Question
modify code based on Instructor's Feedback Instructor's Feedback * poor Id(s) rec, * < < not needed between strings * excessive use of print/cout/cin/ file
modify code based on Instructor's Feedback
Instructor's Feedback * poor Id(s) rec, * << not needed between strings * excessive use of print/cout/cin/ file stream/stringstream and/or endl and/or << >> * const * printInvalidCarRecords () unnessesary complex code, can read from a file using a simple loop with one cout statement while (getline(errorFile, line)) cout<
My code:
#include
const int MAX_ID_LENGTH = 9;
// structure containing carID, model, quantity and price typedef struct { string carID; string model; string quantity; string price; } CarRecord;
// Function Prototypes bool isValidCarRecord(CarRecord car); void getData(CarRecord inventory[100], int *size); void printCarInventory(CarRecord inventory[100], int size, bool valid); int printMenu(); void printInvalidCarRecords(string fileName, CarRecord inventory[100], int size, bool valid); void invalidCarRecords(string fileName, CarRecord inventory[100], int size);
int main() { bool valid; // array of structure CarRecord CarRecord inventory[100]; int size = 0; int choice = 1; // reading file and storing in array getData(inventory, &size); // continue untill user chooses to exit
while (choice != 3) { choice = printMenu();
switch (choice) { case 1: // for case 1 print inventory printCarInventory(inventory, size, valid); break; case 2: // for case 2 print invalid inventory printInvalidCarRecords("error.txt", inventory, size, valid); break; case 3: cout << "Goodbye"; break; default: // printing message for invalid input cout << "Invalid Input. Please try again. "; break; } } }
// Function to check if a car record is valid bool isValidCarRecord(CarRecord car) { if (car.carID.length() != MAX_ID_LENGTH) { return false; } if (!isdigit(car.carID[0]) || !isdigit(car.carID[1])) { return false; } for (int i = 2; i < 6; i++) { if (!isalpha(car.carID[i])) { return false; } if (car.carID[i] < 'A' || car.carID[i] > 'S' || car.carID[i] == 'O') { return false; } } for (int i = 6; i < MAX_ID_LENGTH; i++) { if (!isalnum(car.carID[i])) { return false; } } return true; }
// reading file void getData(CarRecord inventory[100], int *size) { ifstream file("carInventory.txt"); ofstream errorFile("error.txt"); if (file.is_open()) { string line; while (getline(file, line)) { size_t pos = 0; string token; CarRecord temp; pos = line.find(" "); token = line.substr(0, pos); temp.carID = token; if (!isValidCarRecord(temp)) { errorFile << line << endl; continue; } line.erase(0, pos + 1); pos = line.find(" "); token = line.substr(0, pos); temp.model = token; line.erase(0, pos + 1); pos = line.find(" "); token = line.substr(0, pos); temp.quantity = token; line.erase(0, pos + 1); temp.price = line; inventory[*size] = temp; *size = *size + 1; } file.close(); errorFile.close(); } else { cout << "Cannot open file. "; EXIT_SUCCESS; } }
// printing inventory void printCarInventory(CarRecord inventory[100], int size, bool valid) { if (valid == false) { cout << left << setw(10) << "Car ID" << setw(20) << "Model" << right << setw(10) << "Quantity" << setw(10) << "Price" << endl; cout << left << setw(9) << setfill('-') << "-" << setw(20) << setfill('-') << "-" << right << setw(10) << setfill('-') << "-" << setw(10) << setfill('-') << "-" << endl; cout << setfill(' ');
for (int i = 0; i < size; i++) { cout << left << setw(9) << inventory[i].carID << "\t" << setw(15) << inventory[i].model << "\t" << right << setw(10) << inventory[i].quantity << "\t" << fixed << setprecision(2) << setw(10) << inventory[i].price << endl; cout << setprecision(6); }
cout << left << setw(9) << setfill('-') << "-" << setw(20) << setfill('-') << "-" << right << setw(10) << setfill('-') << "-" << setw(10) << setfill('-') << "-" << endl; cout << setfill(' '); } }
int printMenu() { // choice variable int choice = 3; // showing Menu cout << " Please choose : "; cout << "1) Print Inventory "; cout << "2) print invalid records from an error file "; cout << "3) Exit "; // taking choice cin >> choice; // take choice again for invalid input
while (choice < 1 || choice > 3) { cout << "Please choose valid input: "; cin >> choice; } // clearing cin cin.clear(); cin.ignore(); return choice; }
void printInvalidCarRecords( string fileName, CarRecord inventory[100], int size, bool valid) { ifstream errorFile("error.txt"); if (errorFile.is_open()) { string line; while (getline(errorFile, line)) { size_t pos = 0; string token; CarRecord temp; pos = line.find(" "); token = line.substr(0, pos); temp.carID = token; if (!isValidCarRecord(temp)) { cout << " Invalid" << endl; cout << "-----------------------------------" << endl; cout << line << endl; } }
errorFile.close(); } } void invalidCarRecords(string fileName, CarRecord inventory[100], int size) { ofstream fout; fout.open("error.txt", ios::app); for (int i = 0; i < size; i++) { fout << inventory[i].carID << "\t" << inventory[i].model << "\t" << inventory[i].quantity << "\t" << inventory[i].price << endl; }
fout.close(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
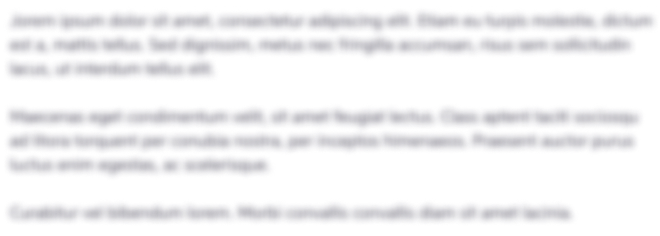
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started