Question
make Junit tests for my code but i'm a bit confused on how to do it. My code makes an empty singly linked list and
make Junit tests for my code but i'm a bit confused on how to do it. My code makes an empty singly linked list and you can add items to the head or tail. my code:
public class practice { // = a generic (this means the singly linked list can be created with any element i.e a string, int, etc)
//singly linked lists are composed of nodes
//implement a node
private static class Node{ //private because only the list should access the nodes
private T element; //element that only the node contains
//Linked list has a node which contains the element itself and then it points to the next element
private Node next;//pointer to the next node
public Node(T e, Node n) { //creating a node with its own element and a pointer (Node) to another type of node (n)
element = e;
next = n;
}
//we want to retrieve the element back from the node above so we can display whatever data is in it (string,int,etc.)
public T getElement() {
return element;
}
//next we need to retrieve the pointer to return the next node
public Node getNext(){
return next;
}
//if we need to change what the pointer is pointing to:
public void setNext(Node n) {//this is going to take a node of the same parameter of a node of the same type
}
}
//singly linked list implementation
//Initialization stage of the singly linked list so both head and tail will be empty
private Node head = null;
private Node tail = null;
private int size = 0; //keeping track of the size of linked list using (size)
public practice() {}; //(name of the file) empty initializer when I don't have anything going on
public int size() {//getting the size back from the singly linked list
return size;
}
public boolean isEmpty() {//return true if the list is empty
return size == 0;
}
public T first(){ //getting the first element in the list
if(isEmpty()) { //if size == o then return nothing
return null;
}//else
return head.getElement(); //if not empty then return the value of the head node
}
public T last(){ //getting the value of the tail node
if(isEmpty()) {
return null;
}//else
return tail.getElement();
}
//this function allows you to add an element to the list itself. You can either add it at the head (beginning) of the linked list and the end (tail) of the linked list
public void addFirst(T e) {//Remember the T = generic, e = element being added
head = new Node<>(e, head);//create a new node which has our node (e) being added and it will point to the head.
if(size == 0) {//remember they (tail/head) have been originally initialized to NULL
tail = head;
}
size++;//size increased
System.out.println("Added head node with " + head.getElement() + " element.");
}
public void addLast(T e) {//adding element at the end (tail) of linked list
Node newNode = new Node<>(e, null);//tail is originally == NULL
if(isEmpty()) {//if we have no other elements within our list the head is going to be the new element added
head = newNode;
}else {
tail.setNext(newNode);
}
tail = newNode;
size++;
System.out.println("Added tail node with " + tail.getElement() + " element.");
}
}
Step by Step Solution
3.60 Rating (154 Votes )
There are 3 Steps involved in it
Step: 1
To create JUnit tests for your practice class that implements a singly linked list you can follow th...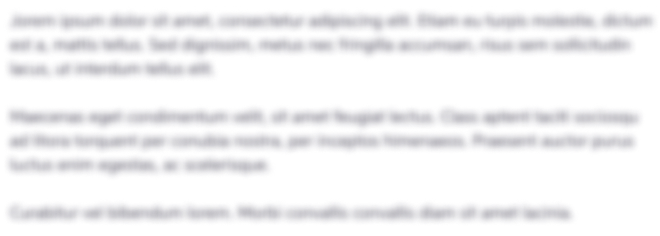
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started