Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Modify the adjacency matrix implementation to use an extra stack where you push a new node id onto the stack when adding a node to
Modify the adjacency matrix implementation to use an extra stack where you push a new node id onto the stack when adding a node to minimize the initialization effort. Do that by scanning the stack to see if a node id has already been plugged into the adj. matrix #include #include #include #include "Graph.hpp using namespace std; template class AdjMatrixGraph: public Graph private: class NodeEntry public: N node; int index; ; const static int maxSize ; bool adjMatrixmaxSizemaxSize; NodeEntry nodesmaxSize; int numNodes ; int findNodeInMatrixN x for int j; j numNodes; j if x nodesjnode return j; return ; public: Default constuctor, create empty AdjMatrixGraph forint i ; i maxSize; i for int j; j maxSize; j adjMatrixij false; Add the nodes in the list to graph AdjMatrixGraphvector newNodes, vector newEdges adjMatrix new NodeEntrymaxSizemaxSize; for typename vector::constiterator it newNodes.begin; it newNodes.end; it NodeEntry ne new NodeEntry; nenode it; neindex numNodes; nodesnumNodes ne; for typename vector::constiterator it newEdges.begin; it newEdges.end; it pair edge it; int sourceIndex findNodeInMatrixedgefirst; int destIndex findNodeInMatrixedgesecond; if sourceIndex if destIndex adjMatrixsourceIndexdestIndex true; Clean up behind ourselves ~AdjMatrixGraph; virtual bool adjacentN x N y bool result false; int xIndex findNodeInMatrixx; int yIndex findNodeInMatrixy; if xIndex && yIndex bool xy adjMatrixxIndexyIndex; bool yx adjMatrixyIndexxIndex; result xy && yx; returnresult; virtual vector neighborsN x vector v new vector; int xIndex findNodeInMatrixx; if xIndex for int i; i numNodes; i if adjMatrixxIndexi true vpushbacknodesinode; return v; virtual void addNodeN node NodeEntry ne new NodeEntry; nenode node; neindex numNodes; nodesnumNodes ne; numNodes; virtual void addEdgeN x N y int xIndex findNodeInMatrixx; int yIndex findNodeInMatrixy; if xIndex && yIndex adjMatrixxIndexyIndex true; virtual void deleteEdgeN x N y int xIndex findNodeInMatrixx; int yIndex findNodeInMatrixy; adjMatrixxIndexyIndex false; Traversals void dfsN startNode, std::function visit map visited; for int i ; i numNodes; i visitednodesinode false; stack s; spushstartNode; while sempty N currentNode stop; spop; bool beenVisited visitedcurrentNode; if beenVisited visitcurrentNode; visitedcurrentNode true; vector neighVec neighborscurrentNode; for auto neighbor: neighVec if visitedneighbor spushneighbor; void bfsN startNode, std::function visit map visited; for int i ; i numNodes; i visitednodesinode false; queue q; qpushstartNode; while qempty N currentNode qfront; qpop; bool beenVisited visitedcurrentNode; if beenVisited visitcurrentNode; visitedcurrentNode true; vector neighVec neighborscurrentNode; for auto neighbor: neighVec if visitedneighbor qp
Modify the adjacency matrix implementation to use an extra stack where you push a new node id onto the stack when adding a node to minimize the initialization effort. Do that by scanning the stack to see if a node id has already been plugged into the adj. matrix
#include
#include
#include
#include "Graph.hpp
using namespace std;
template
class AdjMatrixGraph: public Graph
private:
class NodeEntry
public:
N node;
int index;
;
const static int maxSize ;
bool adjMatrixmaxSizemaxSize;
NodeEntry nodesmaxSize;
int numNodes ;
int findNodeInMatrixN x
for int j; j numNodes; j
if x nodesjnode
return j;
return ;
public:
Default constuctor, create empty
AdjMatrixGraph
forint i ; i maxSize; i
for int j; j maxSize; j
adjMatrixij false;
Add the nodes in the list to graph
AdjMatrixGraphvector newNodes, vector newEdges
adjMatrix new NodeEntrymaxSizemaxSize;
for typename vector::constiterator it newNodes.begin;
it newNodes.end;
it
NodeEntry ne new NodeEntry;
nenode it;
neindex numNodes;
nodesnumNodes ne;
for typename vector::constiterator it newEdges.begin;
it newEdges.end;
it
pair edge it;
int sourceIndex findNodeInMatrixedgefirst;
int destIndex findNodeInMatrixedgesecond;
if sourceIndex
if destIndex
adjMatrixsourceIndexdestIndex true;
Clean up behind ourselves
~AdjMatrixGraph;
virtual bool adjacentN x N y
bool result false;
int xIndex findNodeInMatrixx;
int yIndex findNodeInMatrixy;
if xIndex && yIndex
bool xy adjMatrixxIndexyIndex;
bool yx adjMatrixyIndexxIndex;
result xy && yx;
returnresult;
virtual vector neighborsN x
vector v new vector;
int xIndex findNodeInMatrixx;
if xIndex
for int i; i numNodes; i
if adjMatrixxIndexi true
vpushbacknodesinode;
return v;
virtual void addNodeN node
NodeEntry ne new NodeEntry;
nenode node;
neindex numNodes;
nodesnumNodes ne;
numNodes;
virtual void addEdgeN x N y
int xIndex findNodeInMatrixx;
int yIndex findNodeInMatrixy;
if xIndex && yIndex
adjMatrixxIndexyIndex true;
virtual void deleteEdgeN x N y
int xIndex findNodeInMatrixx;
int yIndex findNodeInMatrixy;
adjMatrixxIndexyIndex false;
Traversals
void dfsN startNode, std::function visit
map visited;
for int i ; i numNodes; i
visitednodesinode false;
stack s;
spushstartNode;
while sempty
N currentNode stop;
spop;
bool beenVisited visitedcurrentNode;
if beenVisited
visitcurrentNode;
visitedcurrentNode true;
vector neighVec neighborscurrentNode;
for auto neighbor: neighVec
if visitedneighbor spushneighbor;
void bfsN startNode, std::function visit
map visited;
for int i ; i numNodes; i
visitednodesinode false;
queue q;
qpushstartNode;
while qempty
N currentNode qfront;
qpop;
bool beenVisited visitedcurrentNode;
if beenVisited
visitcurrentNode;
visitedcurrentNode true;
vector neighVec neighborscurrentNode;
for auto neighbor: neighVec
if visitedneighbor qp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
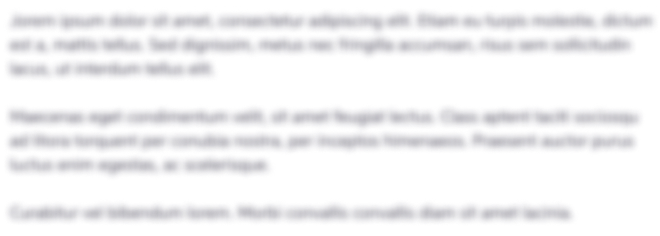
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started