Question
Make an indentation for this code import csv student_grades = [] # First open up your file containing the raw student grades with open(studentGradeFrom.csv, r)
Make an indentation for this code
import csv
student_grades = []
# First open up your file containing the raw student grades
with open("studentGradeFrom.csv", "r") as file:
# Setup your reader with whatever your settings actually are
csv_file = csv.DictReader(file, delimiter=",", quotechar='"')
# Cycle through each row of the csv file
for row in csv_file:
# Calculate the numerical grade of the student
grade = grader(
int(row["test1"]),
int(row["test2"]),
int(row["test3"]),
int(row["test4"])
)
# Calculate the letter score for the student
score = gradeScores(grade)
# Assemble all the data into a dictionary
# Only need to save fields you need in the final output
student_grades.append({
"first_name": row["first_name"],
"last_name": row["last_name"],
"test1": row["test1"],
"test2": row["test2"],
"test3": row["test3"],
"test4": row["test4"],
"grade": grade,
"score": score
})
# Open up a new csv file to save all the grades
with open("studentGradeFrom.csv", "w", newline="") as file:
# List of column names to use as a header for the file
# These will be used to match the dictionary keys set above
# Only need to list the fields you saved above
column_names = [
"first_name", "last_name", "test1", "test2", "test3",
"test4", "grade", "score"
]
# Create the csv writer, using the above headers
csv_file = csv.DictWriter(file, column_names)
# Write the header
csv_file.writeheader()
# Write each dictionary to the csv file
for student in student_grades:
csv_file.writerow(student)
You would need to fine tune this to your exact requirements, but it will hopefully get you going in the right direction. Most of this is documented in the official documentation if you need a specific reference: https://docs.python.org/3.6/library/csv.html.
import csv
student_grades = []
# First open up your file containing the raw student grades
with open("studentGradeFrom.csv", "r") as file:
# Setup your reader with whatever your settings actually are
csv_file = csv.DictReader(file, delimiter=",", quotechar='"')
# Cycle through each row of the csv file
for row in csv_file:
# Calculate the numerical grade of the student
grade = grader(
int(row["test1"]),
int(row["test2"]),
int(row["test3"]),
int(row["test4"])
)
# Calculate the letter score for the student
score = gradeScores(grade)
# Assemble all the data into a dictionary
# Only need to save fields you need in the final output
student_grades.append({
"first_name": row["first_name"],
"last_name": row["last_name"],
"test1": row["test1"],
"test2": row["test2"],
"test3": row["test3"],
"test4": row["test4"],
"grade": grade,
"score": score
})
# Open up a new csv file to save all the grades
with open("studentGradeFrom.csv", "w", newline="") as file:
# List of column names to use as a header for the file
# These will be used to match the dictionary keys set above
# Only need to list the fields you saved above
column_names = [
"first_name", "last_name", "test1", "test2", "test3",
"test4", "grade", "score"
]
# Create the csv writer, using the above headers
csv_file = csv.DictWriter(file, column_names)
# Write the header
csv_file.writeheader()
# Write each dictionary to the csv file
for student in student_grades:
csv_file.writerow(student)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
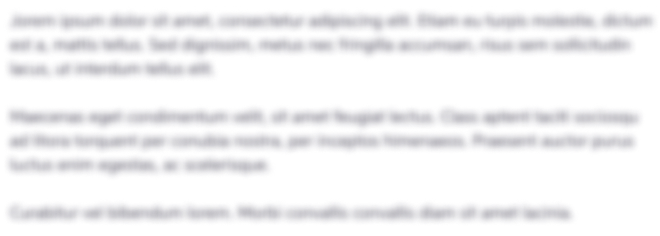
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started