Question
Mak a console interface for managing numeric data, consisting of two classes: NApp and NData, both contained in the NApp.java file. NApp is the user
Mak a console interface for managing numeric data, consisting of two classes: NApp and NData, both contained in the NApp.java file. NApp is the user interface and manages an array of NData objects. Submit your source code: the NApp.java file. The design requirements are:
1) The NData class should be placed after and outside the NApp class definition, as:
public class NApp { // code goes here} class NData { // more code goes here}
Note that only the NApp class can be public!
2) NData [object] data members:
a) a private String name
b) three private ints r, g, and b
3) NData [object] methods:
a) public methods getName and setName:
- The get method will return the NData object's existing name field.
- The set method will accept a String and, if it is neither null nor zero-length, assign it to the NData object's name field.
b) public methods getR/G/B and setR/G/B(six methods total):
- Each get method will return the NData object's r, g, or b field, as appropriate
- Each set method will accept an int and, if it is in the range 0 - 255 inclusive, assign it to the NData object's r, g, or b field, as appropriate.
d) a constructor method that receives a String and three ints as parameters and assigns them respectively to name, r, g, and b.
1) NApp [object] data fields:
a) an array named data of type NData without any initial size (i.e., declared but not created)
b) a Scanner scanner (import the file java.util.Scanner)
2) NApp methods:
a) public [object] method create: prompts the user to enter a String and three ints between 0 and 255, sends those inputs to NData's constructor, and returns the new NData object.
b) public [object] method add: takes an NData object as a parameter, and appends it to the data array - data will need to be expanded by one element to accommodate the new NData object.
c) public [object] method list: for every element in data, display element's index in data, then the NData object's nameand r/g/b fields. If data.length == 0, print the message "no records to display".
d) a constructor method that takes no parameters, creates data as an NData array with size 0, and creates scanner as a new Scanner object using the System.in input stream.
e) a main method that creates an NApp object. Then, starts an infinite while loop (i.e., while(true)) for that object to prompt the user and accept input via the console for the commands: create, add, and list. Each command should call the corresponding NApp method.
f) Additionally, the loop should accept an "exit" command that ends the program the method call System.exit(0);
Error Checking
The application should check for:
- Any input command that cannot be correctly interpreted cause the message "invalid command" to be displayed. The application should keep running as normal afterward - the loop should continue.
- Any invalid inputs for a new NData object will prevent its creation and should display an appropriate error message. The following cases should be handled.
- A null or zero-length String for name
- A non-numeric or non-integer value for any of r, g, or b
- A value outside the 0 - 255 range for any of r, g, or b
Coding Sequence
To avoid spurious errors in Eclipse (from accessing unwritten data & methods in NData): first write both class shells in the NApp.java file, then the data fields & methods in NData, and only then write the code for the data fields& methods in NApp.
Additional Constraints
The program should be implemented as a single source code file. Comments should be provided at the start of the class file (i.e., above the class definition) giving the class author's name and the date the program was finished.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
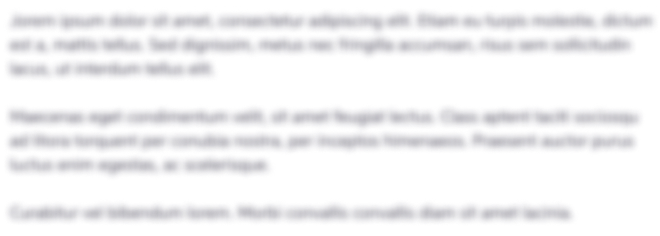
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started