Question
module BusControl(MAX10_CLK1_50, KEY, SW, HEX0, HEX1, HEX2, HEX3, HEX4, HEX5, LEDR); input MAX10_CLK1_50; // System clock input [1:0] KEY; // DE10 Pushbuttons input [9:0] SW;
module BusControl(MAX10_CLK1_50, KEY, SW, HEX0, HEX1, HEX2, HEX3, HEX4, HEX5, LEDR); input MAX10_CLK1_50; // System clock input [1:0] KEY; // DE10 Pushbuttons input [9:0] SW; // DE10 Switches output [6:0] HEX0; // DE10 Seven-segment displays output [6:0] HEX1; output [6:0] HEX2; output [6:0] HEX3; output [6:0] HEX4; output [6:0] HEX5; output [9:0] LEDR; // DE10 LEDs
// END MODULE AND PORT DECLARATION
// BEGIN WIRE DECLARATION // Buttons is the output of a Finite State Machine. // Each time that one of the DE10 pushbuttons is pressed and released, the corresponding value of BUTTONS goes high for one clock period. // This ensures that a single press and release of a pushbutton enables only one register transfer. // YOU MUST USE THE VALUES FROM BUTTONS INSTEAD OF tHE VALUES FROM KEY IN YOUR IMPLEMENTATION. wire [1:0] buttons; // DO NOT MODIFY!
// These values represent the register states. // The synthesis keep directive will allow you to view these wires in simulation.
wire [7:0] val0 /* synthesis keep */ ; // DO NOT MODIFY! wire [7:0] val1 /* synthesis keep */ ; // DO NOT MODIFY! wire [7:0] val2 /* synthesis keep */ ; // DO NOT MODIFY! wire [7:0] valL /* synthesis keep */ ; // DO NOT MODIFY! wire [7:0] valA /* synthesis keep */ ; // DO NOT MODIFY! wire [7:0] valB /* synthesis keep */ ; // DO NOT MODIFY! wire [7:0] valC /* synthesis keep */ ; // DO NOT MODIFY!
// You MAY alter these wire declarations if you wish, or even delete them entirely. // What you replace them with will depend on your design.
wire load; // Register Load wire [7:0] bus; // Input bus
// Add your other wire declarations here wire load0,load1,load2,loadL,loadA,loadB,loadC; wire [7:0]inA,inB,inC,inL; wire L1,L2;
// END WIRE DECLARATION // BEGIN TOP-LEVEL HARDWARE MODEL // // Review the hardware description for the buttonpress module in buttonpress.v. // Use BUTTONS as the control signal for your hardware instead of KEY. // This ensures that a single press and release of a pushbutton enables only one register transfer. // DO NOT ALTER THE INSTANTIATIONS OF THE buttonpress MODULES!
// System clock Pushbutton Enable buttonpress bp1(MAX10_CLK1_50, KEY[1], buttons[1]); buttonpress bp0(MAX10_CLK1_50, KEY[0], buttons[0]);
// These seven-segment decoders show the associated 8-bit register values in hexadecimal on two seven-segment displays // DO NOT ALTER THE INSTANTIATIONS OF THE hexDecoder_7seg MODULES!
// Upper Hex Display Lower Hex Display Register Value hexDecoder_7seg h1(HEX5, HEX4, valA); hexDecoder_7seg h2(HEX3, HEX2, valB); hexDecoder_7seg h3(HEX1, HEX0, valC);
// This continuous assignment connects the valL to the LEDs. // DO NOT ALTER THIS CONTINUOUS ASSIGNMENT! assign LEDR = {2'b00, valL};
// Review the hardware description for the register module in register8bit.v // Register rL acts as both r3 and rD. It should be capable of all register transfers described in the specification. // CHANGE THE LOAD CONTROL and the REGISTER INPUTS as needed by the system you are trying to implement. // DO NOT CHANGE THE CLOCK SOURCE OR THE REGISTER OUTPUT!
// System clock Load control Register inputs Register outputs register8bit r0(MAX10_CLK1_50, load0, SW[7:0], val0); register8bit r1(MAX10_CLK1_50, load1, SW[7:0], val1); register8bit r2(MAX10_CLK1_50, load2, SW[7:0], val2); register8bit rL(MAX10_CLK1_50, loadL, inL, valL);
register8bit rA(MAX10_CLK1_50, loadA, inA, valA); register8bit rB(MAX10_CLK1_50, loadB, inB, valB); register8bit rC(MAX10_CLK1_50, loadC, inC, valC); // Instantiate all other hardware here. // You may also add continuous assignments here. dec2_4 d1(SW[9],SW[8],buttons[0],load0,load1,load2,L1); dec2_4 d2(SW[9],SW[8],buttons[1],loadA,loadB,loadC,L2);
assign loadL = L1^L2;
transfer t0(inA,buttons[1],SW[7],SW[6],val0,val1,val2,valL); transfer t1(inB,buttons[1],SW[7],SW[6],val0,val1,val2,valL); transfer t2(inC,buttons[1],SW[7],SW[6],val0,val1,val2,valL); transfer t3(inL,(buttons[1]^buttons[0]),SW[7],SW[6],val0,val1,val2,valL);
// END TOP-LEVEL HARDWARE MODEL //
endmodule
//////////////////////////////////////////////////////////////////////////////////////////////////// // // Module: tsg_8bit // Author: // Created: // Description: An 8-bit wide tri-state gate module written using Verilog primitives // ////////////////////////////////////////////////////////////////////////////////////////////////////
// Verilog primitive gate bufif1: // - Syntax: bufif1(out, in, enable) // out, in, and enable must all be SCALARS. // - Function: If enable = 1, then out = in. If enable = 0, then out = Z. // // Desired functionality of tsg_8bit: // - If en = 1, out = in. // - If en = 0, out = 8'bzzzz_zzzz.
// USING THE bufif1 VERILOG PRIMITIVE, IMPLEMENT THE FUNCTIONALITY FOR tsg_8bit. // Once you have implemented tsg_8bit correctly, you can place instances of it into BusControl.
module tsg_8bit(in, en, out); input [7:0] in; // 8-bit input input en; // Enable bit output [7:0] out; // 8-bit output
bufif1 b1(out[7:0], in[7:0], en);
endmodule
// Write the remaining hardware models that you instantiate into the top-level module starting here. module dec2_4 (a,b,en,y0,y1,y2,y3);
input a, b, en; output y0,y1,y2,y3;
assign y0= (~a) & (~b) & en; assign y1= (~a) & b & en; assign y2= a & (~ b) & en; assign y3= a & b & en;
endmodule
//////////////////////////////////////////////////////////////////////
module transfer(result,en1,SW1,SW2,R0,R1,R2,RL);
input SW1,SW2; input [7:0]R0; input [7:0]R1; input [7:0]R2; input [7:0]RL; input en1; output [7:0]result;
wire D0,D1,D2,D3;
dec2_4 (SW1,SW2,en1,D0,D1,D2,D3); tsg_8bit(R0, D0, result); tsg_8bit(R1, D1, result); tsg_8bit(R2, D2, result); tsg_8bit(RL, D3, result);
endmodule
How could I debug this? Worked on this for hours, Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
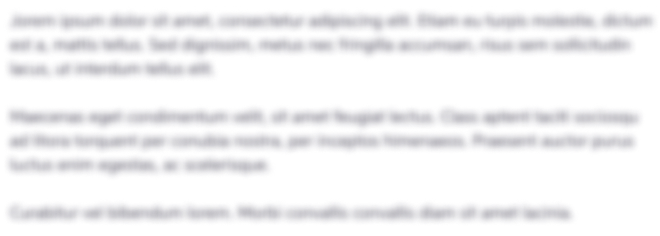
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started