Question
main.cpp file #include SimpleVectorOfInts.h #include #include #include using namespace std; int main() { // test default constructor SimpleVectorOfInts emptyVector; // NOTE: These asserts will fail
main.cpp file
#include "SimpleVectorOfInts.h"
#include
#include
#include
using namespace std;
int main()
{
// test default constructor
SimpleVectorOfInts emptyVector;
// NOTE: These asserts will fail until the default constructor is working properly.
assert(emptyVector.size() == 0);
assert(emptyVector.capacity() == 10);
/*
NOTE: You can put whatever testing code you want in this file. This file will not
be used as a part of the evaluation. This assignment is evalutated by unit-tesing
the SimpleVectorOfInts interface and implmentation.
*/
cout
return 0;
}
SimpleVectorOfInts.cpp file
#include "SimpleVectorOfInts.h"
//***********************************************************
// Default Constructor for SimpleVectorOfInts class. Sets *
// the default size and capacity for the vector and *
// allocates memory for the the internal array. *
//***********************************************************
SimpleVectorOfInts::SimpleVectorOfInts()
{
// put your code here
}
//***********************************************************
// Constructor for SimpleVectorOfInts class. Sets the size *
// of the array and allocates memory for it. *
//***********************************************************
SimpleVectorOfInts::SimpleVectorOfInts(int s)
{
// put your code here
}
//*************************************************
// Copy Constructor for SimpleVectorOfInts class. *
//*************************************************
SimpleVectorOfInts::SimpleVectorOfInts(const SimpleVectorOfInts &obj)
{
// put your code here
}
//********************************************
// Destructor for SimpleVectorOfInts class. *
//********************************************
SimpleVectorOfInts::~SimpleVectorOfInts()
{
// put your code here
}
//*******************************************************
// memError function. Displays an error message and *
// terminates the program when memory allocation fails. *
//*******************************************************
void SimpleVectorOfInts::memError()
{
cout
exit(EXIT_FAILURE);
}
//***********************************************************
// subError function. Displays an error message and *
// terminates the program when a subscript is out of range. *
//***********************************************************
void SimpleVectorOfInts::subError()
{
cout
}
//*******************************************************
// getElementAt function. The argument is a subscript. *
// This function returns the value stored at the sub- *
// cript in the array. *
//*******************************************************
int SimpleVectorOfInts::getElementAt(int sub)
{
// put your code here
return dynamicArrayPtr[sub];
}
//*******************************************************
// Overloaded [] operator. The argument is a subscript. *
// This function returns a reference to the element *
// in the array indexed by the subscript. *
//*******************************************************
int &SimpleVectorOfInts::operator[](const int &sub)
{
return dynamicArrayPtr[sub];
}
//***********************************************************
// The push_back function pushes its argument onto the back *
// of the vector. *
//***********************************************************
void SimpleVectorOfInts::push_back(int val)
{
// put your code here
}
//***********************************************************
// The pop_back function removes the last element *
// of the vector. It also returns that value. *
//***********************************************************
int SimpleVectorOfInts::pop_back()
{
// put your code here & fix return
return 0;
}
SimpleVectorOfInts.h file
#ifndef SimpleVectorOfInts_SimpleVectorOfInts_h
#define SimpleVectorOfInts_SimpleVectorOfInts_h
#include
#include
using namespace std;
class SimpleVectorOfInts
{
private:
int *dynamicArrayPtr; // To point to the allocated array
int numberOfElements; // Number of elements in the array
int arrayCapacity; // capacity of the dynamic array
void memError(); // Handles memory allocation errors
void subError(); // Handles subscripts out of range
public:
// Default constructor
SimpleVectorOfInts();
// Constructor declaration
SimpleVectorOfInts(int);
// Copy constructor declaration
SimpleVectorOfInts(const SimpleVectorOfInts &);
// Destructor declaration
~SimpleVectorOfInts();
// Accessor to return the array size
int size() const
{ return numberOfElements; }
// Accesssor to return the capacity of the vector
int capacity() const
{ return arrayCapacity; }
// Accessor to return a specific element
int getElementAt(int position);
// Overloaded [] operator declaration
int &operator[](const int &);
void push_back(int); // New push_back member
int pop_back(); // New pop_back member
};
#endif
Please complete the codes above in C++. thank you
13.21 CIST2362 Programming Assignment: Exploring the Vector Class Overview This programming assignment explores how the vector class works. The vector class you were introduced to behaves like an array. You used features like pushback0, popback0, size(), etc. In this programming assignment you will work with simplified version of the vector class that only holds integers. This class is named SimpleVectorOfints. Your first task is to read and understand the SimpleVectorOfInts.h file. This is the interface for the class i.e. the contract with external software on the promised behavior. Then your main task is to implement each of the functions in the SimpleVectorOfInts.cpp file. As you implement each of the functions, you should test your functions by writing code in main. For example, once you finish the pushback0 function, then you should test your pushback0 in various scenarios in your main such as pushback0 on an empty vector, pushback on a full vector, etc. The code in the main.cpp will not be uploaded for testing. The only code you will upload for testing is the SimpleVectorOfInts.h and SimpleVectorOfInts.cpp files. These two files will be evaluated using what are known as unit tests. A unit test is a test designed to check and verify a single piece of functionality. While all the tests are run at the same time, you should upload your files as you complete functions. The test will then let you know that you have passed testing for those functions. You will fail tests for unimplemented functions, but at least you can see the progress as you go. Note that you will be able to see the majority of the tests, but there will be some that are hidden. The purpose of this is to have you thoroughly test your code and try to satisfy the unexpected. This will help to sharpen your testing and programming skills. Requirements This SimpleVectorOfInts class is expected to behave like the standard vector class. There are a few requirements that need to be met: 1. The default capacity of the vector should be 10 elements, and the default size should be 0 . 2. When a push_back 0 is performed, the vector is increased by 5 if the vector is already full. 3. The pop_back0 should change the size by 1, but should not affect the capacity. 4. The getElementAt0 should ensure the subscript is within range, however, the [ operator should notStep by Step Solution
There are 3 Steps involved in it
Step: 1
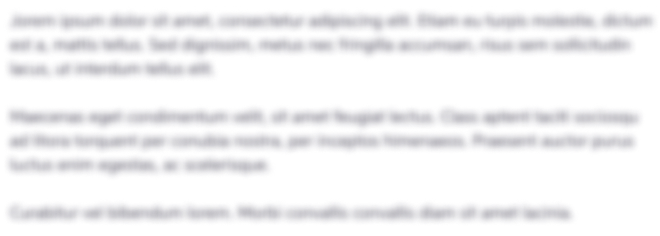
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started