Question
myRandom.java import java.io.FileWriter; import java.io.IOException; import java.io.Writer; import java.util.Random; // In this class you should implement the Xorshift* PRNG function // (See lecture notes if
myRandom.java
import java.io.FileWriter; import java.io.IOException; import java.io.Writer; import java.util.Random;
// In this class you should implement the Xorshift* PRNG function // (See lecture notes if you're unsure how that works) public class myRandom { // This is the internal state variable long state; // Constructor - In this function, you should set the internal state // to the value of the input integer seed public myRandom(long seed) { // TODO: Fill this in } // getRand() should return a pseudorandom long // This is done by fetching the state, shifting it right 12, // left 25, and then right 27. This is then saved back to the state variable. // Then, we multiply it by 2685821657736338717 (add L on the end for long) // And return the resultant value. public long getRand() { // TODO: Fill this in }
// Testing section public static void main(String[] args) { // First, we create the random object with seed = "1234" myRandom newRand = new myRandom(1234); // Then we generate an integer - this should return -4875686705675355890 System.out.println(newRand.getRand()); // This should return 5609927630774915935 System.out.println(newRand.getRand()); // And this should return 7579251470305882622 System.out.println(newRand.getRand()); // Create output files for our generator and the default Java Generator if you want to compare them try { // Write a file with 1000000 longs from our Xorshift* generator Writer fileWriter = new FileWriter("myRandOutput.txt", false); for(int x = 0; x
}
stream.java
// You should import your myRandom class here
//In this class you should implement the Xorshift* PRNG function
//(See lecture notes if you're unsure how that works)
public class stream {
// This is the internal PRNG generator
myRandom rand;
// Constructor - In this function, you should create a new
// myRandom object using the input long seed, and then store it
// in the rand class variable.
public stream(long seed) {
// TODO: Complete this.
}
// In this function you should use a loop to select each character in
// the input string. You should use the charAt() function to convert
// the character to an integer. You should then XOR that integer with:
// Math.abs(((int)rand.getRand() % 10)), which will return pseudorandom
// number between 0 and 10.
// Then, append the character to the output. Once all characters have been
// XOR'd, you should return the output.
// Note: This function also serves to decrypt an encrypted message.
public String encrypt(String input) {
// TODO: Complete this.
// Math.abs(((int)this.rand.getRand() % 10));
}
// Testing section
public static void main(String[] args) {
// First, we create the stream object with seed = "1234"
stream cipher = new stream(1234);
// Pass in a phrase to the encrypt function to encrypt it
// This should print "llhon!vorng+&mru$aipf"
System.out.println(cipher.encrypt("hello world, its cosc"));
// Create a new stream object with the same seed
stream cipher_2 = new stream(1234);
// Use it to decrypt the previous message by running it back through
// This should print "hello world, its cosc"
System.out.println(cipher_2.encrypt("llhon!vorng+&mru$aipf"));
}
}
In this lab, we'll implement the Xorshift* pseudorandom number generator that we talked about in class as a Java Class, and then we'll use it implement a simple stream cipher using a XOR combiner. The myRandom Class The first thing we'll need to do is implement the myRandom class. A skeleton code file with some tests has been provided for you. The Xorshift* algorithm can be found in the Lecture 3 slides. An example is given below in C syntax. You'll want to start by filling out the constructor function, which takes a long seed, and should then store that seed into the class state variable. The state variable will be responsible for holding the internal state of the PRNG. This is analogous to the state->a variable in the example shown below. Follow along with the comments in the skeleton code file. Note that the constant 0x2545F4914F6CDD1D can be written in Java as 2685821657736338717L. The " L " at the end is required, as this number is large enough that it must be stored in a long type. Double check that the tests provided in the main() function return their expected values. Note that this also generates two files - one filled with random numbers from our myRandom class, and the other with values generated by Java's built-in Random class. It's not required for this lab, but if you try compressing these files using a compression utility such as Winzip or 7zip, you should notice that they compress by different amounts. As a general rule, the more a file can be compressed, the less entropy that exists in that file. This can be used as a quick and dirty test of PRNG quality. The stream Class Once you've completed the myRandom class, we can move onto the stream class. Here, we'll implement a basic stream cipher based on our PRNG. The first step will be to set up the constructor. The constructor takes a long seed, which it should use to initialize a new myRandom object that is stored in the rand class variable. Then, you should fill out the encrypt function. This function takes a String input and loops over every character in the input. For each character in the input string, we can use the charAt() function to get the integer equivalent to the character. Then, we'll use the XOR function to combine it with a value from our myRandom PRNG. However, our PRNG produces very large numbers (longs), and the ASCII character table is very small - we would end up with invalid characters in our output if we just XOR'd the two together. So we need to make our random numbers smaller, and positive. We do that with the following bit of code: Math.abs(((int)this.rand.getRand()%10)) This snippet is provided in the skeleton file for the stream class. It takes a random long, casts it to an integer, performs a modulus by 10 , and then takes the absolute value of it (to eliminate negative values). We then XOR this output value with our character. Once we've XOR'd the two together, we can cast the result back to a character, append it to an output string and then print it out once we've finished looping over all characters. This method isn't foolproof however, as it can potentially still result in invalid characters for certain inputs. This is mostly because we're working with strings and chars, instead of raw bits. We'll talk more about that next lab. When you're done, submit your completed myRandom.java and stream.java files on Moodle for markingStep by Step Solution
There are 3 Steps involved in it
Step: 1
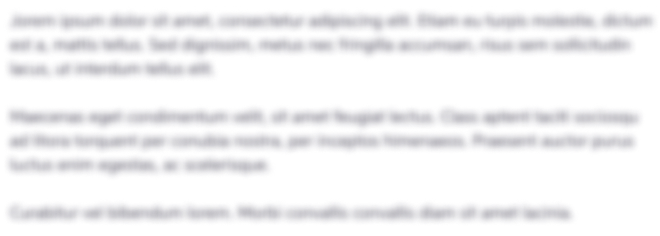
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started