Question
My professor has given us the assignment below and I just need help filling out this CRC Card he's given us as part of the
My professor has given us the assignment below and I just need help filling out this CRC Card he's given us as part of the assignment!
1 Overview
This programming assignment requires creating and test a sorted linked list data structure for holding a list of users. The users in this list structure are sorted based on the increasing order of their date of birth.
2 Requirements
2.1 (Class) Implement DateType class provided in the case study in Chapter 2 Create the specification file (DateType.h) and the implementation file (DateType.cpp) (the codes of these 2 files are also listed on chapter 2 slide 32 and 33). This class must have all data attribute and functions as in the case study.
2.2 (Class) Implement a User class. Create the specification file (User.h) and implementation file (User.cpp). User class has the following attributes: char firstName[7]; char lastName[7]; and dateOfBirth as DateType. The following constructor and functions must be implemented in the User class:
- Default constructor User()
- Void Initialize( char firstName[7], char lastName[7], DateType date): this function is to initialize a user object with given values.
- RelationType comparedTo(User* aUser) const: this function compare the self user with aUser and return a ReliationType value. It must call the CompareTo(DateType aDate) function of the DateType class (see case study in chapter 2). The rule of comparison and returning values is as follow:
- Returns LESS: if CompareTo(DateType aDate) returns LESS
- Return GREATER: if CompareTo(DateType aDate) returns GREATER
- Return EQUAL: if two users have same firstName and lastName and CompareTo(DateType aDate) returns EQUAL
(Note that the RelationType is defined as an enum: enum RelationType{LESS, EQUAL, GREATER} )
- string ToString(): this function returns a string that contains all information of the user (firstName, lastName, and dateOfBirth).
2.3 (Class) Implement SortedUserType as a class sorted user objects. Create and implement the specification file (SortedUserType.h) and implementation file (SortedUserType.cpp). The SortedUserType class uses NodeUser struct to create a node of a user in the list. For testing purposes, SortedUserType can store a maximum of 5 users (maxList). The user object will be inserted based on the increasing order of the users dateOfBirth.
2.4 (Struct) Implement NodeUser struct in the SortedUserType.h and SortedUserType.cpp.
NodeUser has two fields:
User userInfo; // data info
NodeUser* next; // pointer
2.5 (SortedUserType operations) the SortedUserType class has the following operation:
Normal operators:
- (Constructor) SortedUserType ();
- (Transformer) void ResetList();
- (Transformer) void MakeEmpty();// delete all item in the list
- (Observer) bool IsFull() const;
- (Observer) int GetLength() const;
Pointer related operators:
- (Observer) User* GetUser(User* aUser, bool& found) const; // get a user from the list whose firstName, lastName, and dateOfBirth match with aUser.
- (Transformer) void PutUser(User* aUser); // put aUser in to the list according to dateOfBirth order.
- (Transformer) void DeleteUser(User* aUser); // delete a user from the list whose firstName, lastName, and dateOfBirth match with aUser.
- (Observer) User* GetNextUser(); // get the next user in the list
Note that:
* All functions must follow the algorithms of sorted list ADT
** GetUser, PutUser, and DeleteUser functions must use the comparedTo(User* aUser) function of the User class.
***GetUser(User* aUser, bool& found) must search for the user based on firstName, lastName, and dateOfBirth information. It must follow the algorithm of getting an item in a sorted list ADT. The best way to do this is to call comparedTo(User* aUser) function of the User class to determine the match of two user objects. If the current item does not match, go the next item on the list.
***PutUser(User* aUser) must put aUser into the list based on dateOfBirth order. If the new user has the same dateOfBirth, firstName, and lastName with a user in the list, it cannot be put into the list. Again, calling comparedTo(User* aUser) function is the best way to handle this requirement.
***DeleteUser function only can delete a user from the list whose firstName, lastName, and dateOfBirth match with aUser. Again, calling comparedTo(User* aUser) function is the best way to handle this requirement.
2.6 (TestDriver) Implement the test program (TestDriver.cpp). This program must have
- SortedUserType useList: all users in the input file will be inserted in this object
- void PrintNextUser(ofstream& outFile, SortedUserType list): this function writes the current user, at the location of the item accessed by GetNextUser() function. This function must call ToString() function of the Use class.
- void PrintList(ofstream& outFile, SortedUserType list): this function writes all items in the list to the output file. This function must use the GetNextItem() function of the SortedUserType class and it must call the ToString() function of the User class.
- The TestDriver program must test all functions of the SortedUserType listed above.
- Note: if you want, you can change the signatures of PrintNextUser and/or PrintList to pass in references to the output file and the considered list: ofstream& outFile, SortedUserType &list
The input file must contain the following contents:
GetLength PutUser Gary Bryan 09 16 2001 PutUser Andres Lynch 10 06 2002 PutUser Wendy Holt 11 23 2000 PutUser Annie Pratt 04 25 2003 PrintList PutUser Gary Bryan 09 16 2001 PutUser Grant Conner 10 16 2002 PrintList GetUser Andres Lynch 10 06 2002 GetUser Lynda Wells 10 21 1999 PrintNextUser ResetList IsFull DeleteUser Kyla Howard 11 06 2000 IsFull DeleteUser Wendy Holt 11 23 2000 PrintList MakeEmpty PrintList Quit
Note: Your instructor is not your tester. You should develop a testing plan and test every function related to SortedUserType class (the class itself and other associated classes). Error detected during grading will result in loss of points. Make sure that you test your program carefully.
2.7 Commenting Code
- Your name must be included at the beginning of every code file.
- Your code should be commented well. Each function should have preconditions and postconditions listed after the function declaration in the implementation files.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
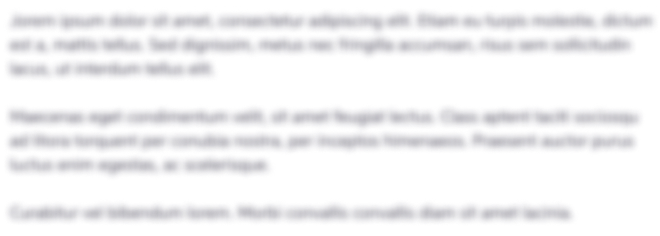
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started