Question
Menu Implementation (ADT Implementation Using Arrays) Summary Implement the MenuInterface interface using an array . (NOT an ArrayList or any other data structure.) Your implementation
Menu Implementation (ADT Implementation Using Arrays)
Summary
Implement the MenuInterface interface using an array. (NOT an ArrayList or any other data structure.) Your implementation must provide suitable code for each of the operations in that interface, and also seven (7) constructors (see below).
Details
I have provided you with an interface that's based on Dr. Scobey's Menu class. It is, however, slightly different.
I have also provided you with a sample client program.
You SHOULD NOT modify those files.
More about the menu class:
A menu is a list of options that the user may choose from. The menu optionally has a title that appears at its top.
Our menus' options will be numbered, and the user will choose an option by typing in the corresponding number at a prompt.
The getChoice method gives the user three chances to enter a valid choice, prompting for each input. If a valid choice gets entered, it is returned immediately. If no valid choice is entered in three tries, the method returns -1.
Typing a non-integer at the prompt results in the method returning -1 immediately. The input stream should be left unchanged.
Your job is to create an array-based implementation of the MenuInterface. Your implementation must be called ArrayMenu.
Much of the code you need can be copied (and lightly modified) from the ArrayBag class provided in the sample code.
Note that the Menu should not grow. Once all available slots have been filled in, any attempt to add an option will fail.
Make sure your implementation is correct according to all behaviours described in the interface documentation.
You must provide the following constructors:
If no title is provided, then the menu has no title. If no options are provided, the menu starts with no options. If no maximum size is given, then either: an array of options has been given, and that is the maximum number of options for the array; or no array of options has been given, and a default maximum number of options is given.
The title may be null, but the array of starting options can't be. If any constructor is given null where an array of options is expected, it should throw a NullPointerException.
If the maximum number of options is less than the number of starting options, then the constructor should throw an IllegalArgumentException with a suitable message.
A constructor that takes a title, an array of String (the options), and an integer giving the maximum number of options that this Menu can be given.
A constructor that takes a title and an array of Strings (which are the options for this menu).
A constructor that takes just a title.
A constructor that takes just an array of Strings (which are the options for this menu).
A constructor that takes a title and an int value (which is the maximum number of options for this menu -- attempting to add more options than that will fail).
A constructor that takes just an int value (again, the the maximum number of options).
A no-arguments constructor.
__________________________________________________________________________________________________________________________________
A03.java
/** * A program to test the ArrayMenu implementation of MenuInterface, using a * text adventure style game. * * @author Mark Young (A00000000) */ public class A03 { private static final Scanner kbd = new Scanner(System.in); public static void main(String[] args) { // create variables String[] allWays = new String[]{"north", "east", "south", "west"}; MenuInterface[] rooms = new MenuInterface[]{ // constructor with just a title new ArrayMenu("You are in the start room. You can go:"), // constructor with title and initial capacity new ArrayMenu("You are in room 1. You can go:", 3), // constructor with title and options new ArrayMenu("You are in room 2. You can go:", new String[]{"northerly", "southerly", "westerly"}), // constructor with just initial capacity new ArrayMenu(4), // constructor with just options new ArrayMenu(new String[]{"northish", "eastish", "southish"}), // no-args constructor new ArrayMenu() }; int[][] exits = new int[][]{ // where we go ... new int[]{1, 2, 3, 4}, // from room[0] new int[]{2, 0, 4}, // from room[1] new int[]{1, 3, 0}, // from room[2] new int[]{0, 2, 5, 4}, // from room[3] new int[]{1, 0, 3}, // from room[4] new int[]{3} // from room[5] }; int currentRoom; int choice; final int END_ROOM = 5; // print introduction printIntroduction(); // finish making the Menus rooms[0].addOptions(allWays); rooms[1].addOption("sorta east"); rooms[1].addOption("sorta south"); rooms[1].addOption("sorta west"); rooms[3].addOptions(new String[]{"north", "east"}); rooms[3].addOptions(new String[]{"south", "west"}); rooms[4].setTitle("You are in room 4. You can go:"); rooms[5].setTitle("This is the END room!"); // neither of these commands should alter the program's behaviour allWays[0] = "to the great white north"; if (rooms[3].addOption("up, up, and away!")) { System.out.println("It added an option when it shouldn't have!"); } // start wandering from room 0 currentRoom = 0; // while we haven't got to the end room while (currentRoom != END_ROOM) { // display the menu for this room and get the choice rooms[currentRoom].display(); choice = rooms[currentRoom].getChoice(kbd); // deal with the choice if (choice > 0) { // move to the selected room currentRoom = exits[currentRoom][choice - 1]; } else { // invalid response -- check for quitting System.out.println(); if (confirm("Would you like to quit? ")) { System.out.println("Seeya, quitter!"); showMenus(rooms, exits); System.exit(0); } } } // finish up with a test of the empty menu System.out.println(); try { rooms[END_ROOM].display(); rooms[END_ROOM].getChoice(kbd); System.out.println("getChoice did not throw IllegaStateEx^n!"); } catch (IllegalStateException ise) { System.out.println("Congratulations! You made it to the end!"); } System.out.println(); // print out all the menu contents: showMenus(rooms, exits); // test exceptions testExceptions(); } /** * Check whether the constructors throw the correct exceptions. */ private static void testExceptions() { try { MenuInterface bad = new ArrayMenu("OK", null, 10); System.out.println("Constructor accepted null options array!"); } catch (NullPointerException npe) { } try { MenuInterface bad = new ArrayMenu("OK", new String[5], 3); System.out.println("Constructor accepted over-large options array!"); } catch (IllegalArgumentException iae) { } try { MenuInterface bad = new ArrayMenu("OK", null); System.out.println("Constructor accepted null options array!"); } catch (NullPointerException npe) { } try { MenuInterface bad = new ArrayMenu("OK", -1); System.out.println("Constructor accepted illegal maximum size!"); } catch (IllegalArgumentException iae) { } } /** * Show all the menus corresponding to the given rooms. * * @param rooms the rooms to show */ private static void showMenus(MenuInterface[] rooms, int[][] exits) { for (int i = 0; i < rooms.length; ++i) { MenuInterface menu = rooms[i]; System.out.println("Menu named " + quote(menu.getTitle()) + " has:"); String[] menuOptions = menu.getOptions(); for (int j = 0; j < menuOptions.length; ++j) { String option = menuOptions[j]; System.out.println("\t" + quote(option) + " --> " + quote(rooms[exits[i][j]].getTitle())); } } } /** * Change a String into a quoted String. Leave nulls unchanged! * * @param str the String to quote * @return str surrounded by double-quotes, or null if str is null */ private static String quote(String str) { if (str == null) { return null; } else { return '"' + str + '"'; } } /** * Print an introductory paragraph for this program. */ private static void printIntroduction() { System.out.println("Menu Demo Program ----------------- " + "This program uses menus to let you wander thru a virtual " + "building. Try to get to the end room by choosing which " + "direction to go. " + "You can quit any time by typing Q at the prompt. " + "by Mark Young (A00000000) "); } /** * Get a YES/NO answer to a question. Keep asking until we get a YES or * NO answer. * * @param question the question to get an answer to. * @return true if the user said YES; false if they said NO. */ private static boolean confirm(String question) { String answer; // until we get a valid answer while (true) { // ask the question System.out.println(); System.out.print(question); answer = kbd.next().toUpperCase(); kbd.nextLine(); // YES if ("YES".startsWith(answer)) { return true; } // NO if ("NO".startsWith(answer)) { return false; } // Neither YES nor NO System.out.println("Please answer yes or no."); } } }
__________________________________________________________________________________________________________________________________
MenuInterface.java
import java.util.Scanner; /** * An interface for menu objects for console programs. * A simple use case would be: * ** MenuInterface mainMenu = new MenuImpl("Main Menu"); * mainMenu.addOption("New"); * mainMenu.addOption("Open"); * mainMenu.addOption("Save"); * mainMenu.addOption("Quit"); * do { * mainMenu.display(); * executeChoice(mainMenu.getChoice(kbd)); * } while (true); ** * @author Mark Young (A00000000) */ public interface MenuInterface { /** * Get the title of an already existing menu. * * @return The title given to the menu. */ public String getTitle(); /** * Get a list of the options for this Menu. The list will contain all and * only the options for this menu. * * @return An array containing the text of the options that have been added * to this menu. */ public String[] getOptions(); /** * Set (or reset) the title of an already existing menu. * * @param menuTitle The title to be given to the menu. * @return true if the title was changed; false otherwise. */ public boolean setTitle(String menuTitle); /** * Add an option to the menu. * * @param option The text of the option to be added to the menu. * @return true if the option was added; false otherwise. */ public boolean addOption(String option); /** * Add multiple options to the menu. Either all the options will be added * or none will. * * @param options An array containing the text of the options to be added * to the menu. * @return true if the options were added; false otherwise. */ public boolean addOptions(String[] options); /** * Display the menu title (if any) and options (if any), with a blank * line between the title and the options (if they both exist). The * menu has at least two blank lines before it on the screen, and * exactly one blank line after the options. The options are numbered, * and the number is printed right-justified in a field four spaces * wide. The number is followed by a period and a space, and then the * text of the option. For example: ** * * Main Menu * * 1. New * 2. Open * 3. Save * 4. Quit * **/ public void display(); /** * Prompt the user to enter a menu choice and then reads the choice * entered by the user at the keyboard. If the method is called for a * menu that has zero options, an IllegalStateException is thrown. * * The user is prompted for input, and is given three chances to enter * a valid choice. * * Returns -1 if the reading fails for any reason. * * @param in the Scanner to read the choice from (usually on System.in) * @return the number of the option chosen; or -1 if no valid option * was chosen. * @throws IllegalStateException if this Menu has no options. */ public int getChoice(Scanner in); }
__________________________________________________________________________________________________________________________________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
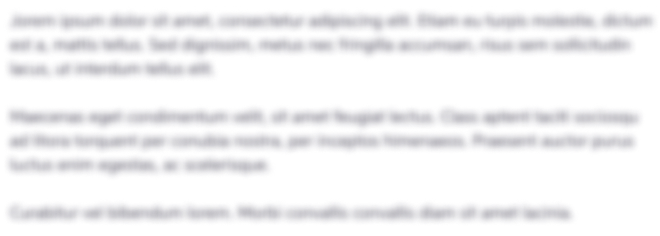
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started