Question
Must be done in C++ Problems 1.The Right Cylinder Glass Company manufactures only one product: a perfectly cylindrical blue drinking glass. All glasses are custom-made,
Must be done in C++
Problems
1.The Right Cylinder Glass Company manufactures only one product: a perfectly cylindrical blue drinking glass. All glasses are custom-made, according to a customer's individual preferences in size and thickness.
In the past few months, however, RCGC has been losing money due to flaws in their manufacturing process. It seems they just can't manage to produce the right of amount of glass to properly fill an order. It's always either too much glass or too little, and both of these cost money.
RCGC has hired you to solve this problem. Your job is to write a program, glassvoli, which computes the amount of glass, in cubic inches, necessary to fill a given order. Each time a user runs your software, they should be prompted for the height, width, and wall-thickness of each glass (given in inches, to the nearest 1/100th of an inch), and the number of glasses in the order (an integer, obviously).
You can assume that each time a user runs your software, she will supply the height, width, and wall-thickness of each glass (given in inches, to the nearest 1/100th of an inch), and the number of glasses in the order (an integer, obviously), in that order. This is sufficient information to compute the answer.
For example (user input is in italics and underlined):
$ ./glassvoli How tall is each cup? 8.2 How wide? 2.2 How thick? 0.1 How many cups in this order? 1000 You will need 5723.98 cubic inches of glass to fill this order
2.Now make a version of your solution to Problem #1 that uses only command-line arguments. For this program, glassvol, you can assume that each time a user runs your software, she will supply the height, width, and wall-thickness of each glass (given in inches, to the nearest 1/100th of an inch), and the number of glasses in the order (an integer, obviously), in that order. This is sufficient information to compute the answer.
Example use:
$ ./glassvol 8.2 2.2 0.1 1000 You will need 5723.98 cubic inches of glass to fill this order.
3.Compound interest is basic model for the growth of investments and also debt. Online calculators for both are common (for example, https://www.investor.gov/financial-tools-calculators/calculators/compound-interest-calculator). For this problem, write a simple version of a compound interest calculator, compound, which takes three command-line arguments, representing (in order) an annual deposit into a savings account, a monthly deposit amount, and an annual percentage rate (expressed as percentage: for example, 2.4% would be given as 2.4). The program should calculate an annual compounding of interest on the total balance (which, for simplicity, takes places before that year's monthly contributions are added in). The program should print the value of this investment after 7 years have passed.
Example uses:
$ ./compound 5000 100 2.3 $14865.06 $ ./compound 5000 -100 15 $19.94
NOTE #1: The choice of when to apply the annual interest compounding vs the 12 monthly contributions will lead to subtle changes. Try to match your program's output against the calculator linked above.
NOTE #2: You can control the formatting of the floating-point values using the setprecision()and fixed operators:
cout << fixed << setprecision(2);
4.Write a program, weekday, which will tell you the day of the week on which a date occurred. Specifically, your program whould take three integer arguments representing a date. The arguments should be given in the order year month day. The program should print a single integer: 0 for Sunday, 1 for Monday, and so on, up to 6 for Saturday.
Example Uses:
$ ./weekday 2023 2 7 2 $ ./weekday 2023 2 3 5 $ ./weekday 2022 2 3 4 $ ./weekday 2022 12 31 6
How to solve this:
There's a set of "magic formulae" for solving this problem. Let's assume we already have the values m (month), d (day) and y (year). Then the weekday for m/d/y (which we'll denote by w) can be found by calculating the following values:
yZ = y - ((14 - m) / 12)
(If the month is January or February, we'll consider them as the 13th or 14th months of the previous year)
mZ = m + (12 * ((14 - m) / 12))
(This keeps the values for March through December at 3 .. 12. January becomes 13 and February becomes 14)
B = yZ % 100
(Years since the century began: i.e., the last two digits of y)
C = yZ / 100
(The century: i.e., the first two digits of y)
leapDays = (B / 4) + (C / 4) + 5*C
(The total number of leap days that have occurred since 0 C.E.)
w = (d + ( ((mZ+1) * 26) / 10) + B + leapDays + 6) % 7
All of the divisions above are integer divisions (i.e. with the fractional parts discarded). Hence any variables you use should be of int type (if you use double values, the formulae won't be quite right).
This formula, known as "Zeller's Congruence", is due to Julius Christian Johannes Zeller, a German mathematician of the 19th century. Although opaque, it is possible, with a little work, to understand how he found it. For the curious, the Wikipedia entry is quite nice: http://en.wikipedia.org/ wiki/Zeller_congruence
Let's start with the data types you'll need. You're working with remainders and integer division, so the best choice is clearly int. So we'll start out the program as
int main(int argc, char *argv[]) { if (argc < 4) { cout << "USAGE: " << argv[0] << "" << " "; exit(1); } int y = stod(argv[1]); int m = stod(argv[2]); int d = stod(argv[3]);
Finish the rest.
5.Write a program that determines the distance between two locations on the globe. The user will enter first the names of these locations as well as the the latitude and longitude of each (in decimal degrees, not radians). Your program will compute and display the orthdromic ("great circle") distance between these points.
This program will have a command-line interface.
Example interactions:
$ ./geodistance Perth -31.95224 115.8614 Hamilton 43.25011 -79.84963 It is 18171.7 kilometers (11291.3 miles) from Perth to Hamilton. $ ./geodistance Ithaca 42.443962 -76.501884 "New York City" 40.728333 -73.994167 It is 282.9 kilometers (175.8 miles) from Ithaca to New York City. $ ./geodistance Toronto 43.651070 -79.347015 Chicago 41.881832 -87.623177 It is 704.1 kilometers (437.5 miles) from Toronto to Chicago.
How to solve this
First, a word about the arguments. You can get the latitude & longitude of any city by looking it up on Wikipedia. You'll see a "coordinates" link at the top right of the page. Click on this, and from the resulting page, you'll see the city's coordinates in several formats. Use the "decimal" format (top of the Geohack page), specifically, the version that uses fractional values rather than degrees/minutes/seconds. Try looking up Toronto and Chicago, and match what you see against the example above.
Now for the program. Begin by getting the correct reading of input values done. Once you have this, set up a mostly-working "print" statement, which is as close as you can get it to the expected output format. What quantities can you compute with just the input? What quantities do you still need to figure out? Fill those in with appropriate variables, but don't worry about getting their values right just yet. Instead, just make sure you can compile and run the program in its "half-solution" state.
This is an example of isolating and delaying concerns.
Now for the messy parts. You need to know: the conversion between miles and kilometers, the radius of the globe you're computing the distance on (i.e. the Earth), and a formula for computing this distance (we're working with a globe, not straight-line distance!)
The most important component is to learn the general formula for computing the distance between two points on a sphere (the "great circle distance"): for points (plat,plon) and (qlat, qlon), the distance on a sphere with radius r is
(Don't over-think this. You're not being asked to come up with a formula! Your only job here is to write code that expresses the formula above.)
While the Earth is neither a true sphere, nor possessed of a perfectly uniform surface, let's ignore that for our present work: assume a perfect sphere with radius of 6378 kilometers.
Do some internet research to figure out how to convert kilometers to miles.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
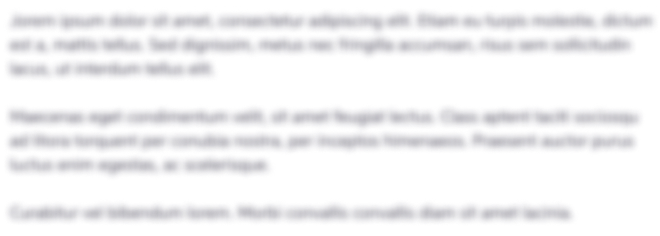
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started