Question
My code crashed. Need help correcting. def get_weather_report(takeoff,weather): Returns the most recent weather report at or before take-off. The weather is a dictionary whose
My code crashed. Need help correcting.
def get_weather_report(takeoff,weather):
"""
Returns the most recent weather report at or before take-off.
The weather is a dictionary whose keys are ISO formatted timestamps and whose values
are weather reports.For example, here is an example of a (small portion of) a
weather dictionary:
{
"2017-04-21T08:00:00-04:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 13.0,
"crosswind": 2.0,
"units": "KT"
},
"temperature": {
"value": 13.9,
"units": "C"
},
"sky": [
{
"cover": "clouds",
"type": "broken",
"height": 700.0,
"units": "FT"
}
],
"code": "201704211056Z"
},
"2017-04-21T07:00:00-04:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 13.0,
"crosswind": 2.0,
"units": "KT"
},
"temperature": {
"value": 13.9,
"units": "C"
},
"sky": [
{
"type": "overcast",
"height": 700.0,
"units": "FT"
}
],
"code": "201704210956Z"
}
...
},
If there is a report whose timestamp matches the ISO representation of takeoff,
this function uses that report.Otherwise it searches the dictionary for the most
recent report before takeoff.If there is no such report, it returns None.
Example: If takeoff was as 8 am on April 21, 2017 (Eastern), this function returns
the value for key '2017-04-21T08:00:00-04:00'.If there is no additional report at
9 am, a 9 am takeoff would use this value as well.
Parameter takeoff: The takeoff time
Precondition: takeoff is a datetime object
Paramater weather: The weather report dictionary
Precondition: weather is a dictionary formatted as described above
"""
# HINT: Looping through the dictionary is VERY slow because it is so large
# You should convert the takeoff time to an ISO string and search for that first.
# Only loop through the dictionary as a back-up if that fails.
# Search for time in dictionary
# As fall back, find the closest time before takeoff
# STEP 1 : Convert the date time to ISO string
iso_string_date = takeoff.isoformat()
# STEP 2: Return the weather data for the iso date we have found.
return weather[iso_string_date]
The call get_weather_report(2017-10-12T11:30:00-04:00,weather) crashed. Traceback (most recent call last): File "/home/codio/workspace/.guides/tests/step3/verifier.py", line 546, in grade_func4 found = func(stamp,report) File "/home/codio/workspace/auditor/violations.py", line 341, in get_weather_report return weather[iso_string_date] KeyError: '2017-10-12T11:30:00-04:00'
__________________________________________________________________________________________________________________________________
Test file.
def test_get_weather_report():
"""
Tests the function get_weather_report
"""
fcn = 'violations.get_weather_report'
parent = os.path.split(__file__)[0]
fpath= os.path.join(parent,'weather.json')
report = utils.read_json(fpath)
tests = [("2017-10-12T11:00:00-04:00","2017-10-12T11:00:00-04:00"),
("2017-10-12T11:30:00-04:00","2017-10-12T11:00:00-04:00"),
("2017-10-13T09:00:00-04:00","2017-10-13T09:00:00-04:00"),
("2017-10-13T09:15:00-04:00","2017-10-13T09:00:00-04:00"),
("2017-03-12T02:00:00-05:00","2017-03-12T02:00:00-05:00"),
("2017-03-12T02:45:00-05:00","2017-03-12T02:00:00-05:00"),
("2017-03-12T03:00:00-05:00","2017-03-12T02:00:00-05:00"),
("2017-12-27T08:00:00-05:00","2017-12-27T08:00:00-05:00"),
("2017-12-27T23:00:00-05:00","2017-12-28T00:00:00-05:00")]
# Perform the tests
for test in tests:
expct = report[test[1]]
stamp = utils.str_to_time(test[0])
found = violations.get_weather_report(stamp,report)
try:
code = 'code='+repr(found['code'])
except:
code = 'no code'
data= (fcn,test[0],'weather',code,repr(expct['code']))
assert_equals(expct, found,'%s(%s,%s) returned a report with %s, not code=%s' % data)
print('%s passed all tests' % fcn)
_______________________________________________________________________________________________________________________________________
utils file
# not sure if you need this to run the test file.
import json
def read_json(filename):
"""
Returns the contents read from the JSON file filename.
This function reads the contents of the file filename, and will use the json module
to covert these contents in to a Python data value.This value will either be a
a dictionary or a list.
Parameter filename: The file to read
Precondition: filename is a string, referring to a file that exists, and that file
is a valid JSON file
"""
#opening the file
fp=open(filename)
#converts the json file to dictionary
data=json.load(fp)
#closing the file
fp.close()
#return dictionary
return data
import pytz
from dateutil.parser import *
import datetime
from pytz import *
def str_to_time(timestamp,tz=None):
"""
Returns the datetime object for the given timestamp (or None if stamp is invalid)
This function should just use the parse function in dateutil.parser to
convert the timestamp to a datetime object.If it is not a valid date (so
the parser crashes), this function should return None.
If the timestamp has a timezone, then it should keep that timezone even if
the value for tz is not None.Otherwise, if timestamp has no timezone and
tz if not None, this this function will assign that timezone to the datetime
object.
The value for tz can either be a string or a time OFFSET. If it is a string,
it will be the name of a timezone, and it should localize the timestamp. If
it is an offset, that offset should be assigned to the datetime object.
Parameter timestamp: The time stamp to convert
Precondition: timestamp is a string
Parameter tz: The timezone to use (OPTIONAL)
Precondition: tz is either None, a string naming a valid time zone,
or a time zone OFFSET.
"""
# HINT: Use the code from the previous exercise and update the timezone
# Use localize if timezone is a string; otherwise replace the timezone if not None
try:
result = parse(timestamp)
if result.tzinfo is None and tz is None:
return result
elif result.tzinfo is None and type(tz) == str:
time1 = pytz.timezone(tz)
e = time1.localize(result)
return e
elif result.tzinfo is not None and tz is None:
return result
elif result.tzinfo is None and tz is not None:
g = result.replace(tzinfo=tz)
return g
elif result.tzinfo is not None and tz is not None:
h = result.replace()
return h
except ValueError:
return None
____________________________________________________________________________________________________________________________________________
Part of the weather.json file (it is an extremely hard file)
{
"2018-01-01T00:00:00-05:00": {
"visibility": {
"prevailing": 1.75,
"units": "SM"
},
"wind": {
"speed": 13.0,
"crosswind": 5.0,
"units": "KT"
},
"temperature": {
"value": -15.0,
"units": "C"
},
"sky": [
{
"cover": "clouds",
"type": "broken",
"height": 1200.0,
"units": "FT"
},
{
"type": "overcast",
"height": 1800.0,
"units": "FT"
}
],
"weather": [
"light snow",
"mist"
],
"code": "201801010456Z"
},
"2017-12-31T23:00:00-05:00": {
"visibility": {
"prevailing": 1.75,
"units": "SM"
},
"wind": {
"speed": 13.0,
"crosswind": 5.0,
"units": "KT"
},
"temperature": {
"value": -15.0,
"units": "C"
},
"sky": [
{
"cover": "clouds",
"type": "broken",
"height": 1300.0,
"units": "FT"
},
{
"type": "overcast",
"height": 2200.0,
"units": "FT"
}
],
"weather": [
"light snow",
"mist"
],
"code": "201801010356Z"
},
"2017-12-31T22:00:00-05:00": {
"visibility": {
"prevailing": 3.0,
"units": "SM"
},
"wind": {
"speed": 11.0,
"crosswind": 7.0,
"units": "KT"
},
"temperature": {
"value": -15.0,
"units": "C"
},
"sky": [
{
"type": "overcast",
"height": 1300.0,
"units": "FT"
}
],
"weather": [
"light snow",
"mist"
],
"code": "201801010317Z"
},
"2017-12-31T21:00:00-05:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 10.0,
"crosswind": 7.0,
"units": "KT"
},
"temperature": {
"value": -16.1,
"units": "C"
},
"sky": [
{
"type": "overcast",
"height": 1700.0,
"units": "FT"
}
],
"code": "201801010156Z"
},
"2017-12-31T20:00:00-05:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 11.0,
"crosswind": 10.0,
"units": "KT"
},
"temperature": {
"value": -16.7,
"units": "C"
},
"sky": [
{
"cover": "clouds",
"type": "broken",
"height": 2000.0,
"units": "FT"
}
],
"code": "201801010056Z"
},
"2017-12-31T19:00:00-05:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 12.0,
"crosswind": 9.0,
"gusts": 19.0,
"units": "KT"
},
"temperature": {
"value": -16.1,
"units": "C"
},
"sky": [
{
"cover": "clouds",
"type": "broken",
"height": 2200.0,
"units": "FT"
}
],
"code": "201712312356Z"
},
"2017-12-31T18:00:00-05:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 12.0,
"crosswind": 8.0,
"units": "KT"
},
"temperature": {
"value": -16.1,
"units": "C"
},
"sky": [
{
"cover": "clouds",
"type": "broken",
"height": 2200.0,
"units": "FT"
}
],
"code": "201712312256Z"
},
"2017-12-31T17:00:00-05:00": {
"visibility": {
"prevailing": 10.0,
"units": "SM"
},
"wind": {
"speed": 11.0,
"crosswind": 9.0,
"units": "KT"
},
"temperature": {
"value": -16.0,
"units": "C"
},
"sky": [
{
"type": "overcast",
"height": 2200.0,
"units": "FT"
}
],
"code": "201712312214Z"
},
Step by Step Solution
There are 3 Steps involved in it
Step: 1
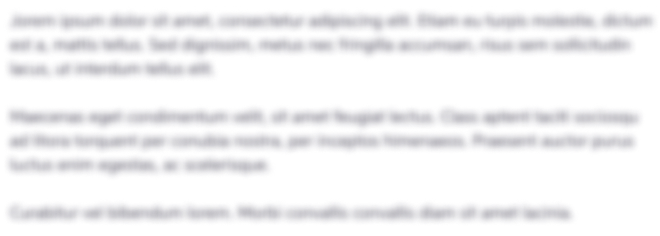
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started