Question
Make ArrayList of Player objects, let players take turns Having a single-player game is pretty boring. There is already a Player class that allows you
Make ArrayList of Player objects, let players take turns
Having a single-player game is pretty boring. There is already a Player class that allows you to create a player object, with a name and a score, etc. Your job in this part is to create an ArrayList of players, so that any number of people can join the game.
Create an ArrayList of Player objects.
In the setupGame method, before showing the rules, ask how many people are going to play. Use the scanners nextInt() method to get the number they enter.
Ask for each players name and use that to create a new Player object with that name and add that Player object to the ArrayList of players.
Now in the playGame() method, you need to change the code so that it cycles through each player, giving each a turn. If there are three players, each should get a turn, and then it should go back to the first player again. Youll need a variable in this method to keep track of which players turn it is. NOTE: this should be a simplified Wheel of Fortune game, where each player gets one spin and one letter guess, and then it is the next players turn. (i.e. a player doesnt get to keep on taking more turns if they guess a letter correctly).
Finish the code in the solvePuzzleAttempt() method that prints out the stats for each player at the end of the round (who won and how much each player earned).
Test your program with multiple players to make sure that it works properly
THE CODE IS PROVIDED FOR YOU BELOW, PLEASE INPUT ALL NEW CODE INTO THIS ONE. THANKS!!!
import java.util.Scanner; import java.util.ArrayList; import java.util.Random; import java.util.InputMismatchException;
/** * WofFortuneGame class * Contains all logistics to run the game * @author */ public class WofFortuneGame {
private boolean puzzleSolved = false;
private Wheel wheel; private Player players; private String phrase = "Once upon a time"; // private Letter[] letter_array = new Letter[16]; private static ArrayList
/** * Constructor * @param wheel Wheel * @throws InterruptedException */ public WofFortuneGame(Wheel wheel) throws InterruptedException { // get the wheel this.wheel = wheel; // do all the initialization for the game setUpGame();
} /** * Plays the game * @throws InterruptedException */ public void playGame() throws InterruptedException { // while the puzzle isn't solved, keep going while (!puzzleSolved){ int guess=0; for(Player turn:Players){ Players.get(guess); guess++; playTurn(turn); if(puzzleSolved){ break;//ends when phrase is guessed } } // let the current player play // playTurn(player1); } } /** * Sets up all necessary information to run the game */ private void setUpGame() { // create a single player // player1 = new Player("Player1"); addPhrase(); // Calling method to add more phrases to the game3 System.out.println("How many players?");//prompting number of players try{ Scanner playerCount = new Scanner(System.in); int player = playerCount.nextInt(); System.out.print(+player); System.out.println("Please enter players names");//prompting for names for(int i =0; i /** * One player's turn in the game * Spin wheel, pick a letter, choose to solve puzzle if letter found * @param player * @throws InterruptedException */ private void playTurn(Player player) throws InterruptedException { int money = 0; Scanner sc = new Scanner(System.in); System.out.println(player.getName() + ", you have $" + player.getWinnings()); System.out.println("Spin the wheel! System.out.println("Would you like to try to solve the puzzle? (Y/N)"); letter = sc.next().charAt(0); System.out.println(); if ((letter == 'Y') || (letter == 'y')) { solvePuzzleAttempt(player); } } } } /** * Logic for when user tries to solve the puzzle * @param player */ private void solvePuzzleAttempt(Player player) { if (player.getNumGuesses() >= 3) { System.out.println("Sorry, but you have used up all your guesses."); return; } player.incrementNumGuesses(); System.out.println("What is your solution?"); Scanner sc = new Scanner(System.in); sc.useDelimiter(" "); String guess = sc.next(); if (guess.compareToIgnoreCase(phrase) == 0) { System.out.println("Congratulations! You guessed it!"); puzzleSolved = true; // Round is over. Write message with final stats // TODO } else { System.out.println("Sorry, but that is not correct."); } } /** * Display the puzzle on the console */ private void showPuzzle() { System.out.print("\t\t"); for (Letter l : Letters) { if (l.isSpace()) { System.out.print(" "); } else { if (l.isFound()) { System.out.print(Character.toUpperCase(l.getLetter()) + " "); } else { System.out.print(" _ "); } } } System.out.println(); } /** * For a new game reset player's number of guesses to 0 */ public void reset() { players.reset(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
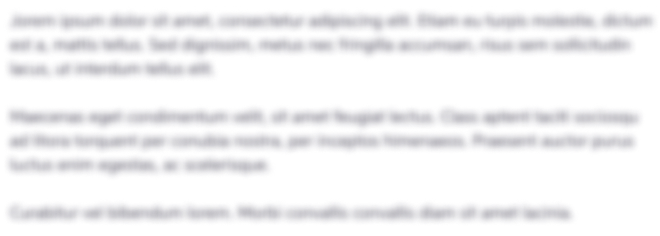
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started