Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Module 1 Recommended Implementation Details In this first part of the signature assignment, develop code to write and read a binary file. An array of
Module Recommended Implementation
Details
In this first part of the signature assignment, develop code to write and read a binary file. An
array of random numbers will be created, and the number of elements and values of each element
will be written into a binary file. Then, to ensure that all is working well, the array is deleted, a
new one is created, and the binary file is read. The first value is the number of elements to read,
which value should control a loop. Then, each value is read and stored in an array element.
Write a function named createArray that creates a dynamic array of integers. The
main function must supply an argument for the length of the array. Use a constant defined with
the value of in the calling code. It is best to define the constant outside the main function.
In createArray, initialize the array with random numbers between and inclusive.
Return a pointer to the dynamically created array to the calling code in main.
Write a second function named writeBinary that takes as a parameter a pointer to an integer
array and a second parameter, which is the arrays length. Open a binary output file named
binarydat as output and write to it an integer indicating the array's length the value of the
parameter Then, in a loop, write each element in the array to the file and close the file. Be sure
to use a reinterpretcast to convert the location of each integer to a constant character
pointer.
Write a third function named readBinary that opens the binary file binarydat file for
input. Its only function parameter should reference an integer in the main function that will store
the length of the returned arraythe length must be read from the file, not passed as a
parameter. Read the first value from the binary file, which is an integer representing the length
of the new array to create; use the address of the reference parameter as the target of a
reinterpretcast. Next, create a new dynamic integer array using the length value for the
size. In a loop, read each number from the binary file and save the value in the array. Return the
pointer to the array from the function.
In the main function, call createArray, passing the array's length use the defined
constant This function will return a pointer to the array, which should be saved in a local
variable. Call the writeBinary function, passing the pointer to the array as the first argument
and the length as a second argument. After writeBinary returns, delete the array created in
the first function and set its pointer to nullptr.
Define a local integer variable for an arrays length do not use the defined constant and an
integer pointer for the array itself. Call the readBinary function, passing a reference to the
variable for the array length. The function will return a pointer to a new array, which should be
saved, and the length variable will be updated. Write a loop to iterate over each value in the
array and print out its value separated by a space. Delete the dynamic array and terminate the
program.
Use these function headers:
int createArrayint length
int readBinaryint& length
void writeBinaryint values, int length
Example output:
Module Recommended Implementation
Details
This second part of the signature assignment will rework the code to create the binary file. Then,
you will develop classes for reading and analyzing the binary data read from the file.
Write a function named createBinaryFile, which takes the name of a file as its first
parameter and the size of the data as the second parameter and returns nothing. Copy the code
from the createArray function for creating the array of random values into this function.
Modify writeBinary to take an additional string parameter; it is the file name. Add a call to
the existing writeBinary function and pass three parameters. When this code works
correctly, eliminate the createArray function since it is no longer needed.
Write a class called BinaryReader. It should have instance variables of an integer pointer
and an integer. The pointer will point to an array of integers; named values. The integer will
hold the arrays size, named size. Add a constructor that takes a single parameter, a constant
string reference to a file name. Add a private method called readValues with one parameter,
the name of the input file. Copy the code from the readBinary function to open the file, input
the array size into the size instance variable, and create the dynamic array using the values
instance variable. Now, read the values from the file into the array. Eliminate the existing
readBinary function. The constructor should call the readValues method to populate the
array and size instance variables. Add a destructor to delete the integer array. Fi
Step by Step Solution
There are 3 Steps involved in it
Step: 1
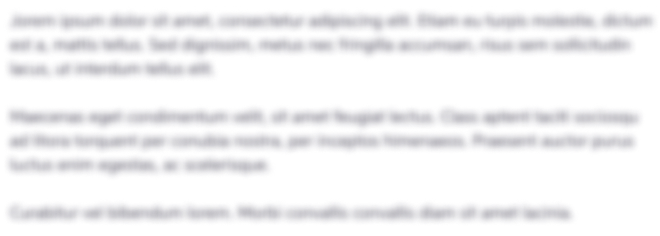
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started