Question
Mobile Service Provider A mobile phone service provider has three different data plans for its customers: Package A: For $39.99 per month, 4 gigabytes are
Mobile Service Provider
A mobile phone service provider has three different data plans for its customers: Package A: For $39.99 per month, 4 gigabytes are provided. Additional data costs $10 per gigabyte. Package B: For $59.99 per month, 8 gigabytes are provided. Additional data costs $5 per gigabyte. Package C: For $69.99 per month, unlimited data is provided. Write a program that calculates a customer's monthly bill. It should ask which package the customer has purchased and how many gigabytes were used. It should then display the total amount due. Input Validation: Be sure the user only selects package A, B, or C.
use the included .cpp file and add it your solution file. Include the following modifications. Declare constants to store base prices for packages. For example, 39.99 for Package A, 59.99 for Package B, and 69.99 for Package C. Make sure the customer name and billing month is accepted. Billing month should be in the range 1-12. If it is not, please display an error message. Make sure a user enters only 'A', 'B' or 'C' for the plan package code. Display an error message if any other letter is entered. Only if a valid package code ('A','B', or 'C') is entered, then the user should be asked to enter the number of gigabytes used Display a bill with month of bill, customer name, package code, charges included in package deal, and charge for additional data. Make sure you use identifying labels too.
//Your Name //Include a brief description of your program //One fun thing you learned this week //What challenged you? #include#include #include using namespace std; int main() { //Decalre variables and constants char package = 'X'; int month= 0; string monthName = ""; cout <<" Welcome to Bytes at your Service"< > package; package = toupper(package); //to make sure that whatever the user entered is converted into upper case //Validate input (A, B, C) if (package != 'A' && package != 'B' && package != 'C') { cout <<"Sorry we do not have that package yet !!" < >month; //Validate for month if ( month < 1 || month > 12) { cout <<"Invalid month "; } else { //Once we have validated that it is a valid month, then we will accept the gigabytes // do the calculations and display the bill //Validate input 1-12 switch (month) { case 1: monthName = "January" ; break; case 2: monthName = "February"; break; case 3: monthName = "March"; break; case 4: monthName = "April"; break; case 5: monthName = "May"; break; case 6: monthName = "June"; break; //You can complete the rest of the Case branches case 12: monthName = "December"; break; } //Now accept gigabytes used and calculated charges according to package A, B or C //start calculations if A, B, or C was selected //Display Bill - Please make sure you include the correct variables in the following cout //statements to display the vaues with the labels cout << "*******Bytes at your Service********** " << endl << "Customer Name: " << endl << "Month of Bill: " << endl << "Package Code: " << endl << "Package Charge: $" << endl << "Additional Data Charge: $" << showpoint << fixed << setprecision(2) << endl << "Total Charge: $" << showpoint << fixed << setprecision(2) << endl << "****************************************" << endl; }//end of the else block of month }//end of the else block of package type system("pause"); return 0; }
Grading criteria:
Template used for program and file name as specified.
Variables defined for name, month, package, and charges for billing.
Constants for base prices.
Steps to accept input for name, month, package. All validations included. Error messages displayed when needed.
Gigabytes accepted only with valid package.
Calculations included for additional charges.
Display a bill with month of bill, customer name, package code, charges included in package deal, and charge for additional data. Identifying labels included too.
Documentation Comments, Good identifier names etc.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
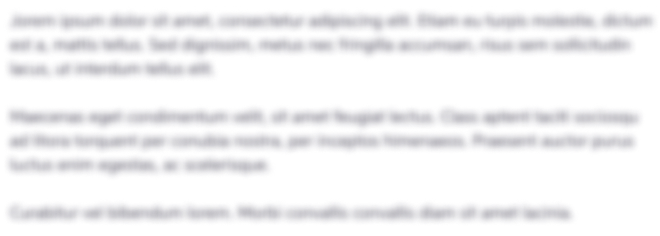
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started