Question
Milestone 4: scheduler.cpp #include #include scheduler.hpp void Course::display() const { std::string start_leading; std::string end_leading; int start_hr = start_time_ / HR_MIN_SPLITTER; int start_min = start_time_ %
Milestone 4:
scheduler.cpp
#include #include "scheduler.hpp"
void Course::display() const { std::string start_leading; std::string end_leading;
int start_hr = start_time_ / HR_MIN_SPLITTER; int start_min = start_time_ % HR_MIN_SPLITTER; int end_hr = end_time_ / HR_MIN_SPLITTER; int end_min = end_time_ % HR_MIN_SPLITTER;
if (start_min
void display_courses(Course *courses[], int size) { for (int i = 0; i
bool load_schedule(const std::string & filename, Course *ptr[], int & size) { std::ifstream input_file; bool valid_format = true; size = 0;
input_file.open(filename);
if (!input_file) { valid_format = false; std::cout
while (getline(input_file, course) && valid_format) {
start_colon = ' '; end_colon = ' ';
if (course.empty()) { valid_format = false; std::cout
else if (!getline(input_file, location)) { valid_format = false; std::cout
else if (!getline(input_file, day_of_week)) { valid_format = false; std::cout
else if (!(input_file >> start_hr >> start_colon >> start_min)) { valid_format = false; std::cout HR_MAX || start_min MIN_MAX || start_colon != ':') { valid_format = false; std::cout = 0) ? "0" : "")
else if (!(input_file >> end_hr >> end_colon >> end_min)) { valid_format = false; std::cout HR_MAX || end_min MIN_MAX || end_colon != ':') { valid_format = false; std::cout = 0) ? "0" : "")
else if (end_hr = 0) ? "0" : "") = 0) ? "0" : "")
input_file.ignore();
int combined_StartTime = start_hr * HR_MIN_SPLITTER + start_min;
int combined_EndTime = end_hr * HR_MIN_SPLITTER + end_min;
ptr[size] = new Course(course, location, day_of_week, combined_StartTime, combined_EndTime);
size++; }
input_file.close(); }
if (!valid_format) { std::cout
return valid_format; }
bool has_conflict(TimeSpan temp, TimeSpan appointments[], int num_appointments) { for (int i = 0; i appointments[i]) || (temp
return true; } return false; }
scheduler.hpp
#include #include #include
const int MIN_MIN = 0; const int HR_MIN = 0; const int MIN_MAX = 59; const int HR_MAX = 24; const int LEADING_ZERO_MIN = 10; const int HR_MIN_SPLITTER = 100;
class Course { private: std::string course_name_; std::string location_; std::string weekly_schedule_; int start_time_; int end_time_;
public:
Course() : course_name_("CPSC 131"), location_("EC 109"), weekly_schedule_("MW"), start_time_(1600), end_time_(1715) { }
Course(std::string name, std::string place, std::string days, int firstTime, int lastTime) : course_name_(name), location_(place), weekly_schedule_(days), start_time_(firstTime), end_time_(lastTime) { }
std::string course_name() const { return course_name_; } std::string location() const { return location_; } std::string weekly_schedule() const { return weekly_schedule_; } int start_time() const { return start_time_; }; int end_time() const { return end_time_; }
void set_course_name(const std::string & course_name) {course_name_ = course_name;} void set_location(const std::string & location) { location_ = location; } void set_weekly_schedule(const std::string & weekly_schedule) {weekly_schedule_ = weekly_schedule; } void set_start_time(int start_time) { start_time_ = start_time; } void set_end_time(int end_time) { end_time_ = end_time; }
void display() const; }; void display_courses(Course *courses[], int size); bool load_schedule(const std::string & filename, Course *ptr[], int & size);
class TimeSpan { private: double start_time_; double end_time_;
public: void set_start_time(int start_time) { start_time_ = start_time; }
int start_time() { return start_time_; }
void set_end_time (int end_time) { end_time_ = end_time; }
int end_time() { return end_time_; }
bool operator> (const TimeSpan & other) {
return(start_time_ > other.end_time_); }
bool operator
return(end_time_
bool has_conflict(TimeSpan temp, TimeSpan appointments[], int num_appointments);
main.cpp
#include #include #include #include #include "scheduler.hpp"
int main() { std::cout
const int SIZE = 100; Course *ptr[SIZE]; int num = 0;
std::string filename;
std::cout > filename;
bool good = load_schedule(filename, ptr, num);
if(good == true) { display_courses(ptr, num); }
for (int i = 0; i
}
Language is C++
code from milestone 4 needs to be modified to implement them as member functions and data members in the classes outlined in the readme. With the code given it needs to be modified based on the instructions given in the photos. Thank you.
README.md TuffyScheduler Milestone 5 This is milestone 5 of the TuffyScheduler Project. Requirements In this milestone we will refactor our code by creating a Course Schedule object to manage the creation and management of dynamically allocated Course objects representing your schedule. Refactor means reorganizing the structure of your code to make it more readable, adaptable, and/or scalable while keeping its intended properties. Classes TimeSpan Summary: Provide constructors for the TimeSpan class to make them easier to use. This allows you to use constructors instead of mutators when initializing your objects. Default constructor Create a default constructor that set both the start and end time of the object to 8000. Non-default constructor Create a non-default constructor that accepts two parameters: a start time and end time. Use an initialization list to assign the object's start and end time data members using the parameters provided. display Create a function called display that prints on-screen information about the object's start and end time. It should not take any parameters nor return any values. Below is an example of what your display function might display. Take note that it should display the time in military format. Start time: 9:00 End time: 13:30 Course Summary: Replace the integer start and end time data members with a single TimeSpan object. Using a TimeSpan data member implements object composition. Your code should now be easier to manage as all time-related functionalities are already implemented in the TimeSpan object and are only called by member functions inside Course Data members Replace the start time and end time integers with a single TimeSpan object, time. Default Constructor Create a default constructor that sets course_name, location, and weekly schedule to an empty string(""). Set time to a TimeSpan object. *Hint: You can create an unnamed object by using the class name followedy by (). For example, Rectangle() will create a Rectangle object using its default constructor NonDefault Constructor Create a nondefault constructor that takes in four parameters: course name, location, weekly schedule, and a time span. It should set the parameters' values to the corresponding data members. Accessors and mutators Remove the accessors and mutators for start and end time, and replace them with an accessor and mutator for the time, data member. display Update your display member function so that it still prints information about the Course. However, it now calls the appropriate member function provided by the TimeSpan class. intersects Create a function called intersects that accepts a Course pointer as a parameter and returns a boolean value. The function will compare the Course object against the other Course object referenced by the pointer (this was passed as a parameter). Course objects intersect when they are scheduled on the same day and their start and end times intersect. You can use the same logic from Milestone 4, but also consider the weekly schedule. Course Schedule Summary: Create a new class called Course Schedule that wil manage your dynamically allocated Course objects. Data members Create an array of Course pointers caled courses that has a maximum capacity of 100 elements. This is the same data type you used in the main function from Milestone 4. Create an integer data member called num_courses that will keep track of the total number of Courses added to courses Default constructor Create a default constructor that initializes num_courses_to O. This indicates that there are no Course objects in the array when a Course Schedule object is created. Accessors and mutators Create an accessor for the num_courses_ data member. There is no need to create accessors or mutators for other data members. has_conflict Create a private member function called has conflict. It should accept a Course pointer as a parameter and return a boolean value. The function should dereference and compare the Course object passed as a parameter against each Course object referenced in the course_array. If the passed Course object intersects with at least one of those objects, then the function should return true. Otherwise, it should return false. add Create a member function called add that accepts five parameters that describe a course: course name, course location, day of the week, start time, and end time. This function needs to dynamically allocate a Course object in the heap. Then, assign the values from the function's parameters to the corresponding data member of the object. Call the has conflict function to check if the newly created Course object conflicts with any of the Courses inside courses. If there are no conflicts, then add the newly created Course object to the course_array and return true. However, if it intersects with any of the Course objects in the course array, remove the newly created Course object from the heap and return false. display Create a display member function that does not accept any parameter or return any value. The function goes through each Course object referenced in courses and displays information about the object on the screen. Use the appropriate member function(s) to print information about the object on the screen. Destructor Create a destructor that will remove (de allocate) all Course objects that were dynamically allocated in the heap. load_schedule Modify your load_schedule global function to accept two parameters: a filename and a reference to a CourseSchedule object. The Course Schedule parameter is passed by reference so that any changes will be reflected back to whoever calls the load_schedule function. The function should behave the same way as in Milestone 4. However, it should use the add member function of the passed Course Schedule object instead. Use the appropriate variables as arguments to the add function. Take note that this new code will replace the implementation from Milestone 4 where we dynamically created and added Course objects to an array main function (Driver) Summary: Modify your main function to use a Course Schedule object instead of manually manipulating your array of Course pointers. Replace your array of Course pointers with a single CourseSchedule object. Modify the call to load schedule so that it passes the filename and the Course Schedule object as arguments Call the appropriate Course Schedule member function to display information about all Courses that were loaded. Removed functions The following functions will no longer be used as they are refactored into our classes. Feel free to remove them. display_courses: refactored as the Course Schedule's display member function has conflict: refactored as the Course Schedule's has_conflict member function Your program should behave the same way as it did in Milestone 4. Sample output Below are samples of the program's output given different cases. Valid file Welcome to Tuffy Scheduler! Please enter the file name containing the list of classes: sched.txt Schedule file loaded. Displaying contents ... Course name: CPSC 131 Location: EC 109 Weekly schedule: MW Start time: 16:00 End time: 17:15 Course name: CPSC 481 Location: CS 408 Weekly schedule: MW Start time: 16:00 End time: 17:15 Course name: CPSC 362 Location: CS 101 Weekly schedule: MW Start time: 8:00 End time: 9:50 Thank you for using Tuffy Scheduler. Start time happens after end time Welcome to Tuffy Scheduler! Please enter the file name containing the list of classes: sched.txt Error: The start time 17:15 should happen before the end time 16:00. Invalid file. Thank you for using Tuffy Scheduler. Invalid weekly schedule Welcome to Tuffy Scheduler! Please enter the file name containing the list of classes: sched.txt Error: Invalid weekly schedule symbol MX. Invalid file. Thank you for using Tuffy Scheduler. README.md TuffyScheduler Milestone 5 This is milestone 5 of the TuffyScheduler Project. Requirements In this milestone we will refactor our code by creating a Course Schedule object to manage the creation and management of dynamically allocated Course objects representing your schedule. Refactor means reorganizing the structure of your code to make it more readable, adaptable, and/or scalable while keeping its intended properties. Classes TimeSpan Summary: Provide constructors for the TimeSpan class to make them easier to use. This allows you to use constructors instead of mutators when initializing your objects. Default constructor Create a default constructor that set both the start and end time of the object to 8000. Non-default constructor Create a non-default constructor that accepts two parameters: a start time and end time. Use an initialization list to assign the object's start and end time data members using the parameters provided. display Create a function called display that prints on-screen information about the object's start and end time. It should not take any parameters nor return any values. Below is an example of what your display function might display. Take note that it should display the time in military format. Start time: 9:00 End time: 13:30 Course Summary: Replace the integer start and end time data members with a single TimeSpan object. Using a TimeSpan data member implements object composition. Your code should now be easier to manage as all time-related functionalities are already implemented in the TimeSpan object and are only called by member functions inside Course Data members Replace the start time and end time integers with a single TimeSpan object, time. Default Constructor Create a default constructor that sets course_name, location, and weekly schedule to an empty string(""). Set time to a TimeSpan object. *Hint: You can create an unnamed object by using the class name followedy by (). For example, Rectangle() will create a Rectangle object using its default constructor NonDefault Constructor Create a nondefault constructor that takes in four parameters: course name, location, weekly schedule, and a time span. It should set the parameters' values to the corresponding data members. Accessors and mutators Remove the accessors and mutators for start and end time, and replace them with an accessor and mutator for the time, data member. display Update your display member function so that it still prints information about the Course. However, it now calls the appropriate member function provided by the TimeSpan class. intersects Create a function called intersects that accepts a Course pointer as a parameter and returns a boolean value. The function will compare the Course object against the other Course object referenced by the pointer (this was passed as a parameter). Course objects intersect when they are scheduled on the same day and their start and end times intersect. You can use the same logic from Milestone 4, but also consider the weekly schedule. Course Schedule Summary: Create a new class called Course Schedule that wil manage your dynamically allocated Course objects. Data members Create an array of Course pointers caled courses that has a maximum capacity of 100 elements. This is the same data type you used in the main function from Milestone 4. Create an integer data member called num_courses that will keep track of the total number of Courses added to courses Default constructor Create a default constructor that initializes num_courses_to O. This indicates that there are no Course objects in the array when a Course Schedule object is created. Accessors and mutators Create an accessor for the num_courses_ data member. There is no need to create accessors or mutators for other data members. has_conflict Create a private member function called has conflict. It should accept a Course pointer as a parameter and return a boolean value. The function should dereference and compare the Course object passed as a parameter against each Course object referenced in the course_array. If the passed Course object intersects with at least one of those objects, then the function should return true. Otherwise, it should return false. add Create a member function called add that accepts five parameters that describe a course: course name, course location, day of the week, start time, and end time. This function needs to dynamically allocate a Course object in the heap. Then, assign the values from the function's parameters to the corresponding data member of the object. Call the has conflict function to check if the newly created Course object conflicts with any of the Courses inside courses. If there are no conflicts, then add the newly created Course object to the course_array and return true. However, if it intersects with any of the Course objects in the course array, remove the newly created Course object from the heap and return false. display Create a display member function that does not accept any parameter or return any value. The function goes through each Course object referenced in courses and displays information about the object on the screen. Use the appropriate member function(s) to print information about the object on the screen. Destructor Create a destructor that will remove (de allocate) all Course objects that were dynamically allocated in the heap. load_schedule Modify your load_schedule global function to accept two parameters: a filename and a reference to a CourseSchedule object. The Course Schedule parameter is passed by reference so that any changes will be reflected back to whoever calls the load_schedule function. The function should behave the same way as in Milestone 4. However, it should use the add member function of the passed Course Schedule object instead. Use the appropriate variables as arguments to the add function. Take note that this new code will replace the implementation from Milestone 4 where we dynamically created and added Course objects to an array main function (Driver) Summary: Modify your main function to use a Course Schedule object instead of manually manipulating your array of Course pointers. Replace your array of Course pointers with a single CourseSchedule object. Modify the call to load schedule so that it passes the filename and the Course Schedule object as arguments Call the appropriate Course Schedule member function to display information about all Courses that were loaded. Removed functions The following functions will no longer be used as they are refactored into our classes. Feel free to remove them. display_courses: refactored as the Course Schedule's display member function has conflict: refactored as the Course Schedule's has_conflict member function Your program should behave the same way as it did in Milestone 4. Sample output Below are samples of the program's output given different cases. Valid file Welcome to Tuffy Scheduler! Please enter the file name containing the list of classes: sched.txt Schedule file loaded. Displaying contents ... Course name: CPSC 131 Location: EC 109 Weekly schedule: MW Start time: 16:00 End time: 17:15 Course name: CPSC 481 Location: CS 408 Weekly schedule: MW Start time: 16:00 End time: 17:15 Course name: CPSC 362 Location: CS 101 Weekly schedule: MW Start time: 8:00 End time: 9:50 Thank you for using Tuffy Scheduler. Start time happens after end time Welcome to Tuffy Scheduler! Please enter the file name containing the list of classes: sched.txt Error: The start time 17:15 should happen before the end time 16:00. Invalid file. Thank you for using Tuffy Scheduler. Invalid weekly schedule Welcome to Tuffy Scheduler! Please enter the file name containing the list of classes: sched.txt Error: Invalid weekly schedule symbol MX. Invalid file. Thank you for using Tuffy SchedulerStep by Step Solution
There are 3 Steps involved in it
Step: 1
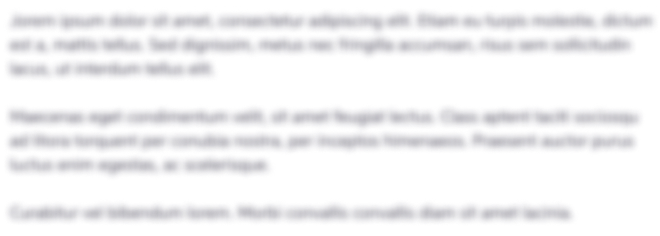
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started