Question
Modify a JAVA program that opens the salesdat.txt file and processes it contents. Provide a user-friendly interface (Console based). The program should display the following:
Modify a JAVA program that opens the salesdat.txt file and processes it contents. Provide a user-friendly interface (Console based). The program should display the following:
Start with and modify the provided source code
Prompt the user to select a store (1-6)
Prompt the user to select one of the below options (a -g)
a - The total sales for each week. (Should print 5 values - one for each week).
b - The average daily sales for each week. (Should print 5 values - one for each week).
c - The total sales for all the weeks. (Should print 1 value)
d - The average weekly sales. (Should print 1 value)
e - The week with the highest amount in sales. (Should print 1 week #)
f - The week with the lowest amount in sales. (Should print 1 week #)
g - all of the above
The file contains the dollars amount of sales that a retail store made each day for a number of weeks. Each line in the file contains thirty five numbers, which are sales numbers for five weeks. The number are separated by space. Each line in the file represents a separate store.
Also please make:
a. Add a class diagram with your submission.
b. Add comments to your code in FileIO class.
c. Make sure you adequately test your code.
d. Provide a user-friendly interface (Console based).
_____________________________________________________________________________________________________________________________________
public class Store
{
private float salesbyweek[][];
Store() //Algorithms belong in store.java
{
salesbyweek = new float[5][7];
}
// getter and setters
// setsaleforweekdayintersection(int week, int day, float sale)
public void setsaleforweekdayintersection(int week, int day, float sale)
{
salesbyweek[week][day] = sale;
}
public void printdata()
{
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 7; j++)
{
System.out.print(salesbyweek[i][j] + " ");
}
System.out.println("");
}
}
// float [] getsalesforentireweek(int week)
// float getsaleforweekdayintersection(int week, int day)
// businessmethod - instance variables
// a. totalsalesforweek (float[] <--instance variables
// b. avgsalesforweek (float[] <--instance variables
// c. totalsalesforallweeks - float - use a <--instance variables
// d. averageweeklysales - float - use b <--instance variables
// e. weekwithhighestsaleamt - float - use a <--instance variables
// f. weekwithlowestsaleamt - float - use a <--instance variables
// analyzeresults //call a through f or all of the above
// print() - prints - well formated - uses printf - prints only the things user wants
}
______________________________________________________________________________________________________________________________________
public class Franchise
{
//array of stores
private Store stores[];
public Franchise(int num)
{
stores = new Store[num];
}
public Store getStores(int i)
{
return stores[i];
}
public void setStores(Store stores, int i)
{
this.stores[i] = stores;
}
public int numberofstores()
{
return stores.length;
}
public void calc()
{
//for (int i=0; i //stores[i].analyze(); } } _______________________________________________________________________________________________________________________________________ import java.io.*; import java.util.*; public class FileIO { private String fname = null; private boolean DEBUG = false; //constructor public FileIO(String fname) { this.fname = fname; } public Franchise readData() { Franchise a1 = null; //counter to count the number of lines int counter = 0; //attempts this block of code, if something goes wrong catch an exception try { //opens a text file FileReader file = new FileReader(fname); //takes the text file and links file to a buffer BufferedReader buff = new BufferedReader(file); String temp; boolean eof = false; while (!eof) { String line = buff.readLine(); counter++; //if line equals null it is the end of the file if (line == null) eof = true; else { if (DEBUG) System.out.println("Reading" + line); if (counter == 1) { //puts the line in temp temp = line; a1 = new Franchise(Integer.parseInt(temp)); if (DEBUG) System.out.println("d " + a1.numberofstores()); } if (counter == 2) ; if (counter > 2) { int x = buildStore(a1, (counter-3), line); if (DEBUG) System.out.println("Reading Store # "+(counter-2)+" " + "Number of weeks read = " + x); if (DEBUG) { System.out.println("Data read:"); a1.getStores(counter-3).printdata(); } } } } buff.close(); } catch (Exception e) //if code does not work catch exception { //prints error message System.out.println("Error -- " + e.toString()); } return a1; } public int buildStore(Franchise a1, int counter, String temp) { //Builds a new store Store tstore = new Store(); String s1 = ""; float sale = 0.0f; int week = 0; int day = 0; StringTokenizer st = new StringTokenizer(temp); //loop to read 7 days of data, stops reading at 7 days while (st.hasMoreTokens()) { for(day=0;day<7;day++) { s1 = st.nextToken(); sale = Float.parseFloat(s1); //sets the end of week by value tstore.setsaleforweekdayintersection(week, day, sale); } week++; } a1.setStores(tstore, counter); return week; } } _______________________________________________________________________________________________________________________________________ public class Driver { public static void main(String[] args) { // TODOAuto-generated method stub FileIO a1 = new FileIO("C:\\Users\\jayadams66\\OneDrive\\Documents\\School\\" + "CURRENT QUARTER\\Winter 2018\\Java 35A\\Assignments\\Assignment 3\\Salesdat.txt"); Franchise f = a1.readData(); //precalculating f.calc(); } } _________________________________________________________________________________________________ SALESDAT.TXT 6 Day1 Day2 Day3 Day4 Day5 Day6 Day7 Day8 Day9 Day10 Day11 Day12 Day13 Day14 Day15 Day16 Day17 Day18 Day19 Day20 Day21 Day22 Day23 Day24 Day25 Day26 Day27 Day28 Day29 Day30 Day31 Day32 Day33 Day34 Day35 2541.56 2258.96 2214 2256 2154 2398 2597 2684 2771 2858 2945 3032 3119 3206 3293 3380 3467 3554 3641 3728 3815 3902 3989 4076 4163 4250 4337 4424 4511 4598 4685 4772 4859 4946 5033 2041.56 1758.96 1714 1756 1654 1898 2097 2184 2271 2358 2445 2532 2619 2706 2793 2880 2967 3054 3141 3228 3315 3402 3489 3576 3663 3750 3837 3924 4011 4098 4185 4272 4359 4446 4533 3041.56 2758.96 2714 2756 2654 2898 3097 3184 3271 3358 3445 3532 3619 3706 3793 3880 3967 4054 4141 4228 4315 4402 4489 4576 4663 4750 4837 4924 5011 5098 5185 5272 5359 5446 5533 3563.54 3280.94 3235.98 3277.98 3175.98 3419.98 3618.98 3705.98 3792.98 3879.98 3966.98 4053.98 4140.98 4227.98 4314.98 4401.98 4488.98 4575.98 4662.98 4749.98 4836.98 4923.98 5010.98 5097.98 5184.98 5271.98 5358.98 5445.98 5532.98 5619.98 5706.98 5793.98 5880.98 5967.98 6054.98 2547.21 2264.61 2219.65 2261.65 2159.65 2403.65 2602.65 2689.65 2776.65 2863.65 2950.65 3037.65 3124.65 3211.65 3298.65 3385.65 3472.65 3559.65 3646.65 3733.65 3820.65 3907.65 3994.65 4081.65 4168.65 4255.65 4342.65 4429.65 4516.65 4603.65 4690.65 4777.65 4864.65 4951.65 5038.65 4040.55 3757.95 3712.99 3754.99 3652.99 3896.99 4095.99 4182.99 4269.99 4356.99 4443.99 4530.99 4617.99 4704.99 4791.99 4878.99 4965.99 5052.99 5139.99 5226.99 5313.99 5400.99 5487.99 5574.99 5661.99 5748.99 5835.99 5922.99 6009.99 6096.99 6183.99 6270.99 6357.99 6444.99 6531.99
Step by Step Solution
There are 3 Steps involved in it
Step: 1
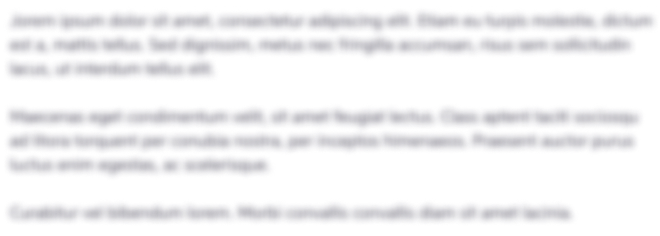
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started