Question
must be in java: a) ( already completed for you ) Swap the first and last elements in the array list. b) Shift all elements
must be in java:
a) (already completed for you) Swap the first and last elements in the array list.
b) Shift all elements by one to the right and move the last element into the first position. For example,
1 4 9 16 25 would become 25 1 4 9 16.
c) Replace all even elements with 0.
d) Replace each element except the first and last by the larger of its two neighbors. For the example in part (b), your output would be 1 9 16 25 25.
e) Remove the middle element if the array list size is odd, or the middle two elements if the length is even. (You will need to test two different array lists here to make sure your method works on both even and odd length array lists).
f) Move all even elements to the front, otherwise preserving the order of the elements. For the example in part (b) your output should be 4 16 1 9 25
g) Return the second-largest element in the array list. (Your method should return this value, and your main method should print the value out). If your input is 3 25 50 50 22 note that 50 should be returned. 50 is both the largest and second largest value in this array list because there are 2 elements with value 50.
h) Return true if the array list is currently sorted in increasing order. (You can create a sorted ArrayList to use as input by starting with an empty Array List. Then use the add() method to add values in order one at a time). (Alternately you can use Collections.sort(); to sort your list. Use your ArrayList as a parameter to the sort() method). Make sure to test both a sorted array list and an unsorted array list as input. Your main method should print whether the array was in order or not.
i) Return true if the array list contains two adjacent duplicate elements.
j) Return true if the array list contains duplicate elements (which need not be adjacent)
starter code:
import java.util.Collections; //need this one for sorting ArrayList import java.util.Random; import java.util.ArrayList; public class ArrayListMethods { //part a, done for you. public static void swapFirstAndLast(ArrayLista) { //save first element int temp = a.get(0); //move last element to first position (0) a.set(0, a.get(a.size()-1)); //put temp value into last position (size() -1) a.set(a.size()-1, temp); } //part b, fill in this method public static void shiftRight(ArrayList a) { } //part c, set all even elements to 0. public static void setEvensToZero(ArrayList a) { } //part d, replace each element except the first and last by larger of two //around it public static void largerOfAdjacents(ArrayList a) { } //part e, remove middle el if odd length, else remove middle two els. public static void removeMiddle(ArrayList a) { } //part f - move all evens to front public static void moveEvensToFront(ArrayList a) { } //part g - return second largest el in array public static int ret2ndLargest(ArrayList a) { return 0; //dummy value } //part H - returns true if array is sorted in increasing order public static boolean isSorted(ArrayList a) { boolean isSorted = false; //initially assume list is not sorted return isSorted; //dummy value } //PART I - return true if array contains 2 adjacent duplicate values public static boolean hasAdjDuplicates(ArrayList a) { boolean hasDup = false; //assume no duplicates, then search array return hasDup; } //PART J - return true if array contains 2 duplicate values //duplicates need not be adjacent to return true public static boolean hasDuplicates(ArrayList a) { boolean hasDup = false; //assume no duplicates, then search array return hasDup; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
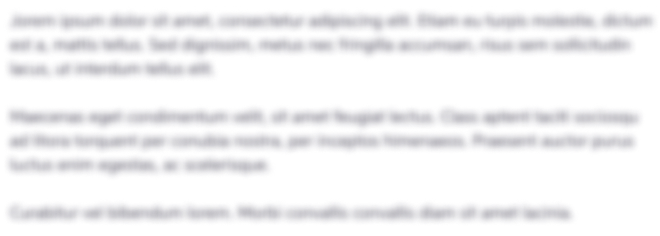
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started