Question
Look at the 2 examples of Pseudocode supplied with this assignment Choose as a program manager which pseudocode you would select to go ahead with
Look at the 2 examples of Pseudocode supplied with this assignment
Choose as a program manager which pseudocode you would select to go ahead with as a project on for an entire group. So which pseudocode was clearest to a person who might not be a full time programmer or who might not know much about C++.
Justify your selection with one or two additional sentences explaining why you selected the one that you did and why you selected that one.
Pseudocode Example 1:
Global variables:
int BurgerPatties - total number of hamburger patties in inventory
int BurgerBuns - total number of hamburger buns
int Hotdogs - total number of hot dogs
int HotdogBuns - total number of hot dog buns
int Chili - total ounces of chili
int FryBaskets - total number of fry baskets in inventory
int Soda - total cans of soda
double CashRegister - total amount of money in register
SellHamburger Function - two arguments (chili, true or false, quantity default is 1):
Sells hamburgers, returns a double that is the total amount of the transaction
double Total - total amount due from customer
double Tax - added sales tax
If chili is true
Assign Total (7.00 times quantity)
Assign Tax (5 divided by 100) times Total
Add Total plus Tax to CashRegister
Decrement Chili by (4 times quantity)
Else
Assign Total (5.00 times quantity)
Assign Tax (5 divided by 100) times Total
Add Total plus Tax to CashRegister
End
Decrement BurgerPatties by quantity
Decrement BurgerBuns by quantity
Return Total plus Tax
End function
SellHotdog Function - two arguments (chili, true or false, quantity default is 1):
Sells hot dog. returns a double
double Total - total amount due
double Tax - added sales tax
If chili is true
Assign Total (7.00 times quantity)
Assign Tax (5 divided by 100) times Total
Add Total plus Tax to CashRegister
Decrement Chili by (4 times quantity)
Else
Assign Total (5.00 times quantity)
Assign Tax (5 divided by 100) times Total
Add Total plus Tax to CashRegister
End
Decrement Hotdogs
Decrement HotdogBuns
Return Total plus Tax
End function
ShowMenu Function
Prints the interactive menu based on inventory
Print the following:
Welcome to Billys Burgs and Furts
Type one of the following characters to do something
q - Quits the program
h - Shows this menu
m - Shows this menu
If BurgerBuns is greater than 0:
Print: b - Hamburger(s) - $5.00 each
If Chili is greater than 4:
Print: e - Chili hamburger(s) - $7.00 each
End
End
If HotdogBuns is greater than 0:
Print: d - Hotdog(s) - $5.00
If Chili is greater than 4:
Print: g - Chili hotdog(s) - $7.00
End
End
If Chili is greater than 12:
Print: c - 12 oz container of chili - $4.00
End
If FryBaskets is greater than 0:
Print: f - Basket of fries - $7.00
End
If Soda is greater than 0:
Print: s - Can of Soda - $2.00
End
End function
Main function:
Assign BurgerPatties 200
Assign BurgerBuns 75
Assign Hotdogs 200
Assign HotdogBuns 75
Assign Chili 500
Assign FryBaskets 75
Assign Soda 200
Assign CashRegister 0.0
char MenuOption - the letter the user types at the menu
int Quantity - number of the specified item to sell
double SubTotal - total amount for this transaction
double Tax - added sales tax
Call ShowMenu
Do:
If BurgerBuns is less than or equal to 15:
Print Running low on hamburgers, only then BurgerBuns then left
End
If HotdogBuns is less than or equal to 15:
Print Running low on hotdogs, only then HotdogBuns then left
End
If Chili is less than or equal to 100:
Print Running low on chili, only then Chili then ounces left
End
If FryBaskets is less than or equal to 15:
Print Running low on fry baskets, only then FryBaskets then left
End
If Soda is less than or equal to 40:
Print Running low on soda, only then Soda then cans left
End
Get string from stdin and store first character in MenuOption
(User presses enter)
If MenuOption is equal to b: (Hamburgers)
If BurgerBuns is equal to 0:
Print Sorry no more burgers
Else:
Print How many hamburgers?
Get integer from stdin and store in Quantity
(User presses enter)
If (BurgerBuns minus Quantity) is greater than or equal to 0:
Call SellHamburger with parameters false and Quantity,
and store result in SubTotal
Print $ then SubTotal
Else:
Print We dont have that many hamburgers
End
End
End
If MenuOption is equal to e: (Chili burgers)
If BurgerBuns is equal to 0:
Print Sorry no more burgers
Else if Chili is less than 4:
Print Sorry not enough chili
Else:
Print How many chili burgers?
Get integer from stdin and store in Quantity
(User presses enter)
If (BurgerBuns minus Quantity) is greater than or equal to 0:
Call SellHamburger with parameters true and Quantity,
and store result in SubTotal
Print $ then SubTotal
Else:
Print We dont have that many hamburgers
End
End
End
If MenuOption is equal to d: (Hotdogs)
If HotdogBuns is equal to 0:
Print Sorry no more hotdogs
Else:
Print How many hot dogs?
Get integer from stdin and store in Quantity
(User presses enter)
If (HotdogBuns minus Quantity) is greater than or equal to 0:
Call SellHotdog with parameters false and Quantity, and
store result in SubTotal
Print $ then SubTotal
Else:
Print We dont have that many hot dogs
End
End
End
If MenuOption is equal to g: (Chili dogs)
If HotdogBuns is equal to 0:
Print Sorry no more hotdogs
Else if Chili is less than 4:
Print Sorry not enough chili
Else:
Print How many chili dogs?
Get integer from stdin and store in Quantity
(User presses enter)
If (HotdogBuns minus Quantity) is greater than or equal to 0:
Call SellHotdog with parameters true and Quantity, and
store result in SubTotal
Print $ then SubTotal
Else:
Print We dont have that many hot dogs
End
End
End
If MenuOption is equal to c: (12 oz of Chili)
Print How many 12-ounce containers of chili?
Get integer from stdin and store in Quantity
(User presses enter)
If (Chili minus Quantity) is greater than or equal to 0:
Decrement Chili by (12 times Quantity)
Assign SubTotal (4.00 times Quantity)
Assign Tax (5 divided by 100) times SubTotal
Add SubTotal plus Tax to CashRegister
Print $ then (SubTotal plus Tax)
Else:
Print We dont have that much chili
End
End
If MenuOption is equal to f: (Basket of fries)
Print How many baskets of fries?
Get integer from stdin and store in Quantity
(User presses enter)
If (FryBaskets minus Quantity) is greater than or equal to 0:
Decrement FryBaskets by Quantity
Assign SubTotal (7.00 times Quantity)
Assign Tax (5 divided by 100) times SubTotal
Add SubTotal plus Tax to CashRegister
Print $ then (SubTotal plus Tax)
Else:
Print We dont have that many fry baskets
End
End
If MenuOption is equal to s: (Cans of soda)
Print How many cans of soda?
Get integer from stdin and store in Quantity
(User presses enter)
If (Soda minus Quantity) is greater than or equal to 0:
Decrement Soda by Quantity
Assign SubTotal (2.00 times Quantity)
Assign Tax (5 divided by 100) times SubTotal
Add SubTotal plus Tax to CashRegister
Print $ then (SubTotal plus Tax)
Else:
Print We dont have that much soda
End
End
If MenuOption is equal to h or MenuOption is equal to m: (Show menu)
Call ShowMenu
End
While MenuOption is not equal to q (quit)
Print Wow we made a lot of money! $ then CashRegister
Print We sold then (75 minus BurgerBuns) then hamburgers!
Print We sold then (75 minus HotdogBuns) then hot dogs!
Print We sold then (75 minus FryBaskets) then fry baskets!
Print We sold then (500 minus Chili) then ounces of chili!
Print We sold then (200 minus Soda) then cans of soda!
Return 0
End function
My approach to this program is to use a large do-while loop, that records the users choice for
menu options. These menu options prompt the user again for the quantity of the selected item,
and tell the user is there is not enough of it in inventory. The total is calculated and added to the
cash register, and printed on the screen after the transaction. The loop checks the inventory for
each item, and warns the user if any of the items are getting low. At the end of the session, after
the user has entered q to quit, the amount of money that was made during the session is
printed on screen, with a detailed list of how many of each item was sold. I wrote three
functions: One for selling hamburgers, one for selling hotdogs, and one for printing the menu on
screen. Each of the inventory items has its own global variable.
Pseudocode Example 2:
Variables:
inventoryLeft (table of values containing inventory of each menu item)
menuItems (table containing strings of menu items)
itemOrdered
orderTotal
Constants:
itemPrices (table containing prices of menu items)
amtPerOrder (table containing amount of each item given every time its ordered)
twentyPercent (table containing values for twenty percent of initial inventory)
Program: RebelMenu
Display menuItems with corresponding numbers
Prompt user for the number corresponding to menu item ordered
Set itemOrdered to user entry
WHILE (itemOrdered does not equal -1)
IF (checkInventory() is true)
Subtract the amtPerOrder of the itemOrdered from the corresponding
inventoryLeft
IF (itemOrdered inventoryLeft is less than the corresponding twentyPercent)
Display Less than 20% inventory remains
END IF
Add the itemOrdereds price to orderTotal
Display orderTotal
END IF
ELSE
Display Not enough inventory, please order something else
END ELSE
Display menuItems with corresponding numbers
Prompt user for the number corresponding to menu item ordered (with -1 to quit and 10
to complete current customer order)
IF (itemOrdered equals 10)
Display orderTotal
Set orderTotal to 0
Display menuItems with corresponding numbers
Prompt user for the number corresponding to menu item ordered (with -1 to quit)
END IF
END WHILE
Function: checkInventory()
IF (itemOrdered inventoryLeft is less than corresponding amtPerOrder)
Replace corresponding menuItem with Sold Out
Return false
END IF
ELSE IF (itemOrdered inventoryLeft is equal to corresponding amtPerOrder)
Replace corresponding menuItem with Sold Out
Return true
END ELSE IF
ELSE
Return true
END ELSE
My approach is associating each menu item with a number, and using several vectors/arrays with
different attributes about the menu items to alter the inventory, get the order total, etc. All the
employee running the system would have to do is enter a number when the customer orders
something, making the program easy to use. When they want to finish an order, they enter 10,
and the order total is calculated. To close out at the end of the night, they enter -1. I use an
additional function to check if the inventory is less than necessary to fulfill the order, as well as
change the menu item to Sold out so next time the employee knows its sold out. Because the
chili is sold in different amounts, you cant guarantee anything more than 0 in inventory means
the order can be fulfilled.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
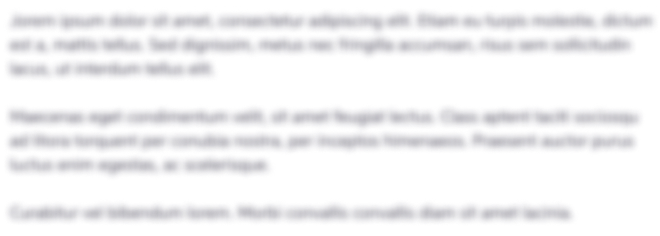
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started