Question
Modify your java program in problem number 1. Instead of having one queue. You are tasked to create a ticketing system for Customer Service counters.
Modify your java program in problem number 1. Instead of having one queue. You are tasked to create a ticketing system for Customer Service counters. Assuming there are 2 counters that need to serve customers of 2 different types of concerns. These are Technical Issues and Billing.
Create a ticketing system that will help the Customer Service department in assisting their customer.
Note: The program should have a menu as shown below and include all necessary error checking for this program.
Menu
[1] Choose Services
[a] Billing
[b] Technical Issue [2] Task Accomplished
[a] Billing
[b] Technical Issue
[3] Display Number of Customers
[a] Billing
[b] Technical Issue [4] Exit
Enter your choice:
Continue on this code :
class Queue {
private int maxSize;
private long[] queArray;
private int front;
private int rear;
private int nItems;
//--------------------------------------------------------------
public Queue(int s) // constructor
{
maxSize = s;
queArray = new long[maxSize];
front = 0;
rear = -1;
nItems = 0;
}
//--------------------------------------------------------------
public void insert(long j) // put item at rear of queue
{
if (rear == maxSize - 1) // deal with wraparound
{
rear = -1;
}
queArray[++rear] = j; // increment rear and insert
nItems++; // one more item
}
//--------------------------------------------------------------
public long remove() // take item from front of queue
{
long temp = queArray[front++]; // get value and incr front
if (front == maxSize) // deal with wraparound
{
front = 0;
}
nItems--; // one less item
return temp;
}
//--------------------------------------------------------------
public long peekFront() // peek at front of queue
{
return queArray[front];
}
//--------------------------------------------------------------
public boolean isEmpty() // true if queue is empty
{
return (nItems == 0);
}
//--------------------------------------------------------------
public boolean isFull() // true if queue is full
{
return (nItems == maxSize);
}
//--------------------------------------------------------------
public int size() // number of items in queue
{
return nItems;
}
//--------------------------------------------------------------
} // end class Queue
////////////////////////////////////////////////////////////////
show the code and output copy and past pleaseee
Step by Step Solution
There are 3 Steps involved in it
Step: 1
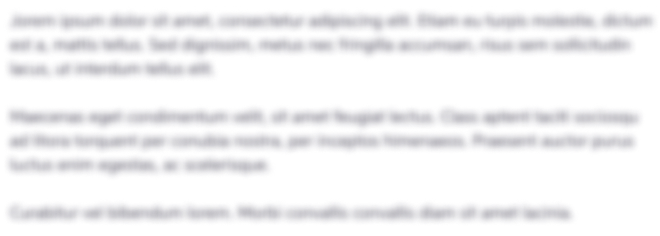
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started