor.
*/
public Alien() {
this("Unknown", "Unknown", Location.ISAT, "Unknown");
}
/**
* Explicit value constructor - Sets each of the attributes based on
the
* parameters and sets wanted to false. This method should increment
the
* alien count.
*
* @param name this alien's original name
* @param homePlanet the origination of this alien
* @param loc the current location for this alien
* @param alias the name this alien goes by on Earth
*/
public Alien(String name, String homePlanet, Location loc, String
alias) {
}
/**
* Indicates that an alien that has been captured and placed at
* the JMU detention facility.
* If the alien was a wanted criminal, then reset wanted to false and
* decrement the rogueCount. In either case, this alien should be
placed
* at the JMU location.
*/
public void capturedJMU() {
}
/**
* Indicates an alien has been deported. It is possible to deport an
* alien without a prior capture. If this alien is wanted, reset the
* the wanted attribute to false and decrement the rogueCount. In
either
* case, set the location to null and decrement the alienCount.
*/
public void deport() {
}
/**
* Identifies that an alien has violated the rules of alien
immigration.
* If this is a new identification (wanted is false), set wanted to
true
* and increment the rogue count. If this alien is already wanted,
then
* do neither.
*/
public void goneRogue() {
}
/**
* Places this alien into a new location.
*
* @param newLoc new current location
*/
public void move(Location newLoc) {
}
/**
* Returns the current location of this alien.
*
* @return current location
*/
public Location getCurrentLocation() {
}
/**
* Returns the total number of aliens on the planet.
*
* @return total number of aliens (including those wanted)
*/
public static int getNumberAliens() {
}
/**
* Returns the total number of wanted aliens on the planet.
*
* @return number of wanted aliens
*/
public static int getNumberWanted() {
}
/**
* Returns basic information about this alien.
*
* @return a String of the form: "%s aka %s from %s currently at %s"
* followed by the term "ROGUE" or the empty String,
* depending on whether isWanted is true or false.
* If location is null, output "Off Planet" in place of the location.
*/
public String toString() {
String location;
String rogue;
if (this.location != null) {
location = this.location.toString();
}
else {
location = "Off Planet";
}
if (wanted) {
rogue = "ROGUE";
}
else {
rogue = "";
}
return String.format("%s aka %s from %s is currently at %s %s",
this.name, this.alias, this.homePlanet,
location, rogue);
}
}
Location.java 1. Is the Location class mutable or immutable? Why? 2. Why is [mutable/immutable] the appropriate design for this class? 3. Why do we have only one constructor? Would there be any use for another? 4. What is the purpose of the two Location constants JMU and ISAT? 5. Is there any danger in making the constants public? Why or why not? Alien.java 6. Why is it important that all the attributes of the Alien class are 7. What is the purpose of the class variables? Why are they private and 8. What would happen if they were not static? (i.e., when you create private? static? objects) 9. Which of the methods in Alien.java are mutator methods? 10. Which of the methods in Alien.java are accessor methods
Step by Step Solution
There are 3 Steps involved in it
Step: 1
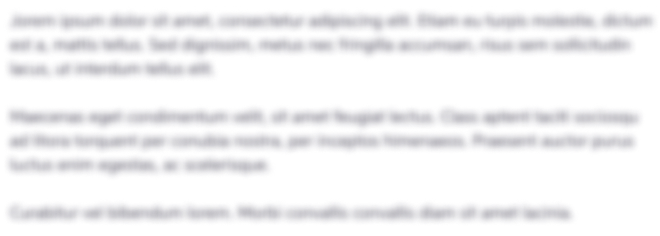
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started