Question
Murach Android Programming Exercise 7-3 Modify a Theme I am using Android Studio. Here are the instructions for the exercise, I have not tried to
Murach Android Programming Exercise 7-3 Modify a Theme
I am using Android Studio. Here are the instructions for the exercise, I have not tried to modify the theme because I am struggling with #4 the Radio Group, when I run it in the emulator, something is not correct. There is supposed to be a +PercentUp and -PercentDown button as well. Under the instructions I will paste my code. Thanks in advance.
In this exercise, you'll modify the theme that's used by the Tip Calculator app presented in this chapter.
1. Open the project named ch07_ex_TipCalculator.
2. Open the styles.xml file in the values directory. This file modifies the theme to automatically apply custome style named TextView.Label that can be applied manually.
3. Open the layout for the app and view its XML. This XML only applies the TextView.Label style.
4. Add a RadioGroup widget to the layout. Note that the radio buttons withing the group use a different text size than the other widgets in the layout.
5. Modify the styles.xml file so it modifies the TextAppearance styles fo the theme instead of modifying the styles for individual widgets. This should set set the textSize property to 20sp for the TextAppearance style and its Small, Medium, and Inverse variations. When you're done, all widgets should use a textSize of 20sp.
package com.murach.tipcalculator; import java.text.NumberFormat; import android.os.Bundle; import android.view.KeyEvent; import android.view.View; import android.view.View.OnClickListener; import android.view.inputmethod.EditorInfo; import android.widget.Button; import android.widget.EditText; import android.widget.RadioButton; import android.widget.RadioGroup; import android.widget.RadioGroup.OnCheckedChangeListener; import android.widget.TextView; import android.widget.TextView.OnEditorActionListener; import android.app.Activity; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; public class TipCalculatorActivity extends Activity implements OnEditorActionListener, OnCheckedChangeListener, OnClickListener { // define variables for the widgets private EditText billAmountEditText; private TextView percentTextView; private Button percentUpButton; private Button percentDownButton; private TextView tipTextView; private TextView totalTextView; private RadioGroup roundingRadioGroup; private RadioButton roundNoneRadioButton; private RadioButton roundTipRadioButton; private RadioButton roundTotalRadioButton; // define the SharedPreferences object private SharedPreferences savedValues; //define rounding constants private final int ROUND_NONE = 0; private final int ROUND_TIP = 1; private final int ROUND_TOTAL = 2; // define instance variables that should be saved private String billAmountString = ""; private float tipPercent = .15f; private int rounding = ROUND_NONE; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_tip_calculator); // get references to the widgets billAmountEditText = (EditText) findViewById(R.id.billAmountEditText); percentTextView = (TextView) findViewById(R.id.percentTextView); percentUpButton = (Button) findViewById(R.id.percentUpButton); percentDownButton = (Button) findViewById(R.id.percentDownButton); tipTextView = (TextView) findViewById(R.id.tipTextView); totalTextView = (TextView) findViewById(R.id.totalTextView); roundingRadioGroup = (RadioGroup) findViewById(R.id.roundingRadioGroup); roundNoneRadioButton = (RadioButton) findViewById(R.id.roundNoneRadioButton); roundTipRadioButton = (RadioButton) findViewById(R.id.roundTipRadioButton); roundTotalRadioButton = (RadioButton) findViewById(R.id.roundTotalRadioButton); // set the listeners billAmountEditText.setOnEditorActionListener(this); percentUpButton.setOnClickListener(this); percentDownButton.setOnClickListener(this); roundingRadioGroup.setOnCheckedChangeListener(this); // get SharedPreferences object savedValues = getSharedPreferences("SavedValues", MODE_PRIVATE); } @Override public void onPause() { // save the instance variables Editor editor = savedValues.edit(); editor.putString("billAmountString", billAmountString); editor.putFloat("tipPercent", tipPercent); editor.putInt("rounding", rounding); editor.commit(); super.onPause(); } @Override public void onResume() { super.onResume(); // get the instance variables billAmountString = savedValues.getString("billAmountString", ""); tipPercent = savedValues.getFloat("tipPercent", 0.15f); rounding = savedValues.getInt("rounding", ROUND_NONE); // set the bill amount on its widget billAmountEditText.setText(billAmountString); calculateAndDisplay(); //set rounding on radio buttons if (rounding == ROUND_NONE) { roundNoneRadioButton.setChecked(true); } else if (rounding == ROUND_TIP) { roundTipRadioButton.setChecked(true); } else if (rounding == ROUND_TOTAL) { roundTotalRadioButton.setChecked(true); } } public void calculateAndDisplay() { // get the bill amount billAmountString = billAmountEditText.getText().toString(); float billAmount; if (billAmountString.equals("")) { billAmount = 0; } else { billAmount = Float.parseFloat(billAmountString); } // calculate tip and total float tipAmount = 0; float totalAmount = 0; if (rounding == ROUND_NONE) { tipAmount = billAmount * tipPercent; totalAmount = billAmount + tipAmount; } else if (rounding == ROUND_TIP) { tipAmount = StrictMath.round(billAmount * tipPercent); totalAmount = billAmount + tipAmount; // tipPercent = totalAmount - billAmount; } else if (rounding == ROUND_TOTAL) { float tipNotRounded = billAmount * tipPercent; totalAmount = StrictMath.round(billAmount + tipNotRounded); tipAmount = totalAmount - billAmount; tipPercent = tipAmount / billAmount; } // display the other results with formatting NumberFormat currency = NumberFormat.getCurrencyInstance(); tipTextView.setText(currency.format(tipAmount)); totalTextView.setText(currency.format(totalAmount)); NumberFormat percent = NumberFormat.getPercentInstance(); percentTextView.setText(percent.format(tipPercent)); } @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { if (actionId == EditorInfo.IME_ACTION_DONE || actionId == EditorInfo.IME_ACTION_UNSPECIFIED) { calculateAndDisplay(); } return false; } @Override public void onClick(View v) { switch (v.getId()) { case R.id.percentDownButton: tipPercent = tipPercent - .01f; calculateAndDisplay(); break; case R.id.percentUpButton: tipPercent = tipPercent + .01f; calculateAndDisplay(); break; } } @Override public void onCheckedChanged(RadioGroup group, int checkedId) { switch (checkedId) { case R.id.roundNoneRadioButton: rounding = ROUND_NONE; break; case R.id.roundTipRadioButton: rounding = ROUND_TIP; break; case R.id.roundTotalRadioButton: rounding = ROUND_TOTAL; break; } calculateAndDisplay(); } }
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="10dp" > <TextView android:id="@+id/billAmountLabel" style="@style/TextView.Label" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/bill_amount_label" /> <EditText android:id="@+id/billAmountEditText" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/billAmountLabel" android:layout_marginLeft="5dp" android:layout_toRightOf="@+id/billAmountLabel" android:ems="8" android:inputType="numberDecimal" android:text="" > <requestFocus /> EditText> <TextView android:id="@+id/percentLabel" style="@style/TextView.Label" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/billAmountLabel" android:layout_below="@+id/billAmountLabel" android:text="@string/tip_percent_label" /> <TextView android:id="@+id/percentTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/percentLabel" android:layout_alignLeft="@+id/billAmountEditText" android:padding="5dp" android:text="@string/tip_percent" /> <Button android:id="@+id/percentDownButton" android:layout_width="45dp" android:layout_height="45dp" android:layout_alignBaseline="@+id/percentLabel" android:layout_marginLeft="25dp" android:layout_toRightOf="@+id/percentTextView" android:text="@string/decrease" /> <Button android:id="@+id/percentUpButton" android:layout_width="45dp" android:layout_height="45dp" android:layout_alignBaseline="@+id/percentLabel" android:layout_toRightOf="@+id/percentDownButton" android:text="@string/increase" /> <TextView android:id="@+id/tipLabel" style="@style/TextView.Label" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/percentLabel" android:layout_below="@+id/percentLabel" android:text="@string/tip_amount_label" /> <TextView android:id="@+id/tipTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/tipLabel" android:layout_alignLeft="@id/billAmountEditText" android:padding="5dp" android:text="@string/tip_amount" /> <TextView android:id="@+id/totalLabel" style="@style/TextView.Label" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/tipLabel" android:layout_below="@+id/tipLabel" android:text="@string/total_amount_label" /> <TextView android:id="@+id/totalTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/totalLabel" android:layout_alignLeft="@+id/tipTextView" android:padding="5dp" android:text="@string/total_amount" /> <TextView android:id="@+id/roundingLabel" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/totalLabel" android:layout_below="@+id/totalLabel" android:text="Rounding" android:textStyle="bold" android:layout_marginLeft="10dp"/> <RadioGroup android:id="@+id/roundingRadioGroup" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" android:layout_below="@+id/totalLabel" android:layout_toRightOf="@+id/billAmountLabel"> <RadioButton android:id="@+id/roundNoneRadioButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:checked="true" android:text="None" /> <RadioButton android:id="@+id/roundTipRadioButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tip" /> <RadioButton android:id="@+id/roundTotalRadioButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Total" /> RadioGroup> RelativeLayout>
<resources> <string name="app_name">Tip Calculatorstring> <string name="title_activity_tip_calculator">Tip Calculatorstring> <string name="menu_settings">Settingsstring> <string name="bill_amount_label">Bill Amountstring> <string name="bill_amount">32.60string> <string name="tip_percent_label">Percentstring> <string name="tip_percent">15%string> <string name="increase">+string> <string name="decrease">-string> <string name="tip_amount_label">Tipstring> <string name="tip_amount">$0.00string> <string name="total_amount_label">Totalstring> <string name="total_amount">$0.00string> <string name ="rounding_label"> Roundingstring> <string name ="round_none">Nonestring> <string name ="round_tip">Tipstring> <string name ="round_total">Totalstring> resources>
Step by Step Solution
There are 3 Steps involved in it
Step: 1
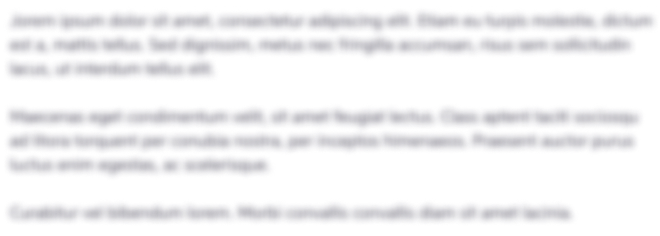
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started