Question
Make use of the following three java classes, and derby.jar file, and construct the MAIN method where user can input data, (in other words user
Make use of the following three java classes, and derby.jar file, and construct the MAIN method where user can input data, (in other words user can inter act with the methods from other class. I think for derby.jar, it can be downloaded here: https://db.apache.org/derby/derby_downloads.html
Complete this main method by using other classes and derby
MAIN CLASS given below:
public class EmployeeSystem {
public static void main(String[] args) throws ClassNotFoundException, IOException, SQLException {
//COMPLETE THIS MAIN METHOD
}
}
SQL CLASS given below:
import java.io.IOException;
import java.sql.*;
import java.util.*;
public class SQL {// runs the SQL codes for other class use.
//Initializes the database.
public static void Initialize() throws IOException, SQLException,
ClassNotFoundException {
String start = "database.properties";
SimpleDataSource.init(start);
}
public static void defaultSet () throws SQLException{
try (Connection conn = SimpleDataSource.getConnection())
{
comAddTable("Employees", "(employeeID INT, Name varchar(40), SSN INT, maritalStatus varchar(20), hourlyPayRate double)");
comAddTable("WeekHours", "(employeeID INT, hoursWorked INT, weekNum INT)");
comAddTable("EmployeeTotals", "(employeeID INT, totalGrossPay double, totalFedralTaxes double, totalFICATaxes double, totalMedicareTaxes double, totalStateTaxes double, totalNetPay double)");
comAddTable("Checks", "(checkNum int, employeeID INT, ammount double, dateIssued varchar(10), grossPay double, deductFederal double, FICA double, Medicare double, stateTaxes double)");
}
}
public static void rowsTest() throws SQLException{
ComInsertRow("Employees", "(0, 'testName', 000000000, 'no', 7.25)");
ComInsertRow("WeekHours", "(0, 100, 0)");
ComInsertRow("EmployeeTotals", "(0, 500.00, 10.00, 15.00, 5.00, 12.00, 542.00)");
ComInsertRow("Checks", "(0, 0, 500.00, '12/4/17', 500.00, 10.00, 15.00, 5.00, 12.00)");
}
//Runs SQL run generated completly by the user. in-progress.
public static void comLine() throws SQLException{
try (Connection conn = SimpleDataSource.getConnection())
{
Statement stat = conn.createStatement();
Scanner in = new Scanner (System.in);
System.out.println("Please type your full SQL line, to print a query type PRINT, to exit type DONE.");
}catch (SQLException e) {
e.printStackTrace();
}
}
//Runs SQL to add a table via human input.
public static void AddTable () throws SQLException {
try (Connection conn = SimpleDataSource.getConnection())
{
Statement stat = conn.createStatement();
Scanner in = new Scanner (System.in);
System.out.println("Name of the Table: (no spaces)");
String tableName = in.next();
System.out.println("Proper Syntax is (CollumnName DateType), please type done to quit. or datatype for list of datatypes.");
String input;
ArrayList
boolean cont = true;
while (cont = true){
input = in.nextLine();
if (input.equalsIgnoreCase("done")){
cont = false;
}
else if(input.equalsIgnoreCase("datatype")) {
System.out.println("data types include:");
System.out.println("INT");
System.out.println("FLOAT");
System.out.println("REAL");
System.out.println("DOUBLE");
System.out.println("DECIMAL(totalDigits, digitsAfterDecimal)");
System.out.println("BOOLEAN");
System.out.println("VARCHAR(length) this is variable length string.");
System.out.println("CHAR(length) fixed length string.");
}
else {
sections.add(input);
}
String connect= sections.get(0);
for (int i=1; i connect = connect + ", " + sections.get(i); } stat.execute("CREATE TABLE "+tableName+ " ("+ connect+ " )"); } in.close(); }catch (SQLException e) { e.printStackTrace(); } } //Runs SQL to add a table via computer input. public static void comAddTable(String tableName, String columns) throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); stat.execute("CREATE TABLE "+tableName+ " "+ columns); }catch (SQLException e) { e.printStackTrace(); } } //Runs SQL to add a row to a table. public static void InsertRow() throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); Scanner in = new Scanner (System.in); System.out.println("Table to add row: (tableName or tableName (columnName1, columnName2, ....)"); String table = in.nextLine(); System.out.println("Values listed: (Values listed in table order, or columnName given order."); String values = in.nextLine(); stat.execute("INSERT INTO " + table + " VALUES (" + values +" )"); in.close(); }catch (SQLException e) { e.printStackTrace(); } } public static void ComInsertRow(String table, String values) throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); stat.execute("INSERT INTO " + table + " VALUES " + values); }catch (SQLException e) { e.printStackTrace(); } } //Runs SQL to let a human to make changes to SQL tables. public static void AlterRow() throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) {// UPDATE SET WHERE Statement stat = conn.createStatement(); Scanner in = new Scanner (System.in); System.out.println("Table to be updated: (one word)"); String table = in.next(); System.out.println("Updated information: (collumnName = 'data' [if more data separate with ,]"); String set = in.nextLine(); System.out.println("Conditions: (collumnName = 'data' [if multiple conditions use AND OR NOT to connect]"); String conditions = in.nextLine(); stat.execute("UPDATE "+ table + " SET "+ set + " WHERE " + conditions); in.close(); }catch (SQLException e) { e.printStackTrace(); } } //Runs SQL to let a computer make changes to SQL tables. public static void ComAlterRow(String table, String set, String conditions) throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); stat.execute("UPDATE "+ table + " SET "+ set + " WHERE " + conditions +" ;"); }catch (SQLException e) { e.printStackTrace(); } } //Runs SQL to Remove a Row. public static void RemoveRow() throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); Scanner in = new Scanner (System.in); System.out.println("Table to remove row from: "); String table = in.next(); System.out.println("Conditions to remove rows: "); String conditions = in.nextLine(); stat.executeQuery("DELETE FROM " + table + " WHERE " + conditions); in.close(); }catch (SQLException e) { e.printStackTrace(); } } //Runs SQL to let a human generate a ResultSet. public static ResultSet UseQuery() throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); ResultSet result; Scanner in = new Scanner(System.in); System.out.println("please input SELECT input"); String Select = in.nextLine(); System.out.println("please input FROM input"); String From = in.nextLine(); System.out.println("please input WHERE input"); String Where = in.nextLine(); result = stat.executeQuery("SELECT"+Select+"FROM"+From+"WHERE"+Where); in.close(); return result; } } //Runs SQL to let a computer generate a ResultSet. public static void ComQuery(String Select, String From, String Where) throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { Statement stat = conn.createStatement(); ResultSet result = stat.executeQuery("SELECT"+Select+"FROM"+From+"WHERE"+Where); ResultSetMetaData meta = result.getMetaData(); int columnsNumber = meta.getColumnCount(); while (result.next()) { for (int i = 1; i <= columnsNumber; i++) { if (i > 1) System.out.print(", "); String columnValue = result.getString(i); System.out.print(columnValue + " " + meta.getColumnName(i)); } System.out.println(""); } } } //Prints a sent ResultSet. public static void PrintResultSet(ResultSet result) throws SQLException{ try (Connection conn = SimpleDataSource.getConnection()) { //System.out.print(result); System.out.print(result.getMetaData()); } } } SimpleDataSource Class given below: import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.io.FileInputStream; import java.io.IOException; import java.util.Properties; /** A simple data source for getting database connections. */ public class SimpleDataSource { private static String url; private static String username; private static String password; /** Initializes the data source. @param fileName the name of the property file that contains the database driver, URL, username, and password */ public static void init(String fileName) throws IOException, ClassNotFoundException { Properties props = new Properties(); FileInputStream in = new FileInputStream(fileName); props.load(in); String driver = props.getProperty("jdbc.driver"); url = props.getProperty("jdbc.url"); username = props.getProperty("jdbc.username"); if (username == null) { username = ""; } password = props.getProperty("jdbc.password"); if (password == null) { password = ""; } if (driver != null) { Class.forName(driver); } } /** Gets a connection to the database. @return the database connection */ public static Connection getConnection() throws SQLException { return DriverManager.getConnection(url, username, password); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
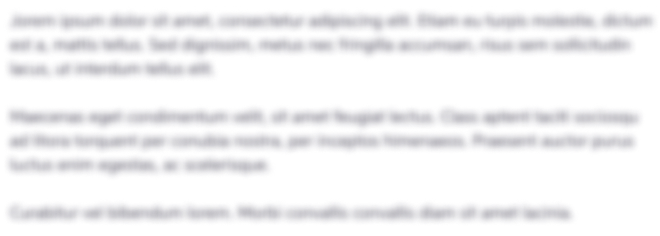
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started