Question
---->My Question how to code a java program showing of polymorphism of 5 different animal classes printining their sounds the istruction of the program is
---->My Question how to code a java program showing of polymorphism of 5 different animal classes printining their sounds
the istruction of the program is stated here below. I have also tried and write my code below the instruction. I tried my best but it can't combile.
Step 1 Constructors and overloading
Add an initialize method to your CDog class and call the set methods from inside the procedure.
public void Initialize( String strName, int intAge, float sngWeight )
Add the following default constructor and parameterized constructors. Call the Initialize method from the previous step inside the constructors.
public CDog( )
public CDog( String strName )
public CDog( String strName, int intAge )
public CDog( String strName, int intAge, float sngWeight )
Add a Print method to your CDog class that prints out all the properties in the class and calls each method.
Make a Step1 procedure in CHomework7b and call it from main. In the Step1 procedure make four different instances of the CDog class each using a different constructor. Call the Print method from the previous step to show the parameterized constructors correctly initialized each instance.
You have now successfully implemented constructors and overloading.
Step 4 Inheritance and super
Create a derived class CTrainedDog that inherits the base class CDog.
Add at least one new private property (e.g. Breed) with public get/set methods. Include some sort of boundary checking in the set method.
Add at least two new methods (e.g. PlayDead). Include some sort of centralized business logic that uses one or more of the properties in either the CDog class or the CTrainedDog class to produce different results.
Add an initialize method to your CTrainedDog class that takes a value for the new property you created in the previous step. Call the set methods from inside the procedure.
public void Initialize(
Add the following constructors:
Add a default constructor. Call super( ) with no parameters to call the default constructor from the parent CDog class.
Add three parameterized constructors that mirror the three parameterized constructors in CDog. Call the super( ) method with the appropriate parameters.
Add one parameterized constructors that take four parameters. It should take name, age, weight and a value for the new trained dog property. Call super( ) with the correct parameters and then call the initialize method with the trained dog property.
Add a Print method to your CTrainedDog class that prints out all the properties in the class and calls each method.
Make a Step2 procedure in CHomework7b and call it from main. In the Step2 procedure make five different instances of the CTrainedDog class each using a different constructor. Call the Print method from the previous step to show the parameterized constructors correctly initialized each instance.
You have now successfully implemented inheritance and super.
here is my code below
// ------------------------------------------------------------------------------------------
// Name: Tesfu Gebremariam
// Class: Java 1
// Abstract: Homework 7 - Classes, classes and more classes
// ------------------------------------------------------------------------------------------
// ------------------------------------------------------------------------------------------
// Imports
// ------------------------------------------------------------------------------------------
public class CHomework7
{
// --------------------------------------------------------------------------------
// Name: main
// Abstract: This is where the program starts.
// --------------------------------------------------------------------------------
private String m_strName = "";
private String m_strType = "";
// ----------------------------------------------------------------------
// Name:
// Abstract:
// ----------------------------------------------------------------------
//Set the name
public void SetName( String strNewName )
{
int intLength = 0;
intLength = strNewName.length( );
//Too Long?
if ( intLength > 50 )
{
//if longer, clip to 5o characters
intLength = 50;
}
m_strName = strNewName.substring ( 0, intLength );
}
//Get the name
public String GetName( )
{
return m_strName;
}
public void SetType ( String strNewType )
{
//Negative?
int intLength = 0;
intLength = strNewType.length ( ) ;
//Too Long?
if ( intLength > 50 )
{
//if longer, clip to 5o characters
intLength = 50;
}
m_strType = strNewType.substring ( 0, intLength );
}
//Get the name
public String GetType( )
{
return m_strType;
}
public void MakeNoise ( )
{
System.out.println( "" );
}
public void SetWeight( float sngNewWeight )
{
//If it is Nagative
if( sngNewWeight < 0 )
{
//Yes, clip it
sngNewWeight=0.0f;
}
m_sngWeight=sngNewWeight;
}
//--------------------------------
//Name: GetWeight
//Abstract: get the weight
//---------------------------------
public float GetWeight()
{
return m_sngWeight;
}
public void Bark()
{
// Big dog
if(GetWeight() < 15 )
{ // No ankle biter
System.out.print(" Yip, Yip, Yip" );
}
else
{
//if yes
System.out.println(" woof, woof" );
}
}
public void SetColor( String strNewColor)
{
int intStopIndex = 0;
// Brown by defualt
if(strNewColor.equals( "" ) == true) strNewColor= " Brown";
//Too long?
intStopIndex= strNewColor.length();
if(intStopIndex > 10 )
{
// yes, clip it to 10 characters
intStopIndex=0;
}
m_strColor= strNewColor. substring( 0, intStopIndex);
}
//-------------------------------
//Color:GetColor
//Abstract: Get the color
public String GetColor()
{
return m_strColor;
}
//------------------
// Name: Graze
// Abstract: Get the color
public void Graize()
{
System.out.println(" Mmmmmm, this is some tasty grass!");
}
//-----------------------
//Name :MakeNoise
// Abstract: Make Noise
//-----------------
public void MakeNoise()
{
System.out.println(" moo" );
}
// -----------------------------------
//-Name: MakeNoise
//Abstract: Make Noise
public void BreatheFire()
{
int intIndex = 0;
// one head
if( GetHeadCount()== 1 )
{
// Yes
System.out.println(" The dragon breathes fire.");
}
else
{
System.out.println(" The dragon breathes from each of its " + GetHeadCount() + " heads:" );
}
for( intIndex = 0; intIndex < GetHeadCount(); intIndex + = 1)
{
System.out.println("***Breathe Fire***" );
}
}
}
//---------------------
// Name: SetBreed
// Abstract: set the breed
//--------------------------
public void SetBreed( String strNewBreed )
{
int intStopIndex =0;
int intStopIndex = strNewBreed.length();
// Too long
if( intStopIndex > 10 )
{
// Yes, clip to 10 character
intStopIndex=10;
}
m_strNewBreed= strNewBreed.substring( 0, intStopIndex );
}
//---------------------
// Name: GetBreed
// Abstract: Get the breed
//--------------------------
public String GetBreed()
{
return m_strBreed;
}
//---------------------
// Name:PlayDead
// Abstract: Play Dead ..... may be.
//--------------------------
public void PlayDead()
{
// Young dog?
if( GetAge() < 5 )
{ // Yes
System.out.println(" Bang! oh, you got me. " );
}
else
{
// No ,can't teach an old dog new tricks
System.out.println("Treat Fist. Trick second. " );
}
}
//---------------------
// Name:FetchNewapaper
// Abstract: Fetch a newspaper
//--------------------------
public void FetchNewspaper()
{
//Young dog
if( GetAge() < 2)
{
//Yes
System.out.println(" I am too young. ");
}
else
{
//No
System.out.println(" Here is your paper." );
}
}
public static void main( String astrCommandLine[] )
{
//call ShowPolymorphism
// --------------------------------------------------------------------------------
// Name: ShowPolymorphism
// Abstract: Show Polymorphism
// --------------------------------------------------------------------------------
public static void ShowPolymorphism( )
{
//declare intIndex, strAnimalType
int intIndex=0;
private String m_strName = "";
private String m_strType = "";
//System.out.println( "Polymorphism - Let's go to the zoo" );
//System.out.println( "----------------------------------------" );
// Make zoo array - make sure it can hold all your animals! (Hint: You need a size in the line below)
CAnimal aclsZoo[] = new CAnimal[ 7 ];
// Populate zoo - Polymorphism example
// Create Dog
CDog clsBuster = new CDog( );
clsBuster.SetName( "Buster" );
clsBuster.SetType ( "Dog" );
clsBuster.SetAge ( 11 );
clsBuster.SetWeight ( 40 );
// Create Cat
CCat clsmitu = new CCat( );
clsmitu.SetName( "mitu" );
clsmitu.SetType ( "Cat" );
clsmitu.SetAge ( 8 );
clsmitu.SetWeight ( 10 );
// Create Duck
CDuck clsDerhomai = new CDuck( );
clsDerhomai.SetName( " Derhomai" );
clsDerhomai.SetType ( "Duck" );
clsDerhomai.SetAge ( 9 );
clsDerhomai.SetWeight ( 40 );
// Create Cow
CCow clsAshkeri = new CCow( );
clsAshkeri.SetName( "Ashkeri" );
clsAshkeri.SetType ( "Cow" );
clsAshkeri.SetAge ( 10 );
clsAshkeri.SetWeight ( 100 );
//Create Dragon
CDragon clsHurri = new CDragon( );
clsHurri.SetName( "Hurri" );
clsHurri.SetType ( "Dragon" );
clsHurri.SetAge ( 12 );
clsHurri.SetWeight ( 70);
//Create Dragon
CGoat clsHurri = new CGoat( );
clsWerho.SetName( "Werho" );
clsWerho.SetType ( "Goat" );
clsWerho.SetAge ( 12 );
clsWerho.SetWeight ( 70);
clsHurri.SetHeadCount( 3 );
// Create Trained Dog
CTrainedDog clsFifi = new CTrainedDog( );
CTrainedDog clsFifi=new CTrainedDog();
clsFifi.SetName( "Fifi" );
clsFifi.SetType ( "TrainedDog" );
clsFifi.SetAge ( 2 );
clsFifi.SetWeight ( 10 );
clsFifi.SetBreed ( "Poodle" );
// Populate zoo array with Animal
CAnimal aclsZoo[ ] = new CAnimal [ 9 ];
aclsZoo [ 0 ] = (CAnimal) clsBuster;
aclsZoo [ 1 ] = (CAnimal) mitu;
aclsZoo [ 2 ] =(CAnimal) clsDerhomai;
aclsZoo [ 3 ] = null;
aclsZoo [ 4 ] = (CAnimal) clsAshkeri;
aclsZoo [ 5 ] = (CAnimal) Hurri;
aclsZoo [ 6 ] = (CAnimal) clsFifi;
// Display zoo animals - Polymorphism example
for( intIndex = 0; intIndex < aclsZoo.length; intIndex += 1 )
{
// Is there an animal in the cage?
if( aclsZoo[ intIndex ] != null )
{
// Polymorphism example
// print Animal in cage #
System.out.println( "Animal in Cage #" + ( intIndex + 1) );
// print Animal name using GetName
System.out.println( "Name: " + aclsZoo[ intIndex ].GetName( ) );
// print Animal type using GetType
System.out.println(" Type: " + aclsZoo[ intIndex ].GetType());
// execute MakeNoise () since it will print the noise
aclsZoo[ intIndex ].MakeNoise();
System.out.println("");
}
}
}
}
// populate strAnimalType using GetType so you can execute methods specific to the animal
// If this is type Dog, call the Fetch method
if( strAnimalType.equals( "Dog" ) == true )
{
// Order we want:
// 1) Get array element
// 2) explicit cast
// 3) call child-specific method
( (CDog) aclsZoo[ intIndex ] ).Fetch( );
}
// If this is type Trained Dog, call PlayDead method and Print method
else if( strAnimalType.equals( "Trained Dog" ) == true )
{
// execute PlayDead
(( CDog) aclsZoo[ intIndex ] ).Fetch();
}
// execute Print (or other method specific to this animal)
else if( strAnimalType.equals( "trained Dog" )==true
{
(( CTrainedDog) aclsZoo[ intIndex ] ).PlayDead();
(( CTrainedDog) aclsZoo[ intIndex ] ).Print();
}
// If this is a Cow, call Graze method and print the color
else if( strAnimalType.equals(" Cow" )==true )
{
// execute Graze method
(( CCow) aclsZoo[ intIndex ] ).Graze();
// print using by using the GetColor method
System.out.println(" Color is " + (( CCow) aclsZooo[ intIndex ] ).GetColor() );
}
// If this is a Dragon, call BreatheFire method
else ( strAnimalType.equals( "Dragon" )==true )
{
//execute BreatheFire method
(( CDragon) aclsZoo[ intIndex ] ).BreatheFire();
}
System.out.println( "" );
}
*****The Classes of animals I form are CCat, CCow, CDog, CDuck, and CGoat
Step by Step Solution
There are 3 Steps involved in it
Step: 1
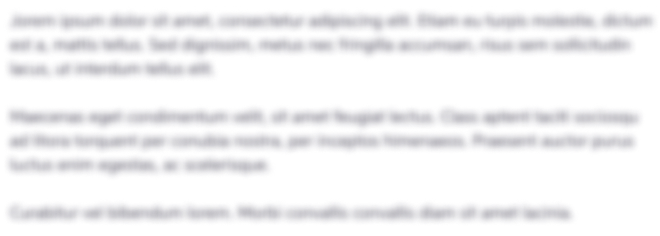
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started