Question
Modify a provided generic linked list class and analyze the performance of your new functionality. Do the following: 1. Modify the class as appropriate so
Modify a provided generic linked list class and analyze the performance of your new functionality.
Do the following:
1. Modify the class as appropriate so that add(T value) performs in constant time. Do not make the list into a doubly linked list.
2. Add a remove() method that removes the last value in the list.
3. Add a remove(int index) method that removes the value at position index, and throws an exception if index is invalid.
4. Add a remove() method to the ForwardIterator. Look at the java.util.Iterator javadocs for information about how this method should function.
5. Create a new ReverseIterator and a method that returns one that visits list elements in reverse order. Because this is a singly linked list, this iterator will perform poorly. That is desirable for our purposes.
6. Write a Tester1 class that demonstrates that your modifications function correctly. Simply test add(T value), remove() and remove(int index). Make sure that size() returns the correct value after adds and removes. Make sure the forward iterator still works, including your new remove() method. Make sure your new reverse iterator works correctly.
7. Write a Tester2 class that shows that your new add(T value) method operates in constant time, rather than linear as in the provided code. Show that your reverse iterator operates in quadratic time.
Code provided:
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
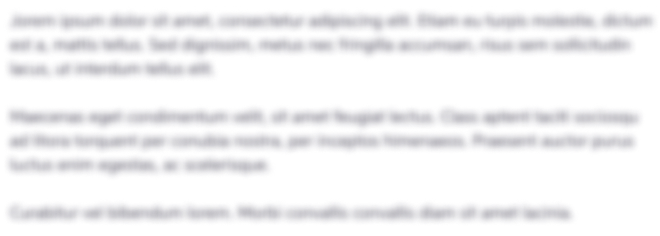
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started