Question
Modify the following code to use a dynamic array for the followers, so that a user can have any number of followers. Every time, we
Modify the following code to use a dynamic array for the followers, so that a user can have any number of followers. Every time, we need to increase the size of the array, we will double the size.
This requires the following changes to be done to the Twitter class declaration. followers was declared as:
DT followers[5]
This should be changed to DT * followers
We also have to add one more data member for the Twitter class, called capacity, which is the current size of the dynamic array. During initialization (i.e., the constructor), we can start with an array of size 2.
You further need to modify AddFollowers function so that the size of the array is increased if needed.
#ifndef TWITTERHEADER_H_INCLUDED #define TWITTERHEADER_H_INCLUDED
#include #include
using namespace std;
template class Twitter { public: Twitter(DT u); void AddFollower(DT p); void RemoveFollower(DT p); void PrintFollowers(); private: DT user; int numFollowers; DT followers[5]; };
template Twitter
::Twitter(DT u) { user = u; numFollowers = 0; }
template void Twitter
::AddFollower(DT p) { if (numFollowers == 5) return; followers[numFollowers++] = p; // add p to the followers at index numFollowers and increment numFollowers }
template void Twitter
::RemoveFollower(DT p) { int index = -1; for (int i = 0; i < numFollowers; i++) { if (followers[i] == p) { index = i; } } for (int i = index; i < numFollowers - 1; i++) { followers[i] = followers[i + 1]; } numFollowers --; }
template void Twitter
::PrintFollowers() { for (int i = 0; i < numFollowers; i++) { cout << followers[i] << ", "; } cout << endl; }
#endif // TWITTERHEADER_H_INCLUDED
#include #include #include "TwitterHeader.h"
using namespace std;
struct Profile { string userName; int age; string state;
bool operator == (Profile p2) { if (p2.userName == userName) return true; else return false; } };
ostream& operator << (ostream & output, Profile p) { output << p.userName; return output; }
int main() { Twitter user1 ("P1"); user1.AddFollower("P2"); user1.PrintFollowers(); user1.AddFollower("P3"); user1.PrintFollowers(); user1.RemoveFollower("P2"); user1.PrintFollowers();
Profile up; up.userName = "UP1"; Twitter user2 (up); up.userName = "UP2"; user2.AddFollower(up); user2.PrintFollowers(); up.userName = "UP3"; user2.AddFollower(up); user2.PrintFollowers(); up.userName = "UP2"; user2.RemoveFollower(up); user2.PrintFollowers(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
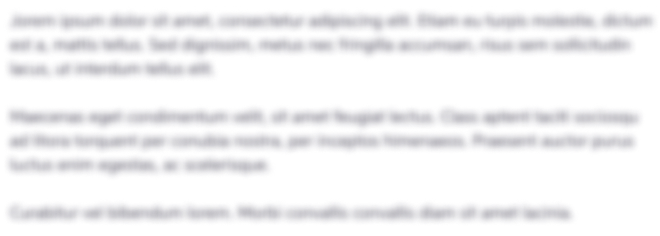
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started