Making a java program that's an Integer calculator. You will provide an infix string as input into the Calculator program. The Calculator program will display
Making a java program that's an Integer calculator. You will provide an infix string as input into the Calculator program. The Calculator program will display the postfix notation and evaluate the postfix notation. I have tried some things but the tests always fail and the evaluation is always wrong. Let me know if you need more info. Thank you! Instructions Below: Need help with steps 2-4. I have already made the stack using GenericList.
- You will need to create a stack data structure. You can either use the GenericList class or SingleLinkList class you created in previous classes. Use the MyStack.java interface provided. Do Not Change the MyStack.java interface.
- Implement the method infixToPostfix in the Calculator class. I have included a complete set of comments for all the steps to implement the Shunting Yard algorithm. Do not change the comments. Place your code for each comment under the comment. You will need to implement a stack to hold the operators as you perform the Shunting Yard algorithm.
- Implement the method evaluatePostfix in the Calculator class. I have included a complete set of comments for all the steps to implement evaluating postfix notation. Do not change the comments. Place your code for each comment under the comment. You will need to implement a stack of type Integer to hold the operands as you perform evaluation of the postfix notation.
- Use CalculatorTest.java to test your code. Cannot Change the Tests
- Have include a Parse code with methods that assist in converting infix to postfix notation. Use the methods in this class to complete your homework assignment.
//Calculator source - take an infix string, change to postfix notation and evaluate.
public class Calculator
{
// Take an infix arithmetic expression and return a postfix arithmetic expression.
public String infixToPostfix(String infixNotation){
// 1. Read a token from the infix expression.
// 2. If the token is a number, then add it to the postfix expression.
// 3. If the stack is empty or contains a left parenthesis on top,
// push the token onto the stack
// 4. If the token is a left parenthesis, push it on the stack.
// 5. If the token is a right parenthesis, pop the stack and add value
// to the postfix expression. Repeat until you see a left parenthesis.
// Discard the left and right parenthesis.
// 6. If the token is ^ (which is right associative) and
// has equal precedence as top of stack
// ( the only operation of equal precedence is ^ ), push it onto the stack
// and continue to the next token.
// This is the second half of the question in step 6.
// Does the token have higher precedence than top of stack,
// push it on the stack.
// 7. If the token has precedence equal to or less than the top of stack,
// pop the stack and add value to the postfix expression.
// Then test the token against the new top of stack and repeat.
// Push the token onto the stack.
// 8. At the end of the expression,
// pop all values from the stack and add to the output queue.
}
public int evaluatePostfix(String postfixNotation)
{
// 1. Push operands onto the stack.
// 2. When you see an operator, pop the first operand off the stack
// and place to the right of the operator. Pop the next operand
// off the stack and place to the left of the operator.
// 3. Perform the operation and push the result onto the stack.
// 4. Repeat from step 1 until expression is completed.
// 5. The value at the top of the stack is the result.
}
public static void main(String[] args){
String infixNotation;
String postfixNotation;
int calculatedValue;
// Take the first argument from the command line as a string with
// infix notation.
if (args.length == 0)
{
System.out.println("You need to enter an infix notation string");
System.exit(1);
}
infixNotation = args[0];
System.out.println("You entered: " + infixNotation);
postfixNotation = new Calculator().infixToPostfix(infixNotation);
System.out.println("Postfix notation: " + postfixNotation);
calculatedValue = new Calculator().evaluatePostfix(postfixNotation);
System.out.println("Result of evaluation: " + calculatedValue);
}
}
Test code-using junit 4.12 The code has to pass these tests.
import static org.junit.Assert.*;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class CalculatorTest//Test Calculator class
{
@Test
public void testInfixToPostfix1()
{
Calculator c = new Calculator();
assertEquals("2 3 4 * + 5 - ", c.infixToPostfix("2 + 3 * 4 - 5"));
}
@Test
public void testInfixToPostfix2()
{
Calculator c = new Calculator();
assertEquals("5 3 - 2 2 / + ", c.infixToPostfix("5 - 3 + 2 / 2"));
}
@Test
public void testInfixToPostfix3()
{
Calculator c = new Calculator();
assertEquals("3 2 3 ^ * 1 + ", c.infixToPostfix("3 * 2 ^ 3 + 1"));
}
@Test
public void testInfixToPostfix4()
{
Calculator c = new Calculator();
assertEquals("1 4 + 5 2 - * ", c.infixToPostfix("( 1 + 4 ) * ( 5 - 2 )"));
}
@Test
public void testInfixToPostfix5()
{
Calculator c = new Calculator();
assertEquals("7 1 4 2 - + 2 ^ * 5 * ", c.infixToPostfix("7 * ( 1 + ( 4 - 2 ) ) ^ 2 * 5"));
}
@Test
public void testInfixToPostfix6()
{
Calculator c = new Calculator();
assertEquals("3 4 2 * 1 5 - 2 3 ^ ^ / + ", c.infixToPostfix("3 + 4 * 2 / ( 1 - 5 ) ^ 2 ^ 3"));
}
@Test
public void testInfixToPostfix7()
{
Calculator c = new Calculator();
assertEquals("3 -4 2 * 1 5 - 2 3 ^ ^ / + ", c.infixToPostfix("3 + -4 * 2 / ( 1 - 5 ) ^ 2 ^ 3"));
}
@Test
public void testInfixToPostfix8()
{
Calculator c = new Calculator();
assertEquals("3 4 2 * 1 5 - 2 3 ^ ^ * + ", c.infixToPostfix("3 + 4 * 2 * ( 1 - 5 ) ^ 2 ^ 3"));
}
@Test//to check the second half of step 6
public void testInfixToPostfix9()
{
Calculator c = new Calculator();
assertEquals("2 3 4 * + ", c.infixToPostfix("2 + 3 * 4"));
}
@Test//to check the second half of step 6
public void testInfixToPostfix10()
{
Calculator c = new Calculator();
assertEquals("2 3 2 ^ * ", c.infixToPostfix("2 * 3 ^ 2"));
}
@Test//to check the second half of step 6
public void testInfixToPostfix11()
{
Calculator c = new Calculator();
assertEquals("2 3 2 ^ ^ ", c.infixToPostfix("2 ^ 3 ^ 2"));
}
@Test
public void testEvaluatePostfix1()
{
Calculator c = new Calculator();
assertEquals(9, c.evaluatePostfix("2 3 4 * + 5 - "));
}
@Test
public void testEvaluatePostfix2()
{
Calculator c = new Calculator();
assertEquals(3, c.evaluatePostfix("5 3 - 2 2 / + "));
}
@Test
public void testEvaluatePostfix3()
{
Calculator c = new Calculator();
assertEquals(25, c.evaluatePostfix("3 2 3 ^ * 1 + "));
}
@Test
public void testEvaluatePostfix4()
{
Calculator c = new Calculator();
assertEquals(15, c.evaluatePostfix("1 4 + 5 2 - * "));
}
@Test
public void testEvaluatePostfix5()
{
Calculator c = new Calculator();
assertEquals(315, c.evaluatePostfix("7 1 4 2 - + 2 ^ * 5 * "));
}
@Test
public void testEvaluatePostfix6()
{
Calculator c = new Calculator();
assertEquals(3, c.evaluatePostfix("3 4 2 * 1 5 - 2 3 ^ ^ / + "));
}
@Test
public void testEvaluatePostfix7()
{
Calculator c = new Calculator();
assertEquals(-3, c.evaluatePostfix("-3 4 2 * 1 5 - 2 3 ^ ^ / + "));
}
@Test
public void testEvaluatePostfix8()
{
Calculator c = new Calculator();
assertEquals(524291, c.evaluatePostfix("3 4 2 * 1 5 - 2 3 ^ ^ * + "));
}
@Test
public void testEvaluatePostfix9()
{
Calculator c = new Calculator();
assertEquals(-524285, c.evaluatePostfix("3 4 -2 * 1 5 - 2 3 ^ ^ * + "));
}
@Test//test step 6
public void testEvaluatePostfix10()
{
Calculator c = new Calculator();
assertEquals(14, c.evaluatePostfix("2 3 4 * +"));
}
@Test//test step 6
public void testEvaluatePostfix11()
{
Calculator c = new Calculator();
assertEquals(18, c.evaluatePostfix("2 3 2 ^ *"));
}
@Test//test step 6
public void testEvaluatePostfix12()
{
Calculator c = new Calculator();
assertEquals(512, c.evaluatePostfix("2 3 2 ^ ^"));
}
}
public class Parse
{
private int tokenPointer;
private String[] tokens;
private int numTokens;
public Parse(String input)
{
// Break string into tokens. One or more consecutive spaces separates tokens.
this.tokens = input.split(" +");
this.tokenPointer = 0;
this.numTokens = tokens.length;
}
public int size()
{
return numTokens;
}
public String toString()
{
String output = "No Tokens";
if (! tokens[0].isEmpty())
{
output = tokens[0];
for (int i=1; ioutput = output + ":" + tokens[i];
}
return output;
}
// Every time this method is called, the next token in the tokens array is
// returned. The tokenPointer is incremented. A token of "" denotes no more
// tokens are available.
public String getNextToken()
{
String token = "";
if (tokenPointer == 0 && tokens[0].isEmpty())
return token;
else
{
if (tokenPointer < numTokens)
{
token = tokens[tokenPointer];
tokenPointer++;
return token;
}
else
return token;
}
}
// This method is equivalent to the expression
// (left isLessThanOrEqualto right)
public boolean lessThanEqualTo(String left, String right)
{
return (tokenPrecedence(left) <= tokenPrecedence(right));
}
// Return true if we have found an operator. If false, assume you have
// an operand or number.
public boolean isOperator(String token)
{
char tkn = token.charAt(0);
switch (tkn)
{
case ')': return true;
case '+': return true;
// If we see a minus we could have the subtract operator or
// the first character of a negative number
case '-': { if ( token.matches("-[0123456789]+") )
return false; // We have a negative number
else
return true;}
case '*': return true;
case '/': return true;
case '^': return true;
case '(': return true;
default: return false;
}
}
// Indicates the precedence from 1 (lowest) to 3 (highest) of an operator.
// This is used by method lessThanEqualTo.
private int tokenPrecedence(String token)
{
char tkn = token.charAt(0);
switch (tkn)
{
case '+': return 1;
case '-': return 1;
case '*': return 2;
case '/': return 2;
case '^': return 3;
default: return 0; // Undefined token
}
}
}
Step by Step Solution
3.35 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
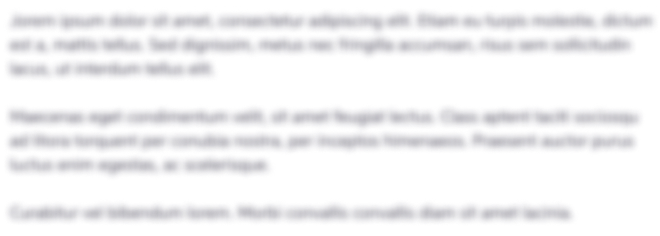
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started