Question
Modify the simple race game so that a player who loses all of his points - due to a collision - loses his next turn
Modify the simple race game so that a player who loses all of his points - due to a collision - loses his next turn and must roll doubles to be able to move again.
A Simple Race Game
Players take turns throwing two dice. A player adds the sum of the dice to his points. If his points equals the maximum points then the game ends and the player wins. If his points are greater than the maximum points then the player loses one-half of his points and also loses his next turn. If the numbers on the two dice are equal then the player gets an extra turn. If the players points equals some other players points then the player gets an extra turn and the other player loses all his points and also loses his next turn.
If players loses a turn then his turns is a negative number and if player wins an extra turn then his turns is a positive number. Otherwise is turns is zero.
Interactions between players may become very complicated. It is possible for a player to lose more than one turn or win more than one extra turn. A player who loses his next and one half of his points may lose all his points and another turn if another player collides with him before his turn comes again; and a player who rolls doubles and collides with another player gets two extra turns.
The complexity of the interactions between the players requires a state table. The players state describes the player immediately after the player rolls the dice and determines how the player responds to the dice. A players state variables are the dice, the players points, and the players collision status.
Two numbers describe the dices state 0 or 1 - the dice are not equal or are equal.
Two numbers describe the collisions state 0 or 1 - a collision does not occur or does occur.
Three numbers describe the points state the points are less than the maximum points, equal to the maximum points, or greater than the maximum points 0, 1, or 2.
The number of combinations of the individual states is 2 x 2 x 3 = 12. That is, at any time a player is in any one of thirty-six states.
We define a state function. The state function uses the state variables to compute the players state a number between 0 and 11.
dice | collide | points | state | actions |
0 | 0 | 0 | 0 | 0 |
0 | 0 | 1 | 1 | 1 |
0 | 0 | 2 | 2 | 2 |
0 | 1 | 0 | 3 | 3 |
0 | 1 | 1 | 4 | impossible-4 |
0 | 1 | 2 | 5 | impossible-5 |
1 | 0 | 0 | 6 | 4 |
1 | 0 | 1 | 7 | 1 |
1 | 0 | 2 | 8 | 5 |
1 | 1 | 0 | 9 | 6 |
1 | 1 | 1 | 10 | impossible-10 |
1 | 1 | 2 | 11 | impossible-11 |
One of the state variables has three values so a pure binary state function would be a little inefficient. A simple state function is:
s(d,c,p) = 6d + 3c + p
where d is the dice state, c is the collision state, p is the points state.
We now associate a set of actions with each state.
0 do nothing
1 end game
2 player loses points and turn
3 player gets extra turn, other player loses points and turn
4 player gets extra turn
5 player loses points
6 player gets 2 extra turns, other player loses points and turn
States 4, 5, 10, and 11 are impossible because two players cannot have maximum points or more than maximum points at the same time.
Note:
The 12 state version of the simple race game has an ugly if statement.
If we add the players 3 value turns property to the state function then the function has 36 values and the state function manages the turns. In some of those states the player rolls the dice and in some the player does not. We have to be careful. We have to make sure that in every state we change at least one state variable. It will sometimes be the case that we change state variables and yet remain in the same state. For example, if a player has several extra turns. In that case the player may continue to roll the dice and remain in the same state until the player has no more extra turns.
At present, the scheduler managed the players turns and the ugly if statement decides who throws the dice and who doesnt.
InFixToPostFix.cpp---------------------------------------------------------------------------------------------------------
#include
void InFixToPostFix::convertToPostfix() { pfx = ""; stack
InFixToPostFix.h-------------------------------------------------------------------------------------------------------------------------------
#pragma once #include
class InFixToPostFix { public: InFixToPostFix(void); bool getInFix(const string& new_infx); void showInFix(ostream& os = cout); void showPostFix(ostream& os = cout); private: void convertToPostfix(); bool isOperand(char symbol); bool isOperator(char symbol); bool isLeftParen(char symbol); bool isRightParen(char symbol); bool precedence(char op1, char op2); private: string pfx; string infx; };
infixtopostfixsource.cpp-------------------------------------------------------------------------------------------
// ConsoleApplication3.cpp : Defines the entry point for the console application. //
int main() { return 0; }
newsource.cpp----------------------------------------------------------------------------------
#include
using namespace std;
const int N = 5; const int M = 64;
struct Player { string name; int turns; int points; }; struct Dice { int die1; int die2; };
default_random_engine e; uniform_int_distribution
void init(string names[]) { for(int index = 0; index < N; index++) { players[index].points = 0; players[index].turns = 0; players[index].name = names[index]; } } void rollDice() { dice.die1 = uDice(e); dice.die2 = uDice(e); }
int findCollision(int player_index) { int old_points = players[player_index].points; if (old_points == -1) players[player_index].points = dice.die1 + dice.die2; else players[player_index].points += dice.die1 + dice.die2; int points = players[player_index].points; int index = 0;
while (index < N && (players[index].points != points || index == player_index)) index++; players[player_index].points = old_points; if (index < N) return index; else return -1; } void manageCollision(int player_index, int collision_index) { players[collision_index].points = 0; players[collision_index].turns--; players[player_index].turns++; } int computePointsState(int player_index) { int old_points = players[player_index].points; if (old_points == -1) players[player_index].points = dice.die1 + dice.die2; else players[player_index].points += dice.die1 + dice.die2; int points = players[player_index].points; int points_state; if (points < -1) points_state = 3; else if (0 < points && points < M) points_state = 0; else if (points == M) points_state = 1; else points_state = 2; players[player_index].points = old_points; return points_state; } int computeState(int player_index) { int dice_state = dice.die1 == dice.die2 ? 1 : 0; int collision_state = findCollision(player_index) > -1 ? 1 : 0;
int points_state = computePointsState(player_index); int state = 8 * dice_state + 4 * collision_state + points_state; return state; } int main() { string names[] = { "Ned", "Zed", "Jed", "Ted", "Red" }; init( names); e.seed((unsigned)time(0)); int index = uPlayers(e); bool game_continues = true; int throw_count = 0; do { if(players[index].turns < 0) { players[index].turns++; cout << players[index].name << " gives up dice" << endl; index = (index + 1) % N; } else { rollDice(); int sum_dice = dice.die1 + dice.die2; //players[index].points += sum_dice; //cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; int state = computeState(index); int index2 = -1; switch (state) { case 0: players[index].points += sum_dice; cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; break; case 1: case 7: players[index].points += sum_dice; cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; cout << players[index].name << " wins" << endl; game_continues = false; break; case 2: players[index].points /= 2; players[index].turns--; cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; cout << players[index].name << " loses 1/2 points and next turn" << endl; index2 = findCollision(index); if (index2 != -1) { manageCollision(index, index2); cout << players[index].name << " recovers next turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; } break; case 3: cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; cout << players[index].name << " did not roll doubles and does not move" << endl; break; case 4: cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; players[index].points += sum_dice; index2 = findCollision(index); manageCollision(index, index2); cout << players[index].name << " gets extra turn - collision" << endl; cout << players[index2].name << " loses points and must roll doubles to move again - collision" << endl; break; case 8: cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; players[index].points += sum_dice; players[index].turns++; cout << players[index].name << " gets extra turn - doubles" << endl; break; case 10: players[index].points /= 2; cout << players[index].name << " loses 1/2 points" << endl; index2 = findCollision(index); if (index2 != -1) { manageCollision(index, index2); cout << players[index].name << " recovers extra turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; } break; case 9: players[index].turns++; index2 = findCollision(index); manageCollision(index, index2); cout << players[index].name << " gets extra turn - doubles" << endl; cout << players[index].name << " gets extra turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; break; } if (players[index].turns <= 0) index = (index + 1) % N; else players[index].turns--; } } while(game_continues); std::system("pause"); return 0; }
oldsource.cpp------------------------------------------------------------------------------
#include
using namespace std;
const int N = 5; const int M = 64;
struct Player { string name; int turns; int points; }; struct Dice { int die1; int die2; };
default_random_engine e; uniform_int_distribution
void init(string names[]) { for(int index = 0; index < N; index++) { players[index].points = 0; players[index].turns = 0; players[index].name = names[index]; } } void rollDice() { dice.die1 = uDice(e); dice.die2 = uDice(e); }
int findCollision(int player_index) { int points = players[player_index].points; int index = 0;
while (index < N && (players[index].points != points || index == player_index)) index++; if (index < N) return index; else return -1; } void manageCollision(int player_index, int collision_index) { players[collision_index].points = 0; players[collision_index].turns--;; players[player_index].turns++; } int computeState(int player_index) { int dice_state = dice.die1 == dice.die2 ? 1 : 0; int collision_state = findCollision(player_index) > -1 ? 1 : 0; int points = players[player_index].points; int points_state = points < M ? 0 : points == M ? 1 : 2; int state = 6 * dice_state + 3 * collision_state + points_state; return state; } int main() { string names[] = { "Ned", "Zed", "Jed", "Ted", "Red" }; init( names); e.seed((unsigned)time(0)); int index = uPlayers(e); bool game_continues = true; int throw_count = 0; do { if(players[index].turns < 0) { players[index].turns++; cout << players[index].name << " gives up dice" << endl; index = (index + 1) % N; } else { rollDice(); int sum_dice = dice.die1 + dice.die2; players[index].points += sum_dice; cout << setw(4) << ++throw_count << '/' << players[index].name << '/' << sum_dice << '/' << players[index].points << endl; int state = computeState(index); int index2 = -1; switch (state) { case 0: break; case 1: case 7: cout << players[index].name << " wins" << endl; game_continues = false; break; case 2: players[index].points /= 2; players[index].turns--; cout << players[index].name << " loses 1/2 points and next turn" << endl; index2 = findCollision(index); if (index2 != -1) { manageCollision(index, index2); cout << players[index].name << " recovers next turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; } break; case 3: index2 = findCollision(index); manageCollision(index, index2); cout << players[index].name << " gets extra turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; break; case 6: players[index].turns++; cout << players[index].name << " gets extra turn - doubles" << endl; break; case 8: players[index].points /= 2; cout << players[index].name << " loses 1/2 points" << endl; index2 = findCollision(index); if (index2 != -1) { manageCollision(index, index2); cout << players[index].name << " recovers extra turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; } break; case 9: players[index].turns++; index2 = findCollision(index); manageCollision(index, index2); cout << players[index].name << " gets extra turn - doubles" << endl; cout << players[index].name << " gets extra turn - collision" << endl; cout << players[index2].name << " loses points and next turn - collision" << endl; break; } if (players[index].turns <= 0) index = (index + 1) % N; else players[index].turns--; } } while(game_continues); std::system("pause"); return 0; }
palindromeswithstacks&queues.cpp-----------------------------------------------------------------------------
// ConsoleApplication1.cpp : Defines the entry point for the console application. //
#include
bool isPalindrome(const string& source) { stack
for(int index = 0; index < source.length(); index++) if (isalpha(source[index])) { char c = toupper(source[index]); s.push(c); q.push(c); } while (!s.empty() && s.top() == q.front()) { s.pop(); q.pop(); } return s.empty(); } int main() { if (isPalindrome("Madam, I'm Adam!")) cout << "Palindrome!" << endl; else cout << "Not!" << endl; if (isPalindrome("Madam, I am Adam!")) cout << "Palindrome!" << endl; else cout << "Not!" << endl; return 0; }
stacksandqueues.cpp------------------------------------------------------------------------------------------
#include
template
Step by Step Solution
There are 3 Steps involved in it
Step: 1
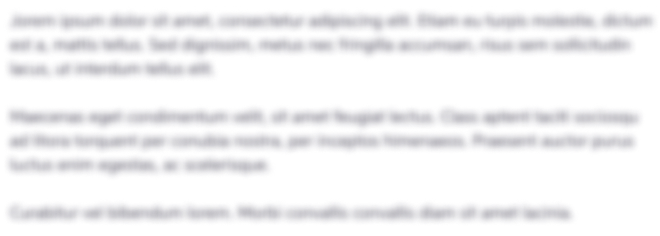
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started