Question
Modify the program to add a function to compute and display the total sales for all the concerts. Support your experimentation with screen captures of
Modify the program to add a function to compute and display the total sales for all the concerts. Support your experimentation with screen captures of executing the new code.
C Code
The following is the C Code that will compile in execute in the online compilers.
// C code // This code will compute the values of the sales ticket sales for concerts // and sort the entries by those values // Developer: Faculty CMIS102 // Date: Jan 31, XXXX
#include
#define MAXN 100 // max characters in a group/concert name #define MAXG 50 // max concerts/groups #define MAXC 3 // max categories
char group [MAXG][MAXN]; int fans [MAXG][MAXC]; float prices [MAXC]; float sales [MAXG];
int count = 0;
void printArray () { printf ("%15s%5s%5s%5s%10s ", "Concert", "s1", "s2", "s3", "Sales"); for (int i = 0; i < count; i++) {
printf ("%15s", group [i]); for (int j = 0; j < MAXC; j++) {
printf ("%5d", fans[i][j]); } // end for each category printf ("%10.2f ", sales [i]);
} // end for each group } // end function printArray
void computeSales () { for (int i = 0; i < count; i++) {
sales [i] = 0; for (int j = 0; j < MAXC; j++) {
sales [i] += prices [j] * fans [i][j]; } // end for each category
} // end for each group } // end function computeSales
void switchRows (int m, int n) { char tc;
int ti; float v; // printf ("Switching %d with %d ", m, n); for (int i = 0; i < MAXN; i++) {
tc = group [m][i]; group [m][i] = group [n][i]; group [n][i] = tc;
} // end for each character in a group name for (int i = 0; i < MAXC; i++) {
ti = fans [m][i]; fans [m][i] = fans [n][i]; fans [n][i] = ti;
Page 9 of 12
} // end for each fan category v = sales [m]; sales [m] = sales [n]; sales [n] = v;
} // end switch
int findMinSales (int m) { float min = sales [m]; int target = m; for (int i = m+1; i < count; i++)
if (sales [i] < min) { min = sales [i]; target = i;
} // end new max found return target;
} // end function findMinSales
void sortBySales () { int target; for (int i = 0; i < count; i++) {
target = findMinSales (i); if (target > i)
switchRows (i, target); } // for each concert
} // end function sortBySales
void getData () { // for (int i = 0; i < MAXG; i++) sales [i] = 0; printf ("Enter ticket prices in each of %d cateogories: ", MAXC); for (int i = 0; i < MAXC; i++)
scanf ("%f", &prices [i]); printf ("-- Enter group and fans in %d categories ", MAXC); printf (" . to finish entries: "); for (int i = 0; i < MAXG; i++) {
scanf ("%s", &group[i]); if (group [i][0] == '.')
break; count++;
for (int j = 0; j < MAXC; j++) scanf ("%d", &fans[i][j]);
} // end for each group } // end function getData
int main(void) { getData (); computeSales (); printArray (); printf (" --- Sorted --- "); sortBySales ();
printArray (); printf("... bye ... "); return 0;
}
Test Plan
To verify this program is working properly a variety of test cases should be used. The following is just one test case, which only tests a very few aspects of the program.
You should be thinking about other data sets to test other aspects of the program, and also create more realistic test sets.
Test Case | Input | Actual Output using repl.it user input in red: |
1 | 123 a123 b331 c531 d335 e112 f943 g456 . | Enter ticket prices in each of 3 cateogories: 1 2 3 -- Enter group and fans in 3 categories . to finish entries: a123 b331 c531 d335 e112 f943 g456 . --- Sorted --- Concert s1 s2 s3 Sales ... bye ... Concert s1 s2 s3 Sales a123 14.00 b331 12.00 c531 14.00 d335 24.00 e112 9.00 f943 26.00 g456 32.00 e112 9.00 b331 12.00 c531 14.00 a123 14.00 d335 24.00 f943 26.00 g456 32.00 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
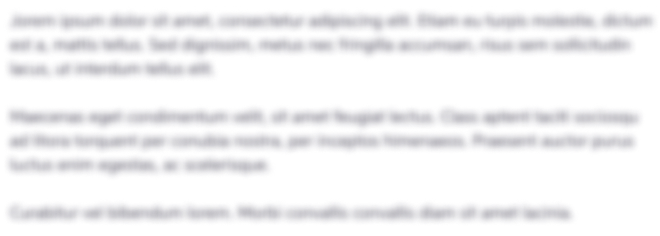
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started