Question
Name your Python script (aka 'module') lab10.py. As always, your program should have a shebang line andcommentary prologue. Be sure you haveimplemented Gem and Knapsack
Name your Python script (aka 'module') lab10.py.
As always, your program should have a shebang line andcommentary prologue.
Be sure you haveimplemented Gem and Knapsack classes.
Use docstrings to thoroughlydocument Gem and Knapsack classes, as well aseach of their methods.
In addition tocontaining Gem and Knapsack classes,the lab10.py module should also haveamain() function. The main() function should executewhenever the lab10.py script is executed from the commandline.
Pay close attention to the expected output, as shown in theSample Run.
Include a shebang line and commentary prologue, as usual.
Define methods print_heading(), get_input(),and main(). Use pass for the body of each method, asa placeholder in our top-down design.
Above the methods defined in the previous step, stub outthe Gem and Knapsack classes. For each of these2 classes, include all the methods specified inthe Object-oriented Design sectionabove. Write docstring's for all your methods, which will helpyou develop a clear understanding of how the classes are intendedto work. A good docstring typically explains what the method does,describes the input parameters (if any), and the return value (ifany).
This is what it should output
Enter Gem filename: gems.txtEnter Knapsack capacity: 5Vault inventory: panite - 1.00 carats, $1800, $1800.00/ct ruby - 2.00 carats, $ 100, $ 50.00/ct diamond - 0.75 carats, $ 900, $1200.00/ct emerald - 3.00 carats, $ 250, $ 83.33/ct amethyst - 2.00 carats, $ 50, $ 25.00/ct opal - 1.00 carats, $ 300, $ 300.00/ct sapphire - 0.50 carats, $ 750, $1500.00/ct benitoite - 1.00 carats, $2000, $2000.00/ct malachite - 1.00 carats, $ 60, $ 60.00/ctBooty in knapsack: benitoite - 1.00 carats, $2000, $2000.00/ct panite - 1.00 carats, $1800, $1800.00/ct sapphire - 0.50 carats, $ 750, $1500.00/ct diamond - 0.75 carats, $ 900, $1200.00/ct opal - 1.00 carats, $ 300, $ 300.00/ctKnapsack capacity: 5.00 ct Booty: 4.25 ct Unused: 0.75 ct Total heist: $5750.00
Implement the print_heading() method which will simplyprint the required heading for the output.
Go back to your Gem class and implement theconstructor and string, __init__ and __str__.
Implement the main() function:
Invoke the print_heading method.
Prompt for and receive user input. The name of the data filewill be a string, and the capacity of the knapsack will be afloating point number.
Initialize a variable (I called this variable vault inmy solution) as an empty list.
Open the data file, and just echo the lines back to theterminal, so that you know this much of your program is workingcorrectly.
Modify the loop in the previous step. For each line in the datafile, don't print to the screen, printing output to the console,but instead instantiate a corresponding Gem object, usingthe Gemconstructor. After you instantiateeach Gem object, add that object to the vault list.
Use the __str__ method of the Gem class toprint the vault inventory to the console, in the format shown inthe Sample Run.
Implement the valuePerCarat method inthe Gem class, then use that method as a key to sort thegems in the vault.
Implement the constructor, unusedCapacity, addGem,and __str__ methods in the Knapsackclass. Theconstructor should initialize the capacity with a floating pointvalue, as specified by the user input. The constructor should alsoinitialize an empty list, which will hold the Gem objectsthat fit in the knapsack. The __str__ method shouldgenerate a string which describes the knapsack, in the fashionshown in the Sample Run..
In the main function, after printing the gems in thevault, add code to initialize a Knapsack object with thedesired capacity.
Iterate through all the gems in the (now sorted) gem vault,selecting those gems which will fit in the knapsack.
Implement the __str__ method to produce a stringdescribing the knapsack (as in the SampleRun) , and use that method in the main functionto print the knapsack.
Step by Step Solution
3.53 Rating (156 Votes )
There are 3 Steps involved in it
Step: 1
usrbinenv python3 Lab 10 Gem and Knapsack Classes This module contains Gem and Knapsack classes as well as a main function which executes when the lab10py file is run from the command line class Gem T...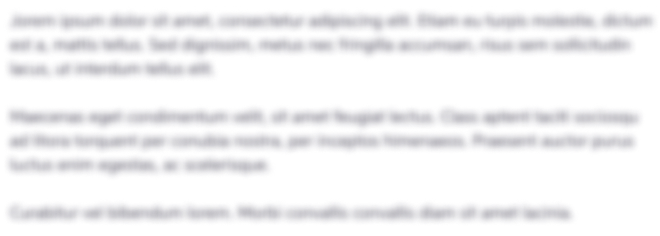
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started