Question
Music.java public class Music { // instance variables private int year; private String title; private String artist; // Constructor for objects of class Music public
Music.java
public class Music { // instance variables private int year; private String title; private String artist;
// Constructor for objects of class Music public Music(String t, int y, String a) { // initialize instance variables title = t; year = y; artist = a; }
public String getTitle() { return title; } public void setTitle(String t) { title = t; } public String getArtist() { return artist; } public void setArtist(String a) { artist = a; } public int getYear() { return year; } public void setTitle(int y) { year = y; } public String toString() { String str = String.format( "%-25s %4d %-20s ", title, year , artist); return str; } }
MusicTester.java
public class MusicTester { public static void main(String[] args) { //Declare an array of 10 Music final int SIZE = 10; Music[] musicArray = new Music[SIZE]; //initialize music array musicArray[0] = new Music("test drive",2021, "Ariana Grande"); musicArray[1] = new Music("We're Good",2021, "Dua Lipa"); musicArray[2] = new Music("Billie Jean",1982, "Michael Jackson"); musicArray[3] = new Music("In My Life",1965, "The Beatles"); musicArray[4] = new Music("I walk the line",1956, "Johnny Cash"); musicArray[5] = new Music("Like a Prayer",1984, "Madonna"); musicArray[6] = new Music("When Doves Cry",1984, "Prince"); musicArray[7] = new Music("Growl",2013, "EXO"); musicArray[8] = new Music("I wanna dance with somebody",1987, "Whitney Houston"); musicArray[9] = new Music("Baby one more time",1998, "Britney Spears");
//Call function printMusic(musicArray); searchArtist(musicArray, "EXO"); searchTitle(musicArray,"Like a Prayer"); searchTitle(musicArray, "test drive"); searchArtist(musicArray,"Dua Lipa"); searchYear(musicArray , 1998); } private static void printMusic(Music[] music) { String str = String.format( "%-25s %4s %-20s ", "Title", "Year" , "Artist"); System.out.println(str); System.out.println("==========================================="); for (Music m:music ) { System.out.println(m); } } //Searches array for particular song private static void searchTitle(Music[] music, String title) { for (Music m:music ) { if(m.getTitle().equalsIgnoreCase(title)) { System.out.println(title+" is found."); return; } } System.out.println(title+" not found."); } private static void searchArtist(Music[] music, String artist) { for (Music m:music ) { if(m.getArtist().equalsIgnoreCase(artist)) { System.out.println(artist+" is found."); return; } } System.out.println(artist+" not found."); } private static void searchYear(Music[] music, int year) { for (Music m: music) { if(m.getYear() == year) { System.out.println(year+" is found."); return; } } System.out.println(year+" is found."); } }
Instructions: For this assignment, you will create several binary search methods and perform the searches using a collection of music. 1. Create a new project called 08.02 Assignment in your Module 08 assignments folder. 2. Copy your Music.java and tester file from the previous search project as a starting point. Rename the tester to v3. Delete the existing search methods. 3. Declare an array of at least 10 Music objects. For each, you will need a song title, year, and artist name. At least one year needs to have multiple entries in the array. Same with one of the artists. Of course, be sure to use school-appropriate songs. For example: Livin' on a Prayer, 1986, Bon Jovi 4. Design a static method that traverses through the array and prints each element. 5. Since the data will need to be sorted prior to conducting a binary search, three static methods to do so need to be created. a. Write static methods to sort by title, year, and artist. You may use the insertion, selection, or merge sort, but not a bubble sort. b. Name and document the methods to clearly indicate the type of sort and the values being sorted. c. Utilize print debugging statements to ensure the sorts worked. Be sure to comment these out prior to submitting your work. 6. Create the following static methods in the tester class. Utilize the binary search algorithm. Each method will take two arguments: the array and the value to find. a. a method that searches the array for a particular song title b. a method that searches the array for year released. The output should list all songs found from that year c. a method that searches the array for the name of the artist. The output should list all songs performed by that artist d. methods to assist with printing all matches after a binary search has found a match. Model your code after the linearPrint method sample 7. Test your search methods by calling each and displaying the results. Start by showing the original array. Then demonstrate searching for a title, showing results when a title is found and when not found. Do the same for year and artist. Include searches that should find more than one match. Be sure to clearly label your output so someone looking at it knows which search criterion was applied each timeStep by Step Solution
There are 3 Steps involved in it
Step: 1
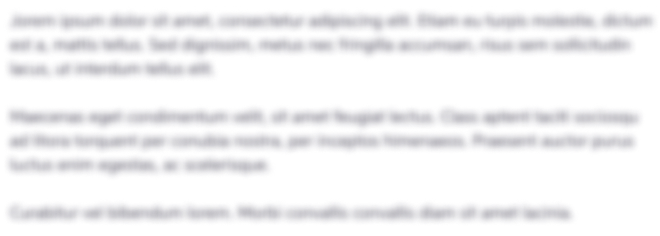
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started