Question
Makeamodulecalled **Stats** thatcanrepresentafileontheharddiskcontainingcomma-separatednumbers. Thismoduleshouldbeabletoloadthenumbersandperformthefollowingtasks. -A **Stats** objectshouldbeabletodisplaythenumbersinatabularformatonostreaminaspecifiednumberofcolumns,columnwidthandprecision. -A **Stats** objectshouldbeabletodisplaythenumbersthatfallwithinaspecificrangeandthenumberoftheiroccurrence. -A **Stats** objectshouldbeabletosortthenumbersinanascendingordescendingorder. -Copyinga **Stats** objectshouldbedonesafelyanddoingsoitshouldalsocopythedatafileontheharddisk.Thenewfilenameshouldbethesameastheoriginalfilewithanadded **C_** prefix. -Assigninga **Stats** objecttoanothershouldbedonesafelyandthecontentofthetargetfileshouldalsobeoverwrittenbythesourcefile. -A **Stats** objectshouldalsomakethenumbersavailabletotheuserprogrambyindexinglikeanarray. -A
Makeamodulecalled**Stats**thatcanrepresentafileontheharddiskcontainingcomma-separatednumbers.
Thismoduleshouldbeabletoloadthenumbersandperformthefollowingtasks.
-A**Stats**objectshouldbeabletodisplaythenumbersinatabularformatonostreaminaspecifiednumberofcolumns,columnwidthandprecision.
-A**Stats**objectshouldbeabletodisplaythenumbersthatfallwithinaspecificrangeandthenumberoftheiroccurrence.
-A**Stats**objectshouldbeabletosortthenumbersinanascendingordescendingorder.
-Copyinga**Stats**objectshouldbedonesafelyanddoingsoitshouldalsocopythedatafileontheharddisk.Thenewfilenameshouldbethesameastheoriginalfilewithanadded**"C_"**prefix.
-Assigninga**Stats**objecttoanothershouldbedonesafelyandthecontentofthetargetfileshouldalsobeoverwrittenbythesourcefile.
-A**Stats**objectshouldalsomakethenumbersavailabletotheuserprogrambyindexinglikeanarray.
-A**Stats**objectshouldbeabletoreceivethedatafilenamefromtheistreamandloadthedatafromthefileontheharddrive.
##Requiredpublicfunctionalities
###construction
####3ArgumentConstructor
```C++
Stats(unsignedcolumnWidth=15,
unsignednoOfColumns=4,
unsignedprecision=2);
```
-columnWidth:widthofeachcolumnincharacters
-noOfColumns:numberofcolumnstodisplaythenumbersin
-precision:numberofdigitsafterthedecimalpointforeachnumber
Inthiscase,the"**Stats**"objectisnottiedtoanyfileontheharddriveandisempty.
####4ArgumentConstructor
```C++
Stats(constchar*filename,
unsignedcolumnWidth=15,
unsignednoOfColumns=4,
unsignedprecision=2);
```
Sameasthepreviousconstructor,butinthiscase,ifthefilenameargumentisnotnullandissuccessfullyopened,thenumbersinthefilewillbeloadedintothe**Stats**object.
####CopyConstructor
Copyinga**Stats**objectshouldbedonesafelyanddoingsoitshouldalsocopythedatafileontheharddisk.Thenewfilenameshouldbethesameastheoriginalfilewithanadded**"C_"**prefix.
###Operatoroverloads
####Copyassignment
Assigninga**Stats**objecttoanothershouldbedonesafelyandthecontentofthetargetfileshouldalsobeoverwrittenbythesourcefile.
####Indexoperatoroverloads
```C++
double&operator[](unsignedidx);
```
Returnsthereferenceofthenumberatidxindex.Iftheindexexceedsthesizeofthearray,itshouldloopbacktothebeginning.Forexample,ifthearraysizeis10,index10willbethereferenceoftheelementatindex0andindex11willbethereferenceoftheelementatindex1.
Ifthe**Stats**objectisempty,itshouldreturnthereferenceofadummydoublemembervariable.
```C++
doubleoperator[](unsignedidx)const;
```
Returnsthevalueofthenumberatidxindex.Iftheindexexceedsthesizeofthearray,itshouldloopbacktothebeginning.Forexample,ifthearraysizeis10,index10willbethevalueoftheelementatindex0andindex11willbethevalueoftheelementatindex1.
Ifthe**Stats**objectisempty,itshouldreturnzero.
###Booleantypeconversionoverload
If**Stats**iscastedtoboolean,itshouldreturntrueonlyifitisnotempty.Otherwise,itshouldreturnfalse.
###Methods(Membervariables)
####sort()
```C++
voidsort(boolascending);
```
Sortsthenumbersinascendingordescendingorder.
####size()
```C++
unsignedsize()const;
```
returnsthenumberofnumbersinthe**Stats**object.
####name()
```C++
constchar*name()const;
```
returnsthenameofthefiletiedtothe**Stats**object.
####occurrence()
```C++
unsignedoccurrence(doublemin,doublemax,std::ostream&ostr=std::cout);
```
displaysthenumbersthatfallwithinaspecificrangeandthenumberoftheiroccurrenceonostream.
Theformatoftheprintoutshouldbethesameasprintingallthenumbers.
##helperimplementations
###ostreaminsertionoperator<<
**Stats**shouldbeprintablebyostream(cout)usingthe```operator<<```.
AStatsobjectshouldbeabletodisplaythenumbersinatabularformatonostreaminaspecifiednumberofcolumns,columnwidthandprecision.Seetheexecutionsamplefile.
###istreamextractionoperator>>
**Stats**objectshouldbeabletoreceivethedatafilenamefromostream(cin)usingoperator>>.Afterreceivingthename,ifthedatafileisopensuccessfully,thenumbersshouldbeloadedintothe**Stats**object.
##Thetesterprogram:
>Modifythetesterprogramtotestallthedifferentcircumstances/casesoftheapplicationifdesiredandnotethattheprofessor'stestermayhavemanymoresamplesthanthetesterprogramhere.
```C++
//Workshop6-diy:
//Version:0.9
//Date:2021/02/20
//Author:FardadSoleimanloo
//Description:
//Thisfileteststhediysectionofyourworkshop
/////////////////////////////////////////////
#include
#include
#include
#include"Stats.h"
usingnamespacesdds;
usingnamespacestd;
voidtwentyNumbers(StatsT);
voidbadIndex(constStats&T);
voidCopy(conststring&dest,conststring&source);
voidDump(conststring&filename);
intmain(){
Copy("numLtl.csv","numbersLtlOriginal.csv");
Copy("numbers.csv","numbersOriginal.csv");
StatsL(10,7,0);
StatsS("numLtl.csv"),Empty,BadFilename("badFilename");
cout<<"Enterthedatafilename:";
cin>>L;
cout< cout< twentyNumbers(L); twentyNumbers(S); cout<<"Totalof"< L[1000]=11111.11; L.occurrence(-12345.0,31342.55); L.sort(true); cout<<"SortedAscending:"< L.occurrence(-12345.0,31342.55); L.sort(false); cout<<"SortedDescending:"< L.occurrence(-12345.0,31342.55); L=S; cout< cout<<"============================================================"< Dump("numbers.csv"); Dump("numLtl.csv"); Dump("C_numbers.csv"); Dump("C_numLtl.csv"); cout<<"Emptyobjecttests:"< cout<<"*"< cout< badIndex(Empty); Empty.sort(true); Empty.occurrence(1,2); cout< return0; } voidtwentyNumbers(StatsT){ if(T){ cout<<">>>20numbersof:"< cout.setf(ios::fixed); cout.precision(1); for(unsignedi=0;i<200;i+=10){ cout<<"T["< } cout.unsetf(ios::fixed); } else{ cout<<"Nothingtoprint!"< } cout< } voidDump(conststring&filename){ cout<<"DUMP---------------------------------------------------------"< cout<<">>>"< ifstreamfin(filename.c_str()); inti=0; charch; while(fin.get(ch)){ if(i++%80==0)cout< cout< } cout< } voidCopy(conststring&dest,conststring&source){ ifstreamfin(source.c_str()); ofstreamfout(dest.c_str()); charch; while(fin.get(ch))fout< } voidbadIndex(constStats&T){ cout< } ``` ##Executionoutputsample [OutputSample](DIY/ExecutionOutputSample.txt)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
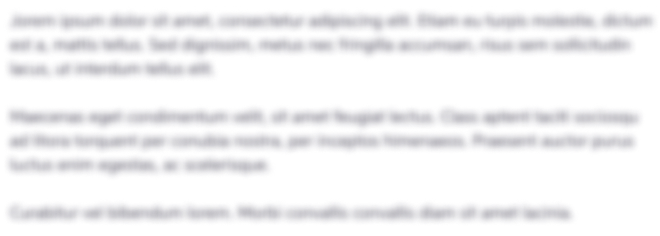
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started