Question
modify the code to where determining the winner is moved to the main () function. # Deals a card. Values range from 1 to 10
modify the code to where determining the winner is moved to the main () function.
# Deals a card. Values range from 1 to 10
def deal_card():
return random.randint(1, 10)
# Handles the player's turn. Returns the point value for the player's hand.
def get_player_score():
# Get the player's score.
player_val = deal_card()
# Get dealer's score.
dealer_val = deal_card()
# Get the player's score.
player_val += deal_card()
# Displays the sum of the first two cards to the player.
print("The sum of the first two cards is:", player_val)
# Checks if the player wants to take another card.
# "while True" will make it loop forever :)
while True:
user_response = input("Do you want to take another card? (Y/N): ")
# upper() method converts all lowercase characters in a string into uppercase characters and returns it
if user_response.upper() == "Y":
# Deals another card to the player and adds it to their score.
player_val += deal_card()
# Displays the updated score to the player.
print("Your hand now has a total value of:", player_val)
if player_val > 21:
# The player has gone bust.
print("BUST! Your score is:", player_val)
print("Your score is over 21. The dealer wins.")
print("** You lose. **")
return player_val, dealer_val
#upper() method converts all lowercase characters in a string into uppercase characters and returns it
elif user_response.upper() == "N":
break
else:
# Validation
print("Invalid input. Please enter Y or N.")
# Get the dealer's score.
while dealer_val < 17:
dealer_val += deal_card()
# Display the final scores to the player.
print("You have stopped taking more cards with a hand value of: ", player_val)
print("The dealer's final score is:", dealer_val)
# Determine the winner.
if dealer_val > 21 or player_val > dealer_val:
print("Congratulations! You win!")
elif dealer_val > player_val:
print("** You lose. **")
# If user and the dealer have the same value of cards...ask user to have a chance to hit again
elif dealer_val == player_val:
# "while True" will make it loop forever :)
while True:
user_response = input("Your hand value is the same as the dealer's. Do you want to hit again? (Y/N): ")
if user_response.upper() == "Y":
player_val += deal_card()
print("Your hand now has a total value of:", player_val)
if player_val > 21:
print("BUST! Your score is:", player_val)
print("Your score is over 21. The dealer wins.")
print("** You lose. **")
return player_val, dealer_val
elif player_val == dealer_val:
print("Your hand value is the same as the dealer's. You can hit again.")
continue
else:
break
elif user_response.upper() == "N":
break
else:
print("Invalid input. Please enter Y or N.")
# The main function. It repeatedly plays games of blackjack until the user decides to stop.
def main():
# Prime the loop and start the first game.
user_response = "Y"
#upper() method converts all lowercase characters in a string into uppercase characters and returns it
while user_response.upper() == "Y":
# Get scores of the player and dealer.
get_player_score()
# Ask user whether they want to play another game.
user_response = input("Do you want to play another game? (Y/N): ")
# Call the main function to start the blackjack program.
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Your code looks almost complete but there are a few changes needed to properly determine the winner within the main function You need to capture the r...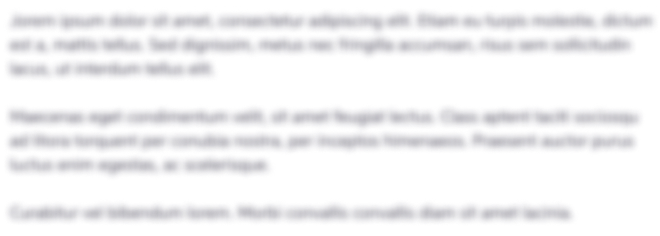
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started