Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Method (member function) SinglyLinkedList::insertAfter(), defined in: #pragma once #include template struct Node { T data; Node * next; Node() = delete; // Intentionally no default
Method (member function) SinglyLinkedList::insertAfter(), defined in:
#pragma once | |
#include | |
template | |
struct Node { | |
T data; | |
Node | |
Node() = delete; // Intentionally no default constructor | |
Node( const T & element ) : data( element ), next( nullptr ) {} | |
}; | |
template | |
class SinglyLinkedList { | |
private: | |
Node | |
Node | |
public: | |
// Constructors | |
SinglyLinkedList(); | |
SinglyLinkedList(const SinglyLinkedList&); | |
SinglyLinkedList& operator=(const SinglyLinkedList&); // assignment operator | |
~SinglyLinkedList(); // destructor | |
// Getters / Setters | |
bool empty(); | |
int size() = delete; // INTENTIONALLY NOT IMPLEMENTED !! | |
void append( const T& ); | |
void prepend( const T& ); | |
void insertAfter( Node | |
void removeAfter( Node | |
void pop_front(); // remove element at front of list | |
T& front(); // return list's front element | |
T& back(); // return list's back element | |
void clear(); | |
}; | |
template | |
SinglyLinkedList | |
template | |
bool SinglyLinkedList | |
return head == nullptr; | |
} | |
template | |
void SinglyLinkedList | |
Node | |
if (head == nullptr) { // List empty | |
head = newNode; | |
tail = newNode; | |
} | |
else{ | |
tail->next = newNode; | |
tail = newNode; | |
} | |
} | |
template | |
void SinglyLinkedList | |
Node | |
if (head == nullptr) { // list empty | |
head = newNode; | |
tail = newNode; | |
} | |
else { | |
newNode->next = head; | |
head = newNode; | |
} | |
} | |
template | |
void SinglyLinkedList | |
// Construct new node | |
Node | |
if (head == nullptr) { // List empty | |
head = newNode; | |
tail = newNode; | |
} else if (curNode == tail) { // Insert after tail | |
tail->next = newNode; | |
tail = newNode; | |
} else { | |
newNode->next = curNode->next; | |
curNode->next = newNode; | |
} | |
} | |
template | |
void SinglyLinkedList | |
if( empty() ) throw std::length_error( "empty list" ); | |
// Special case, remove head | |
if (curNode == nullptr && head != nullptr) { | |
Node | |
head = sucNode; | |
if (sucNode == nullptr) { // Removed last item | |
tail = nullptr; | |
} | |
} | |
else if (curNode->next != nullptr) { | |
Node | |
curNode->next = sucNode; | |
if (sucNode == nullptr) { // Removed tail | |
tail = curNode; | |
} | |
} | |
} | |
template | |
void SinglyLinkedList | |
removeAfter(nullptr); | |
} | |
template | |
T& SinglyLinkedList | |
if( empty() ) throw std::length_error( "empty list" ); | |
return head->data; | |
} | |
template | |
T& SinglyLinkedList | |
if( empty() ) throw std::length_error( "empty list" ); | |
return tail->data; | |
} | |
template | |
void SinglyLinkedList | |
while( !empty() ) | |
pop_front(); | |
} | |
template | |
SinglyLinkedList | |
clear(); | |
} | |
template | |
SinglyLinkedList | |
// Walk the original list adding copies of the elements to this list maintaining order | |
for( Node | |
append( position->data ); | |
} | |
} | |
template | |
SinglyLinkedList | |
if( this != &rhs ) // avoid self assignment | |
{ | |
// Release the contents of this list first | |
clear(); // An optimization might be possible by reusing already allocated nodes | |
// Walk the right hand side list adding copies of the elements to this list maintaining order | |
for( Node | |
append( position->data ); | |
} | |
} | |
return *this; | |
} |
takes two parameters: a pointer (curNode) and a value (newData).
1a. What does the method do if curNode == nullptr but head is not null? (Answer in 1-2 sentences. Be specific: refer to specific lines in the code as necessary.)
b. Modify the method such that when curNode == nullptr, the function will insert the value (newData) at the front of the linked list. Write the modified method.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
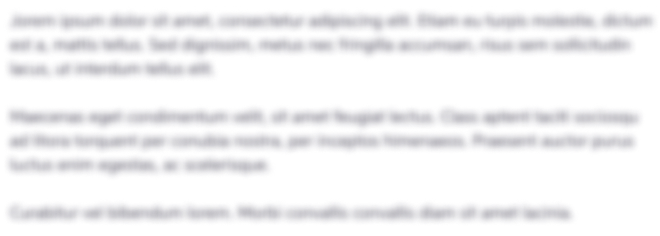
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started