Question
need a movies.h and movies.cpp program fomr a structure program converted to a class program titled moviesh and movies.cpp in covereted h file need both
need a movies.h and movies.cpp program fomr a structure program converted to a class program titled moviesh and movies.cpp in covereted h file need both public and private accesssors
here is the .h structure file
#ifndef MOVIES_H #define MOVIES_H
#include "Movie.h" #include #include #include
using namespace std;
struct Movies { Movie** moviesArray; //an array of pointers - each pointer points to a single Movie int maxMovies; //maximum number of elements in the array int numMovies; //current number of movies in the array };
/* Function name: createMovies Parameters: An integer containing the maximum size of the movie library Returns: A pointer to a new Movies structure Purpose: This function should be called when the user needs to create a library of movies. The function will dynamically allocate a movies array based on the maximum size and will also set the current number of movies to zero. */ Movies* createMovies(int);
/* Function name: addMovieToArray Parameters: 1) The movies structure (which contains the movie library) Returns: none Purpose: This function should be called when you need to add a single movie to the movie library. */ void addMovieToArray(Movies*);
/* Function name: editMovieInArray Parameters: The movies structure (which contains the movie library) Returns: none Purpose: This function should be called when you need to edit a movie in the array */ void editMovieInArray(Movies*);
/* Function name: destroyMovies Parameters: 1) The movies structure (which contains the movie library) Returns: none (void) Purpose: This function should be called when you need to remove all the single movies in the movie library as well as the movie library. This releases all the dynamically allocated space in memory. */ void destroyMovies(Movies*);
/* Function name: displayMovies Parameters: 1) The movies structure (which contains the movie library) Returns: none (void) Purpose: This function should be called when the user wants to have all the movies in the library printed to the screen. */ void displayMovies(Movies*);
/* Function name: displayMovieTitles Parameters: 1) The movies structure (which contains the movie library) Returns: none (void) Purpose: This function should be called when you want to print only the movie titles out of the movie library */ void displayMovieTitles(Movies*);
/* Function name: readMoviesFromFile Parameters: 1) A pointer to a character (c-string or string literal argument) containing the filename 2) The movies structure (which contains the movie library) Returns: none (void) Purpose: This function should be called when the user wants to read movie data from a file and add the movies to the movie library. The file must have data in the following order: title, length, year, genre, rating, num oscars won, star rating */ void readMoviesFromFile(char* filename, Movies* myMovies);
/* Function name: removeMovieFromArray Parameters: The movies structure (which contains the movie library) Returns: none (void) Purpose: This function should be called when the user wants to remove one single movie from the movie library. The function will list all the movie names and allow the user to select the movie that they wish to remove. Then this function removes the movie. */ void removeMovieFromArray(Movies* myMovies);
/* Function name: saveToFile Parameters: 1) A pointer to a character (c-string or string literal argument) containing the filename 2) The movies structure (which contains the movie library) Returns: none (void) Purpose: This function should be called when the user wants to print all the movie data from the movie library to a file. The data is printed in the following order (one piece of data per line): title, length, year, genre, rating, num oscars won, star rating */ void saveToFile(char *filename, Movies* myMovies);
/* Function name: resizeMovieArray Parameters: The movies structure (which contains the movie library) Returns: none (void) Purpose: This function is called by addMovieToArray when the array size is not big enough to hold a new movie that needs to be added. The function makes the array twice as big as it currently is and then moves all the movie pointers to this new array. */ void resizeMovieArray(Movies* myMovies);
#endif
here is the structure .cpp file
#include "Movies.h" #include "Movie.h"
Movies* createMovies(int max) { //dynamically create a new Movies structure Movies* myMovies = new Movies; myMovies->maxMovies = max; myMovies->numMovies = 0; //dynamically create the array that will hold the movies myMovies->moviesArray = new Movie*[max]; return myMovies; }
void resizeMovieArray(Movies* myMovies) { int max = myMovies->maxMovies * 2; //increase size by 2 //make an array that is twice as big as the one I've currently got Movie** newMoviesArray = new Movie*[max]; for(int x = 0; x < myMovies->numMovies; x++) { newMoviesArray[x] = myMovies->moviesArray[x]; } //delete the original array from memory delete [] myMovies->moviesArray; myMovies->moviesArray = newMoviesArray; myMovies->maxMovies = max; }
void addMovieToArray(Movies* myMovies) { char tempString[100]; int length, year, numOscars; double numStars; Text* title; Text* genre; Text* rating; //get movie data from the user cin.ignore(); //remove the from the keyboard buffer cout << " MOVIE TITLE: "; cin.getline(tempString, 100); title = createText(tempString); cout << " MOVIE LENGTH (in minutes): "; cin >> length; cout << " MOVIE YEAR: "; cin >> year; cin.ignore(); cout << " MOVIE GENRE: "; cin.getline(tempString, 100); genre = createText(tempString); cout << " MOVIE RATING: "; cin.getline(tempString, 100); rating = createText(tempString); cout << " NUMBER OF OSCARS WON: "; cin >> numOscars; cout << " STAR RATING (out of 10): "; cin >> numStars; //create the movie Movie* oneMovie = createMovie(title, length, year, genre, rating, numOscars, numStars); //add the movie to the library if(myMovies->numMovies == myMovies->maxMovies) resizeMovieArray(myMovies); //increase size by 2
myMovies->moviesArray[myMovies->numMovies] = oneMovie;
(myMovies->numMovies)++; }
void removeMovieFromArray(Movies* myMovies) { int movieChoice; cout << myMovies->numMovies << endl << endl; //delete a movie if there is more than one movie in the library. if((myMovies->numMovies) <= 1) { cout << endl << "There must always be at least one movie in your library. You can\'t"; cout << " remove any movies right now or you will have no movies in your library. "; } else { cout << " Choose from the following movies to remove: "; displayMovieTitles(myMovies); cout << " Choose a movie to remove between 1& " << myMovies->numMovies << ": "; cin >> movieChoice; while(movieChoice < 1 || movieChoice > myMovies->numMovies) { cout << " Oops! You must enter a number between 1 & " << myMovies->numMovies << ": "; cin >> movieChoice; } int movieIndexToBeRemoved = movieChoice-1; Text* movieTitle; movieTitle = myMovies->moviesArray[movieIndexToBeRemoved]->movieTitle; //destroy this movie destroyMovie(myMovies->moviesArray[movieIndexToBeRemoved]); int numElementsToMoveBack = (myMovies->numMovies) - 1; for(int x = movieIndexToBeRemoved; x < numElementsToMoveBack; x++) { myMovies->moviesArray[x] = myMovies->moviesArray[x+1]; //move array elements! } //set the last movie to a null pointer myMovies->moviesArray[numElementsToMoveBack] = NULL; //decrement the current number of movies (myMovies->numMovies)--; cout << " The movie \""; displayText(movieTitle); cout << "\" has been successfully deleted. "; } }
void editMovieInArray(Movies* myMovies) { int movieChoice; cout << " Choose from the following movies to edit: "; displayMovieTitles(myMovies); cout << " Choose a movie to remove between 1 & "<< myMovies->numMovies << ": "; cin >> movieChoice; while(movieChoice < 1 || movieChoice > myMovies->numMovies) { cout << " Oops! You must enter a number between 1 & " << myMovies->numMovies << ": "; cin >> movieChoice; } Movie* oneMovie = myMovies->moviesArray[movieChoice-1]; editMovie(oneMovie); }
void destroyMovies(Movies* myMovies) { //delete each movie for(int x=0; x< myMovies->numMovies; x++) { //delete myMovies->moviesArray[x]; destroyMovie(myMovies->moviesArray[x]); } //delete movies array delete [] myMovies->moviesArray; //delete myMovies delete myMovies; }
void displayMovies(Movies* myMovies) { if(myMovies->numMovies > 0) { for(int x=0; x < (myMovies->numMovies); x++) { cout << endl << right << setw(50) << "----------MOVIE " << (x+1) << "----------"; printMovieDetails(myMovies->moviesArray[x]); //function is in Movie.cpp } } else cout << " There are no movies in your library yet."; }
void displayMovieTitles(Movies* myMovies) { Text* movieTitle; for(int x=0; x < (myMovies->numMovies); x++) { cout << " MOVIE " << (x+1) <<": "; movieTitle = myMovies->moviesArray[x]->movieTitle; displayText(movieTitle); } }
void readMoviesFromFile(char *filename, Movies* myMovies) { int numMoviesReadFromFile = 0; ifstream inFile; char temp[100]; Text* title; Text* genre; Text* rating; Movie* oneMovie; int movieLength; //length of movie in minutes int movieYear; //year released int movieOscars; //number of oscars won float movieNumStars; //from IMDB out of 10 stars inFile.open(filename); if(inFile.good()) { inFile.getline(temp, 100); while(!inFile.eof()) { title = createText(temp);//create a text for the movie title inFile >> movieLength; inFile >> movieYear; inFile.ignore(); //get rid of in the inFile buffer inFile.getline(temp, 100); //read in genre genre = createText(temp); //create a text for genre inFile.getline(temp, 100); //read in rating rating = createText(temp); //create a text for rating inFile >> movieOscars; inFile >> movieNumStars; inFile.ignore(); //get rid of in the inFile buffer //one movie has been read from the file. Now create a movie object oneMovie = createMovie(title, movieLength, movieYear, genre, rating, movieOscars, movieNumStars); //now add this movie to the library if(myMovies->numMovies == myMovies->maxMovies) resizeMovieArray(myMovies); //increase size by 2 myMovies->moviesArray[myMovies->numMovies] = oneMovie; (myMovies->numMovies)++; //confirm addition to the user cout << endl; displayText(title); cout << " was added to the movie library! "; inFile.getline(temp, 100); //read in the next movie title if there is one numMoviesReadFromFile++; } cout << " " << numMoviesReadFromFile<< " movies were read from the file and added to your movie library. "; } else { cout << " Sorry, I was unable to open the file. "; } }
void saveToFile(char *filename, Movies* myMovies) { ofstream outFile; outFile.open(filename); for(int x=0; x < (myMovies->numMovies); x++) { printMovieDetailsToFile(myMovies->moviesArray[x], outFile); //function in Movies.cpp } outFile.close(); cout << " All movies in your library have been printed to " << filename << endl; }
2,619 answers
ANUNAGA
2,619 answers
"undefined"
Movie.h
#ifndef MOVIE_H
#define MOVIE_H
#include "Text.h"
#include
#include
#include
#include
using namespace std;
class Movie
{
private:
Text *movieTitle; //title of movie
int movieLength; //length of movie in minutes
int movieYear; //year released
Text *movieGenre; //comedy, horror, sci-fi, fantasy, romance, thriller, drama, action, biography
Text *movieRating; //G, PG, PG-13, R, MA
int movieOscars; //number of oscars won
float movieNumStars; //taken from IMDB on 10 star scale
public:
/*
Function name: createMovie (overloaded function)
Parameters: 1) A pointer to a Text variable, containing a c-string and the length of the string.
2) An integer containing the length of the movie
Returns: A pointer to a new Movie structure
Purpose: This function should be called when only the title of the movie and the length of
the movie is known and it will create a new movie with this information.
*/
Movie *createMovie(Text *, int);
/*
Function name: createMovie (overloaded function)
Parameters: 1) A pointer to a Text variable, containing the title of the movie
2) An integer containing the length of the movie
3) An integer containing the year the movie was released
4) A pointer to a Text variable, containing the genre of the movie
5) A pointer to a Text variable, containing the rating of the movie
6) An integer containing the number of oscars the movie won
7) A float containing the IMDB rating of the movie (out of 10 stars)
Returns: A pointer to a new Movie structure
Purpose: This function should be called when all movie information is known and
it will create a new movie with this information.
*/
Movie *createMovie(Text *, int, int, Text *, Text *, int, float);
/*
Function name: editMovie
Parameters: A pointer to a movie structure
Returns: nothing (void)
Purpose: This function should be called when the user wants to edit a single
movie's data
*/
void editMovie();
/*
Function name: destroyMovie
Parameters: A pointer to a movie structure
Returns: nothing (void)
Purpose: This function should be called when there is no longer need for the
movie in the database (like when removing or deleting a movie).
*/
void destroyMovie();
/*
Function name: printMovieDetails
Parameters: A pointer to a movie structure
Returns: nothing (void)
Purpose: This function should be called when the user wants to print ALL
the movie information to the screen.
*/
void printMovieDetails();
/*
Function name: printMovieDetailsToFile
Parameters: A pointer to a movie structure, a file stream object (sent by reference)
Returns: nothing (void)
Purpose: This function should be called when the user wants to print ALL
the movie information to the file.
*/
void printMovieDetailsToFile(ofstream &outFile);
};
#endif
Movie.cpp
#include "Movie.h"
#include "Text.h"
Movie *Movie::createMovie(Text *title, int length)
{
//dynamically allocate a new Movie
Movie *myMovie = new Movie;
//assign parameter data to structure memebers
myMovie->movieTitle = title;
myMovie->movieLength = length;
return myMovie;
}
Movie *Movie::createMovie(Text *title, int length, int year, Text *genre, Text *rating, int nom, float stars) //all info is know
{
//dynamically allocate a new Movie
Movie *myMovie = new Movie;
//assign parameter data to structure members
myMovie->movieTitle = title;
myMovie->movieLength = length;
myMovie->movieYear = year;
myMovie->movieGenre = genre;
myMovie->movieRating = rating;
myMovie->movieOscars = nom;
myMovie->movieNumStars = stars;
return myMovie;
}
void Movie::destroyMovie()
{
destroyText(movieTitle);
destroyText(movieGenre);
destroyText(movieRating);
delete myMovie;
}
void Movie::printMovieDetails()
{
cout << endl;
cout << right << setw(30) << "Movie Title: "<< left;
displayText(movieTitle);
cout << endl;
cout << right << setw(30) << "Length (minutes): " << left << movieLength << endl;
cout << right << setw(30) << "Year Released: "<< left << movieYear << endl;
cout << right << setw(30) << "Genre: "<< left;
displayText(movieGenre);
cout << endl;
cout << right << setw(30) << "Rating: "<< left;
displayText(movieRating);
cout << endl;
cout << right << setw(30) << "Number of Oscars Won: " << left << movieOscars << endl;
cout << right << setw(30) << "Number of Stars: " << left << movieNumStars << endl
<< endl;
}
void Movie::printMovieDetailsToFile(ofstream &outFile)
{
char temp[1000];
strncpy(temp, getText(movieTitle), 1000);
outFile << temp << endl;
outFile << movieLength << endl;
outFile << movieYear << endl;
strncpy(temp, getText(movieGenre), 1000);
outFile << temp << endl;
strncpy(temp, getText(movieRating), 1000);
outFile << temp << endl;
outFile << movieOscars << endl;
outFile << movieNumStars << endl;
}
void Movie::editMovie()
{
int choice;
Text *tempText;
char temp[100];
do
{
cout << " Which detail do you wish to edit? ";
cout << "1. Title ";
cout << "2. Length ";
cout << "3. Year ";
cout << "4. Genre ";
cout << "5. Rating ";
cout << "6. Number of Oscars Won ";
cout << "7. Number of Stars ";
cout << "8. DONE EDITING ";
cout << "CHOOSE 1-8: ";
cin >> choice;
while (choice < 1 || choice > 8)
{
cout << " OOPS! Enter choice 1 through 8: ";
cin >> choice;
}
cin.ignore();
switch (choice)
{
case 1:
cout << " Current Title: ";
displayText(movieTitle);
destroyText(movieTitle);
cout << " NEW TITLE: ";
cin.getline(temp, 100);
tempText = createText(temp);
movieTitle = tempText;
break;
case 2:
cout << " Current Length: " << movieLength;
cout << " NEW LENGTH: ";
cin >> movieLength;
break;
case 3:
cout << " Current Year: " << movieYear;
cout << " NEW LENGTH: ";
cin >> movieYear;
break;
case 4:
cout << " Current Genre: ";
displayText(movieGenre);
destroyText(movieGenre);
cout << " NEW GENRE: ";
cin.getline(temp, 100);
tempText = createText(temp);
movieGenre = tempText;
break;
case 5:
cout << " Current Rating: ";
displayText(movieRating);
destroyText(movieRating);
cout << " NEW GENRE: ";
cin.getline(temp, 100);
tempText = createText(temp);
movieRating = tempText;
break;
case 6:
cout << " Current Number of Oscars Won: " << movieOscars;
cout << " NEW NUMBER OF OSCARS: ";
cin >> movieOscars;
break;
case 7:
cout << " Current Star Rating from IMDB: " << movieNumStars;
cout << " NEW STAR RATING: ";
cin >> movieNumStars;
break;
}
} while (choice != 8);
}
Text *Movie::getMovieTitle()
{
return movieTitle;
}
int Movie::getMovieLength()
{
return movieLength;
}
int Movie::getMovieYear()
{
return movieYear;
}
Text *Movie::getMovieGenre()
{
return movieGenre;
}
Text *Movie::getMovieRating()
{
return movieRating;
}
int Movie::getMovieOscars()
{
return movieOscars;
}
float Movie::getMovieNumStars()
{
return movieNumStars;
}
void Movie::setMovieTitle(Text *title)
{
movieTitle = title;
}
void Movie::setMovieLength(int length)
{
movieLength = length;
}
void Movie::setMovieYear(int year)
{
movieYear = year;
}
void Movie::setMovieGenre(Text *genre)
{
movieGenre = genre;
}
void Movie::setMovieRating(Text *rating)
{
movieRating = rating;
}
void Movie::setMovieOscars(int oscars)
{
movieOscars = oscars;
}
void Movie::setMovieNumStars(float stars)
{
movieNumStars = stars;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
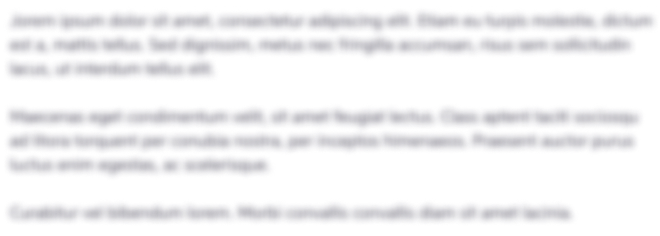
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started